mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-22 03:30:37 +00:00
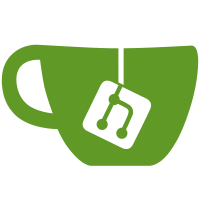
Added CL tests to have test coverage over 60% in all files covered by running ssh ./cl/coverage
42 lines
903 B
Go
42 lines
903 B
Go
package merkle_tree
|
|
|
|
func NextPowerOfTwo(n uint64) uint64 {
|
|
if n == 0 {
|
|
return 1
|
|
}
|
|
// http://graphics.stanford.edu/~seander/bithacks.html#RoundUpPowerOf2
|
|
n--
|
|
n |= n >> 1
|
|
n |= n >> 2
|
|
n |= n >> 4
|
|
n |= n >> 8
|
|
n |= n >> 16
|
|
n |= n >> 32
|
|
n++
|
|
|
|
return n
|
|
}
|
|
|
|
// GetDepth returns the depth of a merkle tree with a given number of nodes.
|
|
// The depth is defined as the number of levels in the tree, with the root
|
|
// node at level 0 and each child node at a level one greater than its parent.
|
|
// If the number of nodes is less than or equal to 1, the depth is 0.
|
|
func GetDepth(v uint64) uint8 {
|
|
// If there are 0 or 1 nodes, the depth is 0.
|
|
if v <= 1 {
|
|
return 0
|
|
}
|
|
|
|
// Initialize the depth to 0.
|
|
depth := uint8(0)
|
|
|
|
// Divide the number of nodes by 2 until it is less than or equal to 1.
|
|
// The number of iterations is the depth of the tree.
|
|
for v > 1 {
|
|
v >>= 1
|
|
depth++
|
|
}
|
|
|
|
return depth
|
|
}
|