mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-22 03:30:37 +00:00
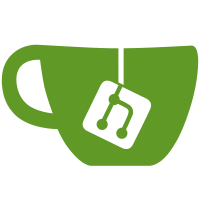
* save state * snapshot update works * save state * snapshot migrator * tx test * save state * migrations stages refactor * refactor snapshot migrator * compilation fixed * integrate snapshot migrator * goerli sync headers * debug async snapshotter on goerly * move verify headers, remove experiments, fix remove old snapshot * save state * refactor snapshotsync injection * fix deadlock * replace snapshot generation stage logic to migrate method * change done for body snapshot * clean * clean&&change deleted value * clean * fix hash len * fix hash len * remove one of wrap methods, add remove snapshots on start * add err check * fix shadowing * stages unwind order debug * matryoshka experiments * steam test * fix build * fix test * fix lint * fix test * fix test datarace * add get test * return timeout * fix mdbx overlap * fix after merge * change epoch size * clean todo * fix * return testdata * added return from sndownloader gorutine * fix review comments * Fix * More info Co-authored-by: Alex Sharp <alexsharp@Alexs-MacBook-Pro.local>
44 lines
1.5 KiB
Go
44 lines
1.5 KiB
Go
// Copyright 2014 The go-ethereum Authors
|
|
// This file is part of the go-ethereum library.
|
|
//
|
|
// The go-ethereum library is free software: you can redistribute it and/or modify
|
|
// it under the terms of the GNU Lesser General Public License as published by
|
|
// the Free Software Foundation, either version 3 of the License, or
|
|
// (at your option) any later version.
|
|
//
|
|
// The go-ethereum library is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
// GNU Lesser General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU Lesser General Public License
|
|
// along with the go-ethereum library. If not, see <http://www.gnu.org/licenses/>.
|
|
|
|
package vm
|
|
|
|
// codeBitmap collects data locations in code.
|
|
func codeBitmap(code []byte) []uint64 {
|
|
// The bitmap is 4 bytes longer than necessary, in case the code
|
|
// ends with a PUSH32, the algorithm will push zeroes onto the
|
|
// bitvector outside the bounds of the actual code.
|
|
bits := make([]uint64, (len(code)+32+63)/64)
|
|
|
|
for pc := 0; pc < len(code); {
|
|
op := OpCode(code[pc])
|
|
pc++
|
|
if op >= PUSH1 && op <= PUSH32 {
|
|
numbits := int(op - PUSH1 + 1)
|
|
x := uint64(1) << (op - PUSH1)
|
|
x = x | (x - 1) // Smear the bit to the right
|
|
idx := pc / 64
|
|
shift := pc & 63
|
|
bits[idx] |= x << shift
|
|
if shift+shift > 64 {
|
|
bits[idx+1] |= x >> (64 - shift)
|
|
}
|
|
pc += numbits
|
|
}
|
|
}
|
|
return bits
|
|
}
|