mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-22 03:30:37 +00:00
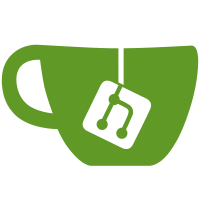
* move mocks to the owner packages * squash single file packages * move types to more appropriate files * remove unused mocks
59 lines
1.3 KiB
Go
59 lines
1.3 KiB
Go
package heimdall
|
|
|
|
import (
|
|
"math/big"
|
|
|
|
libcommon "github.com/ledgerwatch/erigon-lib/common"
|
|
)
|
|
|
|
// milestone defines a response object type of bor milestone
|
|
type Milestone struct {
|
|
Proposer libcommon.Address `json:"proposer"`
|
|
StartBlock *big.Int `json:"start_block"`
|
|
EndBlock *big.Int `json:"end_block"`
|
|
Hash libcommon.Hash `json:"hash"`
|
|
BorChainID string `json:"bor_chain_id"`
|
|
Timestamp uint64 `json:"timestamp"`
|
|
}
|
|
|
|
type MilestoneResponse struct {
|
|
Height string `json:"height"`
|
|
Result Milestone `json:"result"`
|
|
}
|
|
|
|
type MilestoneCount struct {
|
|
Count int64 `json:"count"`
|
|
}
|
|
|
|
type MilestoneCountResponse struct {
|
|
Height string `json:"height"`
|
|
Result MilestoneCount `json:"result"`
|
|
}
|
|
|
|
type MilestoneLastNoAck struct {
|
|
Result string `json:"result"`
|
|
}
|
|
|
|
type MilestoneLastNoAckResponse struct {
|
|
Height string `json:"height"`
|
|
Result MilestoneLastNoAck `json:"result"`
|
|
}
|
|
|
|
type MilestoneNoAck struct {
|
|
Result bool `json:"result"`
|
|
}
|
|
|
|
type MilestoneNoAckResponse struct {
|
|
Height string `json:"height"`
|
|
Result MilestoneNoAck `json:"result"`
|
|
}
|
|
|
|
type MilestoneID struct {
|
|
Result bool `json:"result"`
|
|
}
|
|
|
|
type MilestoneIDResponse struct {
|
|
Height string `json:"height"`
|
|
Result MilestoneID `json:"result"`
|
|
}
|