mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-22 03:30:37 +00:00
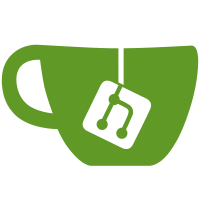
It is possible that a span update happens during a milestone. A headers slice might cross to the new span. Also if 2 forks evolve simulaneously, a shorter fork can still be in the previous span. In these cases we need access to the previous span to calculate difficulty and validate header times. SpansCache will keep recent spans. The cache will be updated on new span events from the heimdall. The cache is pruned on new milestone events and in practice no more than 2 spans are kept. The header difficulty calculation and time validation depends on having a span for that header in the cache.
78 lines
2.2 KiB
Go
78 lines
2.2 KiB
Go
package sync
|
|
|
|
import (
|
|
"fmt"
|
|
"time"
|
|
|
|
lru "github.com/hashicorp/golang-lru/arc/v2"
|
|
|
|
libcommon "github.com/ledgerwatch/erigon-lib/common"
|
|
"github.com/ledgerwatch/erigon/core/types"
|
|
"github.com/ledgerwatch/erigon/eth/stagedsync"
|
|
"github.com/ledgerwatch/erigon/polygon/bor"
|
|
"github.com/ledgerwatch/erigon/polygon/bor/borcfg"
|
|
"github.com/ledgerwatch/erigon/polygon/bor/valset"
|
|
)
|
|
|
|
type HeaderTimeValidator interface {
|
|
ValidateHeaderTime(header *types.Header, now time.Time, parent *types.Header) error
|
|
}
|
|
|
|
type headerTimeValidatorImpl struct {
|
|
borConfig *borcfg.BorConfig
|
|
spans *SpansCache
|
|
validatorSetFactory func(headerNum uint64) validatorSetInterface
|
|
signaturesCache *lru.ARCCache[libcommon.Hash, libcommon.Address]
|
|
}
|
|
|
|
func NewHeaderTimeValidator(
|
|
borConfig *borcfg.BorConfig,
|
|
spans *SpansCache,
|
|
validatorSetFactory func(headerNum uint64) validatorSetInterface,
|
|
signaturesCache *lru.ARCCache[libcommon.Hash, libcommon.Address],
|
|
) HeaderTimeValidator {
|
|
if signaturesCache == nil {
|
|
var err error
|
|
signaturesCache, err = lru.NewARC[libcommon.Hash, libcommon.Address](stagedsync.InMemorySignatures)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
}
|
|
|
|
impl := headerTimeValidatorImpl{
|
|
borConfig: borConfig,
|
|
spans: spans,
|
|
validatorSetFactory: validatorSetFactory,
|
|
signaturesCache: signaturesCache,
|
|
}
|
|
|
|
if validatorSetFactory == nil {
|
|
impl.validatorSetFactory = impl.makeValidatorSet
|
|
}
|
|
|
|
return &impl
|
|
}
|
|
|
|
func (impl *headerTimeValidatorImpl) makeValidatorSet(headerNum uint64) validatorSetInterface {
|
|
span := impl.spans.SpanAt(headerNum)
|
|
if span == nil {
|
|
return nil
|
|
}
|
|
return valset.NewValidatorSet(span.ValidatorSet.Validators)
|
|
}
|
|
|
|
func (impl *headerTimeValidatorImpl) ValidateHeaderTime(header *types.Header, now time.Time, parent *types.Header) error {
|
|
headerNum := header.Number.Uint64()
|
|
validatorSet := impl.validatorSetFactory(headerNum)
|
|
if validatorSet == nil {
|
|
return fmt.Errorf("headerTimeValidatorImpl.ValidateHeaderTime: no span at %d", headerNum)
|
|
}
|
|
|
|
sprintNum := impl.borConfig.CalculateSprintNumber(headerNum)
|
|
if sprintNum > 0 {
|
|
validatorSet.IncrementProposerPriority(int(sprintNum))
|
|
}
|
|
|
|
return bor.ValidateHeaderTime(header, now, parent, validatorSet, impl.borConfig, impl.signaturesCache)
|
|
}
|