mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-22 03:30:37 +00:00
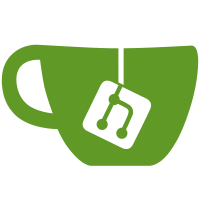
Changed distribution of httpcfg.HttpCfg to be pointer. Added new flags: rpc.slow.log - which is false by default, this flag need to enable logging slow RPC requests rpc.slow.log.threshold - which is 100 by default, this flag specify slow threshold in milliseconds Updated rpc handler to log slow requests: - added map[request id] {method, timestamp} - put every request details to map above - delete request details from map above - added time interval check for elements in map and if time difference is more than given threshold print request id and the method - app will print slow requests in next cases: 1. As soon as request take more than given threshold 2. Every 20 seconds if request still in process 3. After request finished and it took more than give threshold --------- Co-authored-by: alex.sharov <AskAlexSharov@gmail.com>
46 lines
1.5 KiB
Go
46 lines
1.5 KiB
Go
package rpccfg
|
|
|
|
import (
|
|
"time"
|
|
)
|
|
|
|
// HTTPTimeouts represents the configuration params for the HTTP RPC server.
|
|
type HTTPTimeouts struct {
|
|
// ReadTimeout is the maximum duration for reading the entire
|
|
// request, including the body.
|
|
//
|
|
// Because ReadTimeout does not let Handlers make per-request
|
|
// decisions on each request body's acceptable deadline or
|
|
// upload rate, most users will prefer to use
|
|
// ReadHeaderTimeout. It is valid to use them both.
|
|
ReadTimeout time.Duration
|
|
|
|
// WriteTimeout is the maximum duration before timing out
|
|
// writes of the response. It is reset whenever a new
|
|
// request's header is read. Like ReadTimeout, it does not
|
|
// let Handlers make decisions on a per-request basis.
|
|
WriteTimeout time.Duration
|
|
|
|
// IdleTimeout is the maximum amount of time to wait for the
|
|
// next request when keep-alives are enabled. If IdleTimeout
|
|
// is zero, the value of ReadTimeout is used. If both are
|
|
// zero, ReadHeaderTimeout is used.
|
|
IdleTimeout time.Duration
|
|
}
|
|
|
|
// DefaultHTTPTimeouts represents the default timeout values used if further
|
|
// configuration is not provided.
|
|
var DefaultHTTPTimeouts = HTTPTimeouts{
|
|
ReadTimeout: 30 * time.Second,
|
|
WriteTimeout: 30 * time.Minute,
|
|
IdleTimeout: 120 * time.Second,
|
|
}
|
|
|
|
const DefaultEvmCallTimeout = 5 * time.Minute
|
|
|
|
var SlowLogBlackList = []string{
|
|
"eth_getBlock", "eth_getBlockByNumber", "eth_getBlockByHash", "eth_blockNumber",
|
|
"erigon_blockNumber", "erigon_getHeaderByNumber", "erigon_getHeaderByHash", "erigon_getBlockByTimestamp",
|
|
"eth_call",
|
|
}
|