mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-22 03:30:37 +00:00
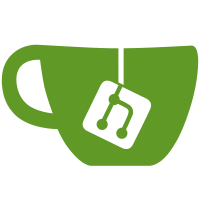
Small fix to the script for the scenario where more than one matching library can be returned. For example, the command `/sbin/ldconfig -p | grep libstdc++ | awk '{ print $NF }'` can result in ``` /lib/x86_64-linux-gnu/libstdc++.so.6 /lib32/libstdc++.so.6 ``` which then fails the check `if [[ ! -L "$link_path" ]]`
88 lines
1.8 KiB
Bash
Executable File
88 lines
1.8 KiB
Bash
Executable File
#!/bin/bash
|
|
|
|
set -e
|
|
set -u
|
|
set -o pipefail
|
|
|
|
OS_RELEASE_PATH=/etc/os-release
|
|
|
|
function glibc_version {
|
|
cmd="ldd --version"
|
|
$cmd | head -1 | awk '{ print $NF }'
|
|
}
|
|
|
|
function glibcpp_version {
|
|
link_path=$(/sbin/ldconfig -p | grep libstdc++ | awk '{ print $NF }' | head -1)
|
|
if [[ ! -L "$link_path" ]]
|
|
then
|
|
echo "0"
|
|
else
|
|
file_name=$(readlink "$link_path")
|
|
echo "${file_name##*.}"
|
|
fi
|
|
}
|
|
|
|
function version_major {
|
|
IFS='.' read -a components <<< "$1"
|
|
echo "${components[0]}"
|
|
}
|
|
|
|
function version_minor {
|
|
IFS='.' read -a components <<< "$1"
|
|
echo "${components[1]}"
|
|
}
|
|
|
|
case $(uname -s) in
|
|
Linux)
|
|
if [[ ! -f "$OS_RELEASE_PATH" ]]
|
|
then
|
|
echo "not supported Linux without $OS_RELEASE_PATH"
|
|
exit 2
|
|
fi
|
|
|
|
source "$OS_RELEASE_PATH"
|
|
|
|
if [[ -n "$ID" ]] && [[ -n "$VERSION_ID" ]]
|
|
then
|
|
version=$(version_major "$VERSION_ID")
|
|
case "$ID" in
|
|
"debian")
|
|
if (( version < 12 ))
|
|
then
|
|
echo "not supported Linux version: $ID $VERSION_ID"
|
|
exit 3
|
|
fi
|
|
;;
|
|
"ubuntu")
|
|
if (( version < 22 ))
|
|
then
|
|
echo "not supported Linux version: $ID $VERSION_ID"
|
|
exit 3
|
|
fi
|
|
;;
|
|
esac
|
|
fi
|
|
|
|
version=$(version_minor "$(glibc_version)")
|
|
if (( version < 34 ))
|
|
then
|
|
echo "not supported glibc version: $version"
|
|
exit 4
|
|
fi
|
|
|
|
version=$(glibcpp_version)
|
|
if (( version < 30 ))
|
|
then
|
|
echo "not supported glibcpp version: $version"
|
|
exit 5
|
|
fi
|
|
|
|
;;
|
|
Darwin)
|
|
;;
|
|
*)
|
|
echo "unsupported OS"
|
|
exit 1
|
|
;;
|
|
esac
|