mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-22 11:41:19 +00:00
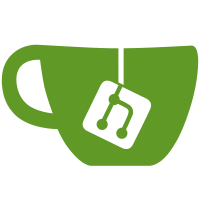
The fixes here fix a couple of issues related to devnet start-up 1. macos threading and syscall error return where causing multi node start to both not wait and fail 2. On windows creating DB's with the default 2 TB mapsize causes the os to reserve about 4GB of committed memory per DB. This may not be used - but is reserved by the OS - so a default bor node reserves around 10GB of storage. Starting many nodes causes the OS page file to become exhausted. To fix this the consensus DB's now use the node's OpenDatabase function rather than their own, which means that the consensus DB's take notice of the config.MdbxDBSizeLimit. This fix leaves one 4GB committed memory allocation in the TX pool which needs its own MapSize setting. --------- Co-authored-by: Alex Sharp <akhounov@gmail.com>
76 lines
2.1 KiB
Go
76 lines
2.1 KiB
Go
package app
|
|
|
|
import (
|
|
"encoding/json"
|
|
"os"
|
|
|
|
"github.com/ledgerwatch/erigon/core/types"
|
|
"github.com/ledgerwatch/erigon/turbo/debug"
|
|
"github.com/ledgerwatch/log/v3"
|
|
"github.com/urfave/cli/v2"
|
|
|
|
"github.com/ledgerwatch/erigon-lib/kv"
|
|
"github.com/ledgerwatch/erigon/cmd/utils"
|
|
"github.com/ledgerwatch/erigon/core"
|
|
"github.com/ledgerwatch/erigon/node"
|
|
)
|
|
|
|
var initCommand = cli.Command{
|
|
Action: MigrateFlags(initGenesis),
|
|
Name: "init",
|
|
Usage: "Bootstrap and initialize a new genesis block",
|
|
ArgsUsage: "<genesisPath>",
|
|
Flags: []cli.Flag{
|
|
&utils.DataDirFlag,
|
|
},
|
|
//Category: "BLOCKCHAIN COMMANDS",
|
|
Description: `
|
|
The init command initializes a new genesis block and definition for the network.
|
|
This is a destructive action and changes the network in which you will be
|
|
participating.
|
|
|
|
It expects the genesis file as argument.`,
|
|
}
|
|
|
|
// initGenesis will initialise the given JSON format genesis file and writes it as
|
|
// the zero'd block (i.e. genesis) or will fail hard if it can't succeed.
|
|
func initGenesis(ctx *cli.Context) error {
|
|
var logger log.Logger
|
|
var err error
|
|
if logger, err = debug.Setup(ctx, true /* rootLogger */); err != nil {
|
|
return err
|
|
}
|
|
// Make sure we have a valid genesis JSON
|
|
genesisPath := ctx.Args().First()
|
|
if len(genesisPath) == 0 {
|
|
utils.Fatalf("Must supply path to genesis JSON file")
|
|
}
|
|
|
|
file, err := os.Open(genesisPath)
|
|
if err != nil {
|
|
utils.Fatalf("Failed to read genesis file: %v", err)
|
|
}
|
|
defer file.Close()
|
|
|
|
genesis := new(types.Genesis)
|
|
if err := json.NewDecoder(file).Decode(genesis); err != nil {
|
|
utils.Fatalf("invalid genesis file: %v", err)
|
|
}
|
|
|
|
// Open and initialise both full and light databases
|
|
stack := MakeConfigNodeDefault(ctx, logger)
|
|
defer stack.Close()
|
|
|
|
chaindb, err := node.OpenDatabase(stack.Config(), kv.ChainDB, "", false, logger)
|
|
if err != nil {
|
|
utils.Fatalf("Failed to open database: %v", err)
|
|
}
|
|
_, hash, err := core.CommitGenesisBlock(chaindb, genesis, "", logger)
|
|
if err != nil {
|
|
utils.Fatalf("Failed to write genesis block: %v", err)
|
|
}
|
|
chaindb.Close()
|
|
logger.Info("Successfully wrote genesis state", "hash", hash.Hash())
|
|
return nil
|
|
}
|