mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-25 21:17:16 +00:00
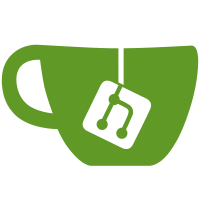
* share config object * create default config and logger * move db connection to common func * move server start to cli package * clear * clear * rename cli to rpc * use unified SetupLogger func * make all root flag persistent * use common flags in different packages * use common flags in different packages * move TraceTx method to eth package * use native slice flags * create package "turbo" * disable geth api * disable geth api * move more data types to turbo/adapter package * add support for customApiList * run more * run more * run more * dog-food * move DoCall * move DoCall * fix tests * fix test
31 lines
1.1 KiB
Go
31 lines
1.1 KiB
Go
package commands
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"github.com/ledgerwatch/turbo-geth/common"
|
|
"github.com/ledgerwatch/turbo-geth/core/rawdb"
|
|
"github.com/ledgerwatch/turbo-geth/eth"
|
|
"github.com/ledgerwatch/turbo-geth/params"
|
|
"github.com/ledgerwatch/turbo-geth/turbo/adapter"
|
|
"github.com/ledgerwatch/turbo-geth/turbo/transactions"
|
|
)
|
|
|
|
// TraceTransaction returns the structured logs created during the execution of EVM
|
|
// and returns them as a JSON object.
|
|
func (api *PrivateDebugAPIImpl) TraceTransaction(ctx context.Context, hash common.Hash, config *eth.TraceConfig) (interface{}, error) {
|
|
// Retrieve the transaction and assemble its EVM context
|
|
tx, blockHash, _, txIndex := rawdb.ReadTransaction(api.dbReader, hash)
|
|
if tx == nil {
|
|
return nil, fmt.Errorf("transaction %#x not found", hash)
|
|
}
|
|
getter := adapter.NewBlockGetter(api.dbReader)
|
|
chainContext := adapter.NewChainContext(api.dbReader)
|
|
msg, vmctx, ibs, _, err := transactions.ComputeTxEnv(ctx, getter, params.MainnetChainConfig, chainContext, api.db, blockHash, txIndex)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
// Trace the transaction and return
|
|
return transactions.TraceTx(ctx, msg, vmctx, ibs, config)
|
|
}
|