mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-25 21:17:16 +00:00
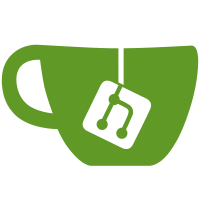
* First algorithm * Update doc * Second algorithm implemented * Prepend (unfinished) * More on prepend * More fixes * Change CalcDifficulty interface * fix make test * Fix formatting * Another test * More on Preprend * Another test for Prepend * More tests for Prepend * More tests for Prepend * Move files to turbo * Add another test for Prepend * Started adding Append algorithm * More work on Append * Start changing the terminology * Split trees into segments * More fixes * Fix compilation * Connect * FindAnchors, FindTip * Tip has anchor and not anchorParent field * Remove Prepend and Append * Add Connect * NewAnchor algorithm * More to recovery from files * Add more to AddAnchor * Request more headers * Header fix-sizes serialisation * Upgrade CI to 1.15 * Fix lint * Fix lint * replace RLP with fixed-sized serialisation * Fix lint * More algos * Fix lint
79 lines
2.6 KiB
Go
79 lines
2.6 KiB
Go
package adapter
|
|
|
|
import (
|
|
"math/big"
|
|
|
|
"github.com/ledgerwatch/turbo-geth/common"
|
|
"github.com/ledgerwatch/turbo-geth/consensus"
|
|
"github.com/ledgerwatch/turbo-geth/core/rawdb"
|
|
"github.com/ledgerwatch/turbo-geth/core/state"
|
|
"github.com/ledgerwatch/turbo-geth/core/types"
|
|
"github.com/ledgerwatch/turbo-geth/params"
|
|
"github.com/ledgerwatch/turbo-geth/rpc"
|
|
)
|
|
|
|
type chainContext struct {
|
|
db rawdb.DatabaseReader
|
|
}
|
|
|
|
func NewChainContext(db rawdb.DatabaseReader) *chainContext {
|
|
return &chainContext{
|
|
db: db,
|
|
}
|
|
}
|
|
|
|
type powEngine struct {
|
|
}
|
|
|
|
func (c *powEngine) VerifyHeader(chain consensus.ChainHeaderReader, header *types.Header, seal bool) error {
|
|
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) VerifyHeaders(chain consensus.ChainHeaderReader, headers []*types.Header, seals []bool) (func(), <-chan error) {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) VerifyUncles(chain consensus.ChainReader, block *types.Block) error {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) VerifySeal(chain consensus.ChainHeaderReader, header *types.Header) error {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) Prepare(chain consensus.ChainHeaderReader, header *types.Header) error {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) Finalize(chainConfig *params.ChainConfig, header *types.Header, state *state.IntraBlockState, txs []*types.Transaction, uncles []*types.Header) {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) FinalizeAndAssemble(chainConfig *params.ChainConfig, header *types.Header, state *state.IntraBlockState, txs []*types.Transaction,
|
|
uncles []*types.Header, receipts []*types.Receipt) (*types.Block, error) {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) Seal(_ consensus.Cancel, chain consensus.ChainHeaderReader, block *types.Block, results chan<- consensus.ResultWithContext, stop <-chan struct{}) error {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) SealHash(header *types.Header) common.Hash {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) CalcDifficulty(chain consensus.ChainHeaderReader, time, parentTime uint64, parentDifficulty, parentNumber *big.Int, parentHash, parentUncleHash common.Hash) *big.Int {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) APIs(chain consensus.ChainHeaderReader) []rpc.API {
|
|
panic("must not be called")
|
|
}
|
|
|
|
func (c *powEngine) Close() error {
|
|
panic("must not be called")
|
|
}
|
|
|
|
func (c *powEngine) Author(header *types.Header) (common.Address, error) {
|
|
return header.Coinbase, nil
|
|
}
|
|
|
|
func (c *chainContext) GetHeader(hash common.Hash, number uint64) *types.Header {
|
|
return rawdb.ReadHeader(c.db, hash, number)
|
|
}
|
|
|
|
func (c *chainContext) Engine() consensus.Engine {
|
|
return &powEngine{}
|
|
}
|