mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-22 11:41:19 +00:00
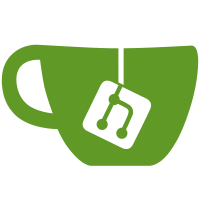
rlp2 is a package that aims to replace the existing erigon-lib/rlp package and the erigon/common/rlp it is called rlp2 for now because it requires breaking changes to erigon-lib/rlp and i do not have the time right now to test all current uses of such functions however, the encoder/decoder characteristics of rlp2 might be desirable for caplin, and also for execution layer parsing blob txns, so im putting it in a folder called rlp2 (note that it exports package rlp for easier switching later) importantly, rlp2 is designed for single-pass decoding with the ability to skip elements one does not care about. it also is zero alloc.
67 lines
1.5 KiB
Go
67 lines
1.5 KiB
Go
package rlp_test
|
|
|
|
import (
|
|
"testing"
|
|
|
|
rlp "github.com/ledgerwatch/erigon-lib/rlp2"
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
type plusOne int
|
|
|
|
func (p *plusOne) UnmarshalRLP(data []byte) error {
|
|
var s int
|
|
err := rlp.Unmarshal(data, &s)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
(*p) = plusOne(s + 1)
|
|
return nil
|
|
}
|
|
|
|
func TestDecoder(t *testing.T) {
|
|
|
|
type simple struct {
|
|
Key string
|
|
Value string
|
|
}
|
|
|
|
t.Run("ShortString", func(t *testing.T) {
|
|
t.Run("ToString", func(t *testing.T) {
|
|
bts := []byte{0x83, 'd', 'o', 'g'}
|
|
var s string
|
|
err := rlp.Unmarshal(bts, &s)
|
|
require.NoError(t, err)
|
|
require.EqualValues(t, "dog", s)
|
|
})
|
|
t.Run("ToBytes", func(t *testing.T) {
|
|
bts := []byte{0x83, 'd', 'o', 'g'}
|
|
var s []byte
|
|
err := rlp.Unmarshal(bts, &s)
|
|
require.NoError(t, err)
|
|
require.EqualValues(t, []byte("dog"), s)
|
|
})
|
|
t.Run("ToInt", func(t *testing.T) {
|
|
bts := []byte{0x82, 0x04, 0x00}
|
|
var s int
|
|
err := rlp.Unmarshal(bts, &s)
|
|
require.NoError(t, err)
|
|
require.EqualValues(t, 1024, s)
|
|
})
|
|
t.Run("ToIntUnmarshaler", func(t *testing.T) {
|
|
bts := []byte{0x82, 0x04, 0x00}
|
|
var s plusOne
|
|
err := rlp.Unmarshal(bts, &s)
|
|
require.NoError(t, err)
|
|
require.EqualValues(t, plusOne(1025), s)
|
|
})
|
|
t.Run("ToSimpleStruct", func(t *testing.T) {
|
|
bts := []byte{0xc8, 0x83, 'c', 'a', 't', 0x83, 'd', 'o', 'g'}
|
|
var s simple
|
|
err := rlp.Unmarshal(bts, &s)
|
|
require.NoError(t, err)
|
|
require.EqualValues(t, simple{Key: "cat", Value: "dog"}, s)
|
|
})
|
|
})
|
|
}
|