mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-25 21:17:16 +00:00
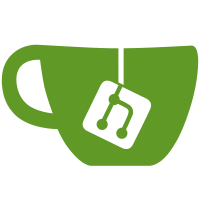
* Add kv.tx.bucket.Clear() and db.ClearBuckets() methods * Add kv.tx.bucket.Clear() and db.ClearBuckets() methods * choose db based on file suffix * implement db.id method * implement db.id method * use ethdb.NewDatabase method * use ethb.MustOpen method * cleanup * support TEST_DB env flag * create db path automatically needed * bolt - don't change prefix on happy path
69 lines
1.5 KiB
Go
69 lines
1.5 KiB
Go
package verify
|
|
|
|
import (
|
|
"bytes"
|
|
"fmt"
|
|
"math/big"
|
|
"os"
|
|
"os/signal"
|
|
"time"
|
|
|
|
"github.com/ledgerwatch/turbo-geth/common"
|
|
"github.com/ledgerwatch/turbo-geth/common/dbutils"
|
|
"github.com/ledgerwatch/turbo-geth/core/rawdb"
|
|
"github.com/ledgerwatch/turbo-geth/ethdb"
|
|
"github.com/ledgerwatch/turbo-geth/log"
|
|
)
|
|
|
|
func ValidateTxLookups(chaindata string) error {
|
|
db := ethdb.MustOpen(chaindata)
|
|
|
|
ch := make(chan os.Signal, 1)
|
|
quitCh := make(chan struct{})
|
|
signal.Notify(ch, os.Interrupt)
|
|
go func() {
|
|
<-ch
|
|
close(quitCh)
|
|
}()
|
|
t := time.Now()
|
|
defer func() {
|
|
log.Info("Validation ended", "it took", time.Since(t))
|
|
}()
|
|
var blockNum uint64
|
|
iterations := 0
|
|
var interrupt bool
|
|
// Validation Process
|
|
blockBytes := big.NewInt(0)
|
|
for !interrupt {
|
|
if err := common.Stopped(quitCh); err != nil {
|
|
return err
|
|
}
|
|
blockHash := rawdb.ReadCanonicalHash(db, blockNum)
|
|
body := rawdb.ReadBody(db, blockHash, blockNum)
|
|
|
|
if body == nil {
|
|
log.Error("Empty body", "blocknum", blockNum)
|
|
break
|
|
}
|
|
blockBytes.SetUint64(blockNum)
|
|
bn := blockBytes.Bytes()
|
|
|
|
for _, tx := range body.Transactions {
|
|
val, err := db.Get(dbutils.TxLookupPrefix, tx.Hash().Bytes())
|
|
iterations++
|
|
if iterations%100000 == 0 {
|
|
log.Info("Validated", "entries", iterations, "number", blockNum)
|
|
|
|
}
|
|
if !bytes.Equal(val, bn) {
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
panic(fmt.Sprintf("Validation process failed(%d). Expected %b, got %b", iterations, bn, val))
|
|
}
|
|
}
|
|
blockNum++
|
|
}
|
|
return nil
|
|
}
|