mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-29 07:07:16 +00:00
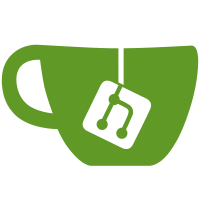
* Split History from Domain * Add History.prune * More on history * Fix HistoryHistory test * Merge history files * Scan file test for history * Add aggregator for erigon 2.2 * Change to generics, introduce contexts * Delete to belong to Aggregator * Fix lint * Fix lint * Fix lint * Fix lint * Use pointers to InvertedIndex again * Remove prints * Close embedded InvertedIndex * Fix closing files * Print * Update ci.yml * More printing * Fix * Make InvertedIndex pointer inside History * Fix * Update ci.yml * Remove print Co-authored-by: Alex Sharp <alexsharp@Alexs-MacBook-Pro.local> Co-authored-by: Alexey Sharp <alexeysharp@Alexeys-iMac.local>
77 lines
1.8 KiB
Go
77 lines
1.8 KiB
Go
/*
|
|
Copyright 2021 Erigon contributors
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
*/
|
|
|
|
package recsplit
|
|
|
|
import (
|
|
"sync"
|
|
|
|
"github.com/spaolacci/murmur3"
|
|
)
|
|
|
|
// IndexReader encapsulates Hash128 to allow concurrent access to Index
|
|
type IndexReader struct {
|
|
mu sync.RWMutex
|
|
hasher murmur3.Hash128
|
|
index *Index
|
|
}
|
|
|
|
// NewIndexReader creates new IndexReader
|
|
func NewIndexReader(index *Index) *IndexReader {
|
|
return &IndexReader{
|
|
hasher: murmur3.New128WithSeed(index.salt),
|
|
index: index,
|
|
}
|
|
}
|
|
|
|
func (r *IndexReader) sum(key []byte) (uint64, uint64) {
|
|
r.mu.Lock()
|
|
defer r.mu.Unlock()
|
|
r.hasher.Reset()
|
|
r.hasher.Write(key) //nolint:errcheck
|
|
return r.hasher.Sum128()
|
|
}
|
|
|
|
func (r *IndexReader) sum2(key1, key2 []byte) (uint64, uint64) {
|
|
r.mu.Lock()
|
|
defer r.mu.Unlock()
|
|
r.hasher.Reset()
|
|
r.hasher.Write(key1) //nolint:errcheck
|
|
r.hasher.Write(key2) //nolint:errcheck
|
|
return r.hasher.Sum128()
|
|
}
|
|
|
|
// Lookup wraps index Lookup
|
|
func (r *IndexReader) Lookup(key []byte) uint64 {
|
|
bucketHash, fingerprint := r.sum(key)
|
|
if r.index != nil {
|
|
return r.index.Lookup(bucketHash, fingerprint)
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (r *IndexReader) Lookup2(key1, key2 []byte) uint64 {
|
|
bucketHash, fingerprint := r.sum2(key1, key2)
|
|
if r.index != nil {
|
|
return r.index.Lookup(bucketHash, fingerprint)
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (r *IndexReader) Empty() bool {
|
|
return r.index.Empty()
|
|
}
|