mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2025-01-07 11:32:20 +00:00
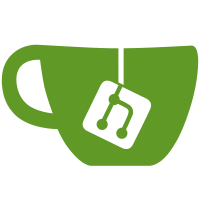
* adding eth_getLogs functionality for contract events for the devnet tool * Made changes to fix double hashing * Fixed lint errors * Changed strings.Replace to strings.ReplaceAll for replacing strings * Cleaned up print statements across files * Fixed logs not being populated in result typee.go Co-authored-by: Alexey Sharp <alexeysharp@Alexeys-iMac.local>
39 lines
757 B
Go
39 lines
757 B
Go
package requests
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"net/http"
|
|
"strconv"
|
|
"strings"
|
|
"time"
|
|
|
|
"github.com/ledgerwatch/log/v3"
|
|
)
|
|
|
|
func post(client *http.Client, url, request string, response interface{}) error {
|
|
start := time.Now()
|
|
r, err := client.Post(url, "application/json", strings.NewReader(request))
|
|
if err != nil {
|
|
return err
|
|
}
|
|
defer r.Body.Close()
|
|
|
|
if r.StatusCode != 200 {
|
|
return fmt.Errorf("status %s", r.Status)
|
|
}
|
|
|
|
decoder := json.NewDecoder(r.Body)
|
|
err = decoder.Decode(response)
|
|
log.Info("Got in", "time", time.Since(start).Seconds())
|
|
return err
|
|
}
|
|
|
|
func HexToInt(hexStr string) uint64 {
|
|
// Remove the 0x prefix
|
|
cleaned := strings.ReplaceAll(hexStr, "0x", "")
|
|
|
|
result, _ := strconv.ParseUint(cleaned, 16, 64)
|
|
return result
|
|
}
|