mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-25 21:17:16 +00:00
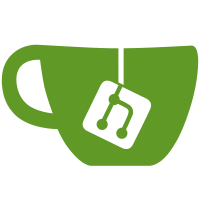
* Finish CmdSeek and add CmdNext * Add remoteDb listener and RPC daemon CLI * Fix test * Fix CLI * Fix lint * Fix unreachable code * Fix lint * First working RPC command eth_blockNumber * Fix lint * Fix lint * Fix memprofile/cpuprofile confusion * Add comment
83 lines
1.7 KiB
Go
83 lines
1.7 KiB
Go
package commands
|
|
|
|
import (
|
|
"fmt"
|
|
"io"
|
|
"os"
|
|
"runtime"
|
|
"runtime/pprof"
|
|
|
|
"github.com/spf13/cobra"
|
|
|
|
"github.com/ledgerwatch/turbo-geth/log"
|
|
)
|
|
|
|
var (
|
|
cpuprofile string
|
|
cpuProfileFile io.WriteCloser
|
|
|
|
memprofile string
|
|
)
|
|
|
|
func init() {
|
|
rootCmd.PersistentFlags().StringVar(&cpuprofile, "cpuprofile", "", "write cpu profile `file`")
|
|
rootCmd.PersistentFlags().StringVar(&memprofile, "memprofile", "", "write memory profile `file`")
|
|
}
|
|
|
|
var rootCmd = &cobra.Command{
|
|
Use: "state",
|
|
Short: "state is a utility for Stateless ethereum clients",
|
|
PersistentPreRun: func(cmd *cobra.Command, args []string) {
|
|
startProfilingIfNeeded()
|
|
|
|
},
|
|
PersistentPostRun: func(cmd *cobra.Command, args []string) {
|
|
stopProfilingIfNeeded()
|
|
},
|
|
}
|
|
|
|
func Execute() {
|
|
if err := rootCmd.Execute(); err != nil {
|
|
fmt.Println(err)
|
|
os.Exit(1)
|
|
}
|
|
}
|
|
|
|
func startProfilingIfNeeded() {
|
|
if cpuprofile != "" {
|
|
fmt.Println("starting CPU profiling")
|
|
cpuProfileFile, err := os.Create(cpuprofile)
|
|
if err != nil {
|
|
log.Error("could not create CPU profile", "error", err)
|
|
return
|
|
}
|
|
if err := pprof.StartCPUProfile(cpuProfileFile); err != nil {
|
|
log.Error("could not start CPU profile", "error", err)
|
|
return
|
|
}
|
|
}
|
|
}
|
|
|
|
func stopProfilingIfNeeded() {
|
|
if cpuprofile != "" {
|
|
fmt.Println("stopping CPU profiling")
|
|
pprof.StopCPUProfile()
|
|
}
|
|
|
|
if cpuProfileFile != nil {
|
|
cpuProfileFile.Close()
|
|
}
|
|
if memprofile != "" {
|
|
f, err := os.Create(memprofile)
|
|
if err != nil {
|
|
log.Error("could not create mem profile", "error", err)
|
|
return
|
|
}
|
|
runtime.GC() // get up-to-date statistics
|
|
if err := pprof.WriteHeapProfile(f); err != nil {
|
|
log.Error("could not write memory profile", "error", err)
|
|
return
|
|
}
|
|
}
|
|
}
|