mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2025-01-08 03:51:20 +00:00
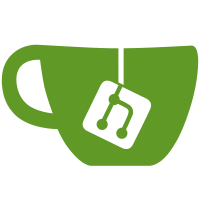
This is the initial merge for polygon milestones it implements an rpc call used by heimdall but does not directly impact any chain processing
52 lines
1.3 KiB
Go
52 lines
1.3 KiB
Go
package whitelist
|
|
|
|
import (
|
|
"github.com/ledgerwatch/erigon-lib/common"
|
|
"github.com/ledgerwatch/erigon/core/types"
|
|
"github.com/ledgerwatch/erigon/eth/borfinality/rawdb"
|
|
)
|
|
|
|
type checkpoint struct {
|
|
finality[*rawdb.Checkpoint]
|
|
}
|
|
type checkpointService interface {
|
|
finalityService
|
|
}
|
|
|
|
// TODO: Uncomment once metrics is added
|
|
// var (
|
|
// //Metrics for collecting the whitelisted milestone number
|
|
// whitelistedCheckpointNumberMeter = metrics.NewRegisteredGauge("chain/checkpoint/latest", nil)
|
|
|
|
// //Metrics for collecting the number of invalid chains received
|
|
// CheckpointChainMeter = metrics.NewRegisteredMeter("chain/checkpoint/isvalidchain", nil)
|
|
// )
|
|
|
|
// IsValidChain checks the validity of chain by comparing it
|
|
// against the local checkpoint entry
|
|
func (w *checkpoint) IsValidChain(currentHeader uint64, chain []*types.Header) bool {
|
|
w.finality.RLock()
|
|
defer w.finality.RUnlock()
|
|
|
|
res := w.finality.IsValidChain(currentHeader, chain)
|
|
|
|
// TODO: Uncomment once metrics is added
|
|
// if res {
|
|
// CheckpointChainMeter.Mark(int64(1))
|
|
// } else {
|
|
// CheckpointPeerMeter.Mark(int64(-1))
|
|
// }
|
|
|
|
return res
|
|
}
|
|
|
|
func (w *checkpoint) Process(block uint64, hash common.Hash) {
|
|
w.finality.Lock()
|
|
defer w.finality.Unlock()
|
|
|
|
w.finality.Process(block, hash)
|
|
|
|
// TODO: Uncomment once metrics is added
|
|
// whitelistedCheckpointNumberMeter.Update(int64(block))
|
|
}
|