mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-22 11:41:19 +00:00
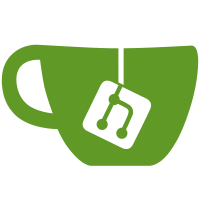
Added operation pools for beacon chain. operations are the equivalent of txs for eth2 Added operation pools for: * Attester Slashings * Proposer Slashings * VoluntaryExits * BLSExecutionToChange * Postponed to later: Attestations (or maybe not)
36 lines
928 B
Go
36 lines
928 B
Go
package pool
|
|
|
|
import (
|
|
"github.com/ledgerwatch/erigon/cl/phase1/core/state/lru"
|
|
)
|
|
|
|
var operationsMultiplier = 20 // Cap the amount of cached element to max_operations_per_block * operations_multiplier
|
|
|
|
type OperationPool[K comparable, T any] struct {
|
|
pool *lru.Cache[K, T] // Map the Signature to the underlying object
|
|
}
|
|
|
|
func NewOperationPool[K comparable, T any](maxOperationsPerBlock int, matricName string) *OperationPool[K, T] {
|
|
pool, err := lru.New[K, T](matricName, maxOperationsPerBlock*operationsMultiplier)
|
|
if err != nil {
|
|
panic(err)
|
|
}
|
|
return &OperationPool[K, T]{pool: pool}
|
|
}
|
|
|
|
func (o *OperationPool[K, T]) Insert(k K, operation T) {
|
|
o.pool.Add(k, operation)
|
|
}
|
|
|
|
func (o *OperationPool[K, T]) DeleteIfExist(k K) (removed bool) {
|
|
return o.pool.Remove(k)
|
|
}
|
|
|
|
func (o *OperationPool[K, T]) Has(k K) (hash bool) {
|
|
return o.pool.Contains(k)
|
|
}
|
|
|
|
func (o *OperationPool[K, T]) Raw() []T {
|
|
return o.pool.Values()
|
|
}
|