mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2025-01-06 02:52:19 +00:00
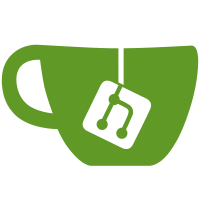
* saving * Implemented and tested subscription of logs * Fixed lint errors * fixed compilation error * Removed print statements across code * made review changes * Validated hex addresses
37 lines
930 B
Go
37 lines
930 B
Go
package requests
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"strconv"
|
|
"strings"
|
|
)
|
|
|
|
// HexToInt converts a hex string to a type uint64
|
|
func HexToInt(hexStr string) uint64 {
|
|
// Remove the 0x prefix
|
|
cleaned := strings.ReplaceAll(hexStr, "0x", "")
|
|
|
|
result, _ := strconv.ParseUint(cleaned, 16, 64)
|
|
return result
|
|
}
|
|
|
|
// parseResponse converts any of the rpctest interfaces to a string for readability
|
|
func parseResponse(resp interface{}) (string, error) {
|
|
result, err := json.Marshal(resp)
|
|
if err != nil {
|
|
return "", fmt.Errorf("error trying to marshal response: %v", err)
|
|
}
|
|
|
|
return string(result), nil
|
|
}
|
|
|
|
// NamespaceAndSubMethodFromMethod splits a parent method into namespace and the actual method
|
|
func NamespaceAndSubMethodFromMethod(method string) (string, string, error) {
|
|
parts := strings.SplitN(method, "_", 2)
|
|
if len(parts) != 2 {
|
|
return "", "", fmt.Errorf("invalid string to split")
|
|
}
|
|
return parts[0], parts[1], nil
|
|
}
|