mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2025-01-06 02:52:19 +00:00
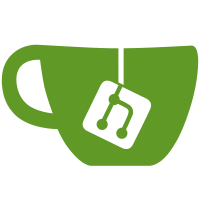
* save state * snapshot update works * save state * snapshot migrator * tx test * save state * migrations stages refactor * refactor snapshot migrator * compilation fixed * integrate snapshot migrator * goerli sync headers * debug async snapshotter on goerly * move verify headers, remove experiments, fix remove old snapshot * save state * refactor snapshotsync injection * fix deadlock * replace snapshot generation stage logic to migrate method * change done for body snapshot * clean * clean&&change deleted value * clean * fix hash len * fix hash len * remove one of wrap methods, add remove snapshots on start * add err check * fix shadowing * stages unwind order debug * matryoshka experiments * steam test * fix build * fix test * fix lint * fix test * fix test datarace * add get test * return timeout * fix mdbx overlap * fix after merge * change epoch size * clean todo * fix * return testdata * added return from sndownloader gorutine * fix review comments * Fix * More info Co-authored-by: Alex Sharp <alexsharp@Alexs-MacBook-Pro.local>
82 lines
2.8 KiB
Go
82 lines
2.8 KiB
Go
package commands
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
"io/ioutil"
|
|
"os"
|
|
"time"
|
|
|
|
"github.com/ledgerwatch/lmdb-go/lmdb"
|
|
"github.com/ledgerwatch/turbo-geth/common/dbutils"
|
|
"github.com/ledgerwatch/turbo-geth/core/rawdb"
|
|
"github.com/ledgerwatch/turbo-geth/eth/stagedsync"
|
|
"github.com/ledgerwatch/turbo-geth/ethdb"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
func init() {
|
|
withDatadir(verifyStateSnapshotCmd)
|
|
withSnapshotFile(verifyStateSnapshotCmd)
|
|
withBlock(verifyStateSnapshotCmd)
|
|
|
|
rootCmd.AddCommand(verifyStateSnapshotCmd)
|
|
}
|
|
|
|
//
|
|
var verifyStateSnapshotCmd = &cobra.Command{
|
|
Use: "verify_state",
|
|
Short: "Verify state snapshot",
|
|
Example: "go run cmd/snapshots/generator/main.go verify_state --block 11000000 --snapshot /media/b00ris/nvme/snapshots/state/ --datadir /media/b00ris/nvme/backup/snapshotsync/",
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
return VerifyStateSnapshot(cmd.Context(), chaindata, snapshotFile, block)
|
|
},
|
|
}
|
|
|
|
func VerifyStateSnapshot(ctx context.Context, dbPath, snapshotPath string, block uint64) error {
|
|
snkv := ethdb.NewLMDB().WithBucketsConfig(func(defaultBuckets dbutils.BucketsCfg) dbutils.BucketsCfg {
|
|
return dbutils.BucketsCfg{
|
|
dbutils.PlainStateBucket: dbutils.BucketsConfigs[dbutils.PlainStateBucket],
|
|
dbutils.PlainContractCodeBucket: dbutils.BucketsConfigs[dbutils.PlainContractCodeBucket],
|
|
dbutils.CodeBucket: dbutils.BucketsConfigs[dbutils.CodeBucket],
|
|
}
|
|
}).Path(snapshotPath).Flags(func(flags uint) uint { return flags | lmdb.Readonly }).MustOpen()
|
|
|
|
tmpPath, err := ioutil.TempDir(os.TempDir(), "vrf*")
|
|
if err != nil {
|
|
return err
|
|
}
|
|
tmpDB := ethdb.NewLMDB().Path(tmpPath).MustOpen()
|
|
defer os.RemoveAll(tmpPath)
|
|
defer tmpDB.Close()
|
|
snkv = ethdb.NewSnapshotKV().SnapshotDB([]string{dbutils.PlainStateBucket, dbutils.PlainContractCodeBucket, dbutils.CodeBucket}, snkv).DB(tmpDB).Open()
|
|
sndb := ethdb.NewObjectDatabase(snkv)
|
|
tx, err := sndb.Begin(context.Background(), ethdb.RW)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
defer tx.Rollback()
|
|
hash, err := rawdb.ReadCanonicalHash(tx, block)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
syncHeadHeader := rawdb.ReadHeader(tx, hash, block)
|
|
if syncHeadHeader == nil {
|
|
return fmt.Errorf("empty header")
|
|
}
|
|
expectedRootHash := syncHeadHeader.Root
|
|
tt := time.Now()
|
|
err = stagedsync.PromoteHashedStateCleanly("", tx.(ethdb.HasTx).Tx().(ethdb.RwTx), stagedsync.StageHashStateCfg(sndb.RwKV(), os.TempDir()), ctx.Done())
|
|
fmt.Println("Promote took", time.Since(tt))
|
|
if err != nil {
|
|
return fmt.Errorf("promote state err: %w", err)
|
|
}
|
|
|
|
_, err = stagedsync.RegenerateIntermediateHashes("", tx.(ethdb.HasTx).Tx().(ethdb.RwTx), stagedsync.StageTrieCfg(sndb.RwKV(), true, true, os.TempDir()), expectedRootHash, ctx.Done())
|
|
if err != nil {
|
|
return fmt.Errorf("regenerateIntermediateHashes err: %w", err)
|
|
}
|
|
return nil
|
|
}
|