mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-26 13:40:05 +00:00
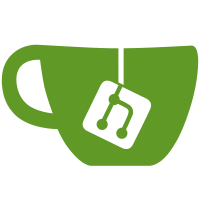
* save state * save state * save state * refactoring * fix * save state * save state * fmt * fix lint * restore torrents for external downloader * fix lint * download * skip debug test * debug * remote debug * small cli fixes * skip debug test * external snapshot predownloader * get rid of remote downloader * fix lint * clean makefile * fix lint * fix lint * cleanup * fix ci * fmt * remove proto from interfaces * Squashed 'interfaces/' content from commit acd02bb94 git-subtree-dir: interfaces git-subtree-split: acd02bb94c5a421aa8f8d1fd76cd8aad668e9fcb
82 lines
1.4 KiB
Go
82 lines
1.4 KiB
Go
package snapshotsync
|
|
|
|
import "fmt"
|
|
|
|
var DefaultSnapshotMode = SnapshotMode{}
|
|
|
|
type SnapshotMode struct {
|
|
Headers bool
|
|
Bodies bool
|
|
State bool
|
|
Receipts bool
|
|
}
|
|
|
|
func (m SnapshotMode) ToString() string {
|
|
var mode string
|
|
if m.Headers {
|
|
mode += "h"
|
|
}
|
|
if m.Bodies {
|
|
mode += "b"
|
|
}
|
|
if m.State {
|
|
mode += "s"
|
|
}
|
|
if m.Receipts {
|
|
mode += "r"
|
|
}
|
|
return mode
|
|
}
|
|
|
|
func (m SnapshotMode) ToSnapshotTypes() []SnapshotType {
|
|
var types []SnapshotType
|
|
if m.Headers {
|
|
types = append(types, SnapshotType_headers)
|
|
}
|
|
if m.Bodies {
|
|
types = append(types, SnapshotType_bodies)
|
|
}
|
|
if m.State {
|
|
types = append(types, SnapshotType_state)
|
|
}
|
|
if m.Receipts {
|
|
types = append(types, SnapshotType_receipts)
|
|
}
|
|
return types
|
|
}
|
|
|
|
func FromSnapshotTypes(st []SnapshotType) SnapshotMode {
|
|
var mode SnapshotMode
|
|
for i := range st {
|
|
switch st[i] {
|
|
case SnapshotType_headers:
|
|
mode.Headers = true
|
|
case SnapshotType_bodies:
|
|
mode.Bodies = true
|
|
case SnapshotType_state:
|
|
mode.State = true
|
|
case SnapshotType_receipts:
|
|
mode.Receipts = true
|
|
}
|
|
}
|
|
return mode
|
|
}
|
|
func SnapshotModeFromString(flags string) (SnapshotMode, error) {
|
|
mode := SnapshotMode{}
|
|
for _, flag := range flags {
|
|
switch flag {
|
|
case 'h':
|
|
mode.Headers = true
|
|
case 'b':
|
|
mode.Bodies = true
|
|
case 's':
|
|
mode.State = true
|
|
case 'r':
|
|
mode.Receipts = true
|
|
default:
|
|
return mode, fmt.Errorf("unexpected flag found: %c", flag)
|
|
}
|
|
}
|
|
return mode, nil
|
|
}
|