mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2025-01-05 10:32:19 +00:00
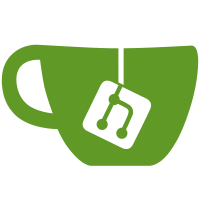
* fix "genesis hash does not match" when dev nodes connect The "dev" nodes need to have the same --miner.etherbase in order to generate the same genesis ExtraData by DeveloperGenesisBlock(). Override DevnetEtherbase global var that's used if --miner.etherbase is not passed. (for NonBlockProducer case) * fix missing private key for the hardcoded DevnetEtherbase Fixes panic if SigKey is not found. Bor non-producers will use a default `DevnetEtherbase` while Dev nodes modify it. Save hardcoded DevnetEtherbase/DevnetSignPrivateKey into accounts so that SigKey can recover it. * refactor devnet.node to contain Node config This avoids interface{} type casts and fixes an error with Heimdall.validatorSet == nil * add connection retries to rpcCall and Subscribe of requestGenerator Fixes "connection refused" errors due to node not ready to handle early RPC requests. * fix deadlock in Heimdall.NodeStarted * fix GetBlockByNumber Fixes "cannot unmarshal string into Go struct field body.transactions of type jsonrpc.RPCTransaction" * demote "no of blocks on childchain is less than confirmations required" to Info (#8626) * demote "mismatched pending subpool size" to Debug (#8615) * revert wiggle testing code
63 lines
1.5 KiB
Go
63 lines
1.5 KiB
Go
package requests
|
|
|
|
import (
|
|
"fmt"
|
|
)
|
|
|
|
type EthTxPool struct {
|
|
CommonResponse
|
|
Result interface{} `json:"result"`
|
|
}
|
|
|
|
func (reqGen *requestGenerator) TxpoolContent() (int, int, int, error) {
|
|
var (
|
|
b EthTxPool
|
|
pending map[string]interface{}
|
|
queued map[string]interface{}
|
|
baseFee map[string]interface{}
|
|
)
|
|
|
|
method, body := reqGen.txpoolContent()
|
|
if res := reqGen.rpcCallJSON(method, body, &b); res.Err != nil {
|
|
return len(pending), len(queued), len(baseFee), fmt.Errorf("failed to fetch txpool content: %v", res.Err)
|
|
}
|
|
|
|
resp, ok := b.Result.(map[string]interface{})
|
|
|
|
if !ok {
|
|
return 0, 0, 0, fmt.Errorf("Unexpected result type: %T", b.Result)
|
|
}
|
|
|
|
pendingLen := 0
|
|
queuedLen := 0
|
|
baseFeeLen := 0
|
|
|
|
if resp["pending"] != nil {
|
|
pending = resp["pending"].(map[string]interface{})
|
|
for _, txs := range pending { // iterate over senders
|
|
pendingLen += len(txs.(map[string]interface{}))
|
|
}
|
|
}
|
|
|
|
if resp["queue"] != nil {
|
|
queued = resp["queue"].(map[string]interface{})
|
|
for _, txs := range queued {
|
|
queuedLen += len(txs.(map[string]interface{}))
|
|
}
|
|
}
|
|
|
|
if resp["baseFee"] != nil {
|
|
baseFee = resp["baseFee"].(map[string]interface{})
|
|
for _, txs := range baseFee {
|
|
baseFeeLen += len(txs.(map[string]interface{}))
|
|
}
|
|
}
|
|
|
|
return pendingLen, queuedLen, baseFeeLen, nil
|
|
}
|
|
|
|
func (req *requestGenerator) txpoolContent() (RPCMethod, string) {
|
|
const template = `{"jsonrpc":"2.0","method":%q,"params":[],"id":%d}`
|
|
return Methods.TxpoolContent, fmt.Sprintf(template, Methods.TxpoolContent, req.reqID)
|
|
}
|