mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-22 11:41:19 +00:00
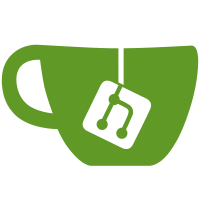
So there is an issue with tracing certain blocks/transactions on Polygon, for example: ``` > '{"method": "trace_transaction","params":["0xb198d93f640343a98f90d93aa2b74b4fc5c64f3a649f1608d2bfd1004f9dee0e"],"id":1,"jsonrpc":"2.0"}' ``` gives the error `first run for txIndex 1 error: insufficient funds for gas * price + value: address 0x10AD27A96CDBffC90ab3b83bF695911426A69f5E have 16927727762862809 want 17594166808296934` The reason is that this transaction is from the author of the block, which doesn't have enough ETH to pay for the gas fee + tx value if he's not the block author receiving transactions fees. The issue is that currently the APIs are using `ethash.NewFaker()` Engine for running traces, etc. which doesn't know how to get the author for a specific block (which is consensus dependant); as it was noting in several TODO comments. The fix is to pass the Engine to the BaseAPI, which can then be used to create the right Block Context. I chose to split the current Engine interface in 2, with Reader and Writer, so that the BaseAPI only receives the Reader one, which might be safer (even though it's only used for getting the block Author).
150 lines
4.9 KiB
Go
150 lines
4.9 KiB
Go
// Copyright 2016 The go-ethereum Authors
|
|
// This file is part of the go-ethereum library.
|
|
//
|
|
// The go-ethereum library is free software: you can redistribute it and/or modify
|
|
// it under the terms of the GNU Lesser General Public License as published by
|
|
// the Free Software Foundation, either version 3 of the License, or
|
|
// (at your option) any later version.
|
|
//
|
|
// The go-ethereum library is distributed in the hope that it will be useful,
|
|
// but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
// MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
// GNU Lesser General Public License for more details.
|
|
//
|
|
// You should have received a copy of the GNU Lesser General Public License
|
|
// along with the go-ethereum library. If not, see <http://www.gnu.org/licenses/>.
|
|
|
|
package core
|
|
|
|
import (
|
|
"fmt"
|
|
"math/big"
|
|
|
|
"github.com/holiman/uint256"
|
|
"github.com/ledgerwatch/erigon/common"
|
|
"github.com/ledgerwatch/erigon/consensus"
|
|
"github.com/ledgerwatch/erigon/consensus/serenity"
|
|
"github.com/ledgerwatch/erigon/core/types"
|
|
"github.com/ledgerwatch/erigon/core/vm/evmtypes"
|
|
"github.com/ledgerwatch/erigon/params"
|
|
)
|
|
|
|
// NewEVMBlockContext creates a new context for use in the EVM.
|
|
func NewEVMBlockContext(header *types.Header, blockHashFunc func(n uint64) common.Hash, engine consensus.EngineReader, author *common.Address) evmtypes.BlockContext {
|
|
// If we don't have an explicit author (i.e. not mining), extract from the header
|
|
var beneficiary common.Address
|
|
if author == nil {
|
|
beneficiary, _ = engine.Author(header) // Ignore error, we're past header validation
|
|
} else {
|
|
beneficiary = *author
|
|
}
|
|
var baseFee uint256.Int
|
|
if header.BaseFee != nil {
|
|
overflow := baseFee.SetFromBig(header.BaseFee)
|
|
if overflow {
|
|
panic(fmt.Errorf("header.BaseFee higher than 2^256-1"))
|
|
}
|
|
}
|
|
|
|
var prevRandDao *common.Hash
|
|
if header.Difficulty.Cmp(serenity.SerenityDifficulty) == 0 {
|
|
// EIP-4399. We use SerenityDifficulty (i.e. 0) as a telltale of Proof-of-Stake blocks.
|
|
prevRandDao = &header.MixDigest
|
|
}
|
|
|
|
var transferFunc evmtypes.TransferFunc
|
|
if engine != nil && engine.Type() == params.BorConsensus {
|
|
transferFunc = BorTransfer
|
|
} else {
|
|
transferFunc = Transfer
|
|
}
|
|
|
|
return evmtypes.BlockContext{
|
|
CanTransfer: CanTransfer,
|
|
Transfer: transferFunc,
|
|
GetHash: blockHashFunc,
|
|
Coinbase: beneficiary,
|
|
BlockNumber: header.Number.Uint64(),
|
|
Time: header.Time,
|
|
Difficulty: new(big.Int).Set(header.Difficulty),
|
|
BaseFee: &baseFee,
|
|
GasLimit: header.GasLimit,
|
|
PrevRanDao: prevRandDao,
|
|
}
|
|
}
|
|
|
|
// NewEVMTxContext creates a new transaction context for a single transaction.
|
|
func NewEVMTxContext(msg Message) evmtypes.TxContext {
|
|
return evmtypes.TxContext{
|
|
Origin: msg.From(),
|
|
GasPrice: msg.GasPrice(),
|
|
}
|
|
}
|
|
|
|
// GetHashFn returns a GetHashFunc which retrieves header hashes by number
|
|
func GetHashFn(ref *types.Header, getHeader func(hash common.Hash, number uint64) *types.Header) func(n uint64) common.Hash {
|
|
// Cache will initially contain [refHash.parent],
|
|
// Then fill up with [refHash.p, refHash.pp, refHash.ppp, ...]
|
|
var cache []common.Hash
|
|
|
|
return func(n uint64) common.Hash {
|
|
// If there's no hash cache yet, make one
|
|
if len(cache) == 0 {
|
|
cache = append(cache, ref.ParentHash)
|
|
}
|
|
if idx := ref.Number.Uint64() - n - 1; idx < uint64(len(cache)) {
|
|
return cache[idx]
|
|
}
|
|
// No luck in the cache, but we can start iterating from the last element we already know
|
|
lastKnownHash := cache[len(cache)-1]
|
|
lastKnownNumber := ref.Number.Uint64() - uint64(len(cache))
|
|
|
|
for {
|
|
header := getHeader(lastKnownHash, lastKnownNumber)
|
|
if header == nil {
|
|
break
|
|
}
|
|
cache = append(cache, header.ParentHash)
|
|
lastKnownHash = header.ParentHash
|
|
lastKnownNumber = header.Number.Uint64() - 1
|
|
if n == lastKnownNumber {
|
|
return lastKnownHash
|
|
}
|
|
}
|
|
return common.Hash{}
|
|
}
|
|
}
|
|
|
|
// CanTransfer checks whether there are enough funds in the address' account to make a transfer.
|
|
// This does not take the necessary gas in to account to make the transfer valid.
|
|
func CanTransfer(db evmtypes.IntraBlockState, addr common.Address, amount *uint256.Int) bool {
|
|
return !db.GetBalance(addr).Lt(amount)
|
|
}
|
|
|
|
// Transfer subtracts amount from sender and adds amount to recipient using the given Db
|
|
func Transfer(db evmtypes.IntraBlockState, sender, recipient common.Address, amount *uint256.Int, bailout bool) {
|
|
if !bailout {
|
|
db.SubBalance(sender, amount)
|
|
}
|
|
db.AddBalance(recipient, amount)
|
|
}
|
|
|
|
// BorTransfer transfer in Bor
|
|
func BorTransfer(db evmtypes.IntraBlockState, sender, recipient common.Address, amount *uint256.Int, bailout bool) {
|
|
// get inputs before
|
|
input1 := db.GetBalance(sender).Clone()
|
|
input2 := db.GetBalance(recipient).Clone()
|
|
|
|
if !bailout {
|
|
db.SubBalance(sender, amount)
|
|
}
|
|
db.AddBalance(recipient, amount)
|
|
|
|
// get outputs after
|
|
output1 := db.GetBalance(sender).Clone()
|
|
output2 := db.GetBalance(recipient).Clone()
|
|
|
|
// add transfer log
|
|
AddTransferLog(db, sender, recipient, amount, input1, input2, output1, output2)
|
|
}
|