mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-25 04:57:17 +00:00
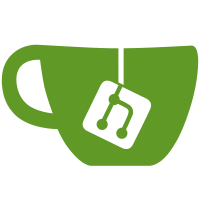
* implemented crash reporting for all goroutine panics that aren't handled explicitly * implemented crash reporting for all goroutine panics that aren't handled explicitly * changed node defaults back to originals after testing * implemented panic handling for all goroutines that don't explicitly handle them, outputting the stack trace to a file in crashreports * handling panics on all goroutines gracefully * updated missing call * error assignment * implemented suggestions * path.Join added * implemented Evgeny's suggestions * changed path.Join to filepath.Join for cross-platform * added err check * updated RecoverStackTrace to LogPanic * updated closures * removed call of common.Go to some goroutines * updated scope capture * removed testing files * reverted back to original method, I feel like its less intrusive * update filename for clarity
46 lines
1.3 KiB
Go
46 lines
1.3 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"net"
|
|
"os"
|
|
|
|
"github.com/ledgerwatch/erigon/cmd/utils"
|
|
"github.com/ledgerwatch/erigon/common/debug"
|
|
"github.com/ledgerwatch/erigon/log"
|
|
"github.com/ledgerwatch/erigon/params"
|
|
erigoncli "github.com/ledgerwatch/erigon/turbo/cli"
|
|
"github.com/ledgerwatch/erigon/turbo/node"
|
|
"github.com/urfave/cli"
|
|
)
|
|
|
|
func main() {
|
|
// catch panics from main thread and logs stack trace into a file
|
|
defer func() { debug.LogPanic(nil, true, recover()) }()
|
|
// creating a erigon-api app with all defaults
|
|
app := erigoncli.MakeApp(runErigon, erigoncli.DefaultFlags)
|
|
if err := app.Run(os.Args); err != nil {
|
|
fmt.Fprintln(os.Stderr, err)
|
|
os.Exit(1)
|
|
}
|
|
}
|
|
|
|
func runErigon(cliCtx *cli.Context) {
|
|
// creating staged sync with all default parameters
|
|
ctx, _ := utils.RootContext()
|
|
|
|
// initializing the node and providing the current git commit there
|
|
log.Info("Build info", "git_branch", params.GitBranch, "git_tag", params.GitTag, "git_commit", params.GitCommit)
|
|
eri := node.New(cliCtx, node.Params{})
|
|
eri.SetP2PListenFunc(func(network, addr string) (net.Listener, error) {
|
|
var lc net.ListenConfig
|
|
return lc.Listen(ctx, network, addr)
|
|
})
|
|
// running the node
|
|
err := eri.Serve()
|
|
|
|
if err != nil {
|
|
log.Error("error while serving a Erigon node", "err", err)
|
|
}
|
|
}
|