mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2025-01-05 18:42:19 +00:00
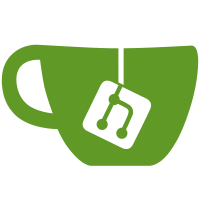
* Erigon2 upgrade 2 prototype * Latest erigon-lib * Fixes * Fix print * Fix maxSpan * Reduce maxSpan * Remove duplicate joins * TxNum * Fix resuming * first draft of history22 * Introduce historical reads * Update to erigon-lib * Update erigon-lib * Update erigon-lib * Fixes and tracing for checkChangeSets * More trace * Print account details * fix getHeader * Update to erigon-lib main * Add tracer indices and event log indices * Fix calltracer * Fix calltracer * Duplicate rpcdaemon into rpcdaemon22 * Fix tests * Fix tests * Fix tests * Update to latest erigon-lib Co-authored-by: Alexey Sharp <alexeysharp@Alexeys-iMac.local> Co-authored-by: Alex Sharp <alexsharp@Alexs-MacBook-Pro.local>
58 lines
1.7 KiB
Go
58 lines
1.7 KiB
Go
package commands
|
|
|
|
import (
|
|
"math/big"
|
|
"time"
|
|
|
|
libstate "github.com/ledgerwatch/erigon-lib/state"
|
|
"github.com/ledgerwatch/erigon/common"
|
|
"github.com/ledgerwatch/erigon/core/vm"
|
|
)
|
|
|
|
type CallTracer struct {
|
|
froms map[common.Address]struct{}
|
|
tos map[common.Address]struct{}
|
|
}
|
|
|
|
func NewCallTracer() *CallTracer {
|
|
return &CallTracer{
|
|
froms: map[common.Address]struct{}{},
|
|
tos: map[common.Address]struct{}{},
|
|
}
|
|
}
|
|
|
|
func (ct *CallTracer) CaptureStart(evm *vm.EVM, depth int, from common.Address, to common.Address, precompile bool, create bool, calltype vm.CallType, input []byte, gas uint64, value *big.Int, code []byte) {
|
|
ct.froms[from] = struct{}{}
|
|
ct.tos[to] = struct{}{}
|
|
}
|
|
func (ct *CallTracer) CaptureState(env *vm.EVM, pc uint64, op vm.OpCode, gas, cost uint64, scope *vm.ScopeContext, rData []byte, depth int, err error) {
|
|
}
|
|
func (ct *CallTracer) CaptureFault(env *vm.EVM, pc uint64, op vm.OpCode, gas, cost uint64, scope *vm.ScopeContext, depth int, err error) {
|
|
}
|
|
func (ct *CallTracer) CaptureEnd(depth int, output []byte, startGas, endGas uint64, t time.Duration, err error) {
|
|
}
|
|
func (ct *CallTracer) CaptureSelfDestruct(from common.Address, to common.Address, value *big.Int) {
|
|
ct.froms[from] = struct{}{}
|
|
ct.tos[to] = struct{}{}
|
|
}
|
|
func (ct *CallTracer) CaptureAccountRead(account common.Address) error {
|
|
return nil
|
|
}
|
|
func (ct *CallTracer) CaptureAccountWrite(account common.Address) error {
|
|
return nil
|
|
}
|
|
|
|
func (ct *CallTracer) AddToAggregator(a *libstate.Aggregator) error {
|
|
for from := range ct.froms {
|
|
if err := a.AddTraceFrom(from[:]); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
for to := range ct.tos {
|
|
if err := a.AddTraceTo(to[:]); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}
|