mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-24 20:47:16 +00:00
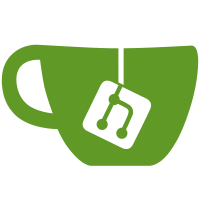
* Initial commit for CallTraces index * Fix compilation * fix lint, add comment * Fix integration * Add Close function to ethdb.Cursor, fix some compile errors * Try to stop cursor leak in Get * Fix compile errors in RPC daemon * Fix compile errors * fixing another way * Some fixes * More fixes * More fixes * More fixes * Fixes to core/state * Fix lint * Fix lint * Fixes * Stage caching for call trace stage * Add mem stats * Try to stop the leak * Turn off debug * Chunks for 10k blocks * Print * Revert "Print" This reverts commit 5ffada4828d61e00e5dad1ca12c98258dfbbad00. * Revert "Chunks for 10k blocks" This reverts commit cfb9d498e782e5583d41c30abf0e2137da27383e. * Trying to fix the leak * Don't compute receipts in re-tracing * Not compose block * Print speed, fix receipts, bigger caches * Fix lint * Utilise changeset info * Counters * Use NoReceipts and ReadOnly * ReadOnly is incompatible with caching * Skip test leaking transactions * Fix block test * Change disable message for call-traces stage * Use block option for call traces integration * Fix retracing due to incarnation
38 lines
1.2 KiB
Go
38 lines
1.2 KiB
Go
package commands
|
|
|
|
import (
|
|
"context"
|
|
"fmt"
|
|
|
|
"github.com/ledgerwatch/turbo-geth/common"
|
|
"github.com/ledgerwatch/turbo-geth/core/rawdb"
|
|
"github.com/ledgerwatch/turbo-geth/eth"
|
|
"github.com/ledgerwatch/turbo-geth/params"
|
|
"github.com/ledgerwatch/turbo-geth/turbo/adapter"
|
|
"github.com/ledgerwatch/turbo-geth/turbo/transactions"
|
|
)
|
|
|
|
// TraceTransaction returns the structured logs created during the execution of EVM
|
|
// and returns them as a JSON object.
|
|
func (api *PrivateDebugAPIImpl) TraceTransaction(ctx context.Context, hash common.Hash, config *eth.TraceConfig) (interface{}, error) {
|
|
tx, err := api.db.Begin(ctx, nil, false)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
defer tx.Rollback()
|
|
|
|
// Retrieve the transaction and assemble its EVM context
|
|
txn, blockHash, _, txIndex := rawdb.ReadTransaction(tx, hash)
|
|
if txn == nil {
|
|
return nil, fmt.Errorf("transaction %#x not found", hash)
|
|
}
|
|
getter := adapter.NewBlockGetter(tx)
|
|
chainContext := adapter.NewChainContext(tx)
|
|
msg, vmctx, ibs, _, err := transactions.ComputeTxEnv(ctx, getter, params.MainnetChainConfig, chainContext, tx, blockHash, txIndex)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
// Trace the transaction and return
|
|
return transactions.TraceTx(ctx, msg, vmctx, ibs, config)
|
|
}
|