mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2025-01-10 13:01:21 +00:00
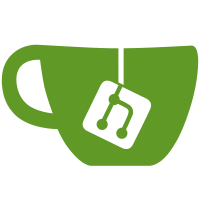
This is the initial merge for polygon milestones it implements an rpc call used by heimdall but does not directly impact any chain processing
38 lines
2.0 KiB
Go
38 lines
2.0 KiB
Go
package bor
|
|
|
|
import (
|
|
"context"
|
|
|
|
"github.com/ledgerwatch/erigon/consensus/bor/clerk"
|
|
"github.com/ledgerwatch/erigon/consensus/bor/heimdall/checkpoint"
|
|
"github.com/ledgerwatch/erigon/consensus/bor/heimdall/milestone"
|
|
"github.com/ledgerwatch/erigon/consensus/bor/heimdall/span"
|
|
)
|
|
|
|
//go:generate mockgen -destination=../../tests/bor/mocks/IHeimdallClient.go -package=mocks . IHeimdallClient
|
|
type IHeimdallClient interface {
|
|
StateSyncEvents(ctx context.Context, fromID uint64, to int64) ([]*clerk.EventRecordWithTime, error)
|
|
Span(ctx context.Context, spanID uint64) (*span.HeimdallSpan, error)
|
|
FetchCheckpoint(ctx context.Context, number int64) (*checkpoint.Checkpoint, error)
|
|
FetchCheckpointCount(ctx context.Context) (int64, error)
|
|
FetchMilestone(ctx context.Context) (*milestone.Milestone, error)
|
|
FetchMilestoneCount(ctx context.Context) (int64, error)
|
|
FetchNoAckMilestone(ctx context.Context, milestoneID string) error //Fetch the bool value whether milestone corresponding to the given id failed in the Heimdall
|
|
FetchLastNoAckMilestone(ctx context.Context) (string, error) //Fetch latest failed milestone id
|
|
FetchMilestoneID(ctx context.Context, milestoneID string) error //Fetch the bool value whether milestone corresponding to the given id is in process in Heimdall
|
|
Close()
|
|
}
|
|
|
|
type HeimdallServer interface {
|
|
StateSyncEvents(ctx context.Context, fromID uint64, to int64, limit int) (uint64, []*clerk.EventRecordWithTime, error)
|
|
Span(ctx context.Context, spanID uint64) (*span.HeimdallSpan, error)
|
|
FetchCheckpoint(ctx context.Context, number int64) (*checkpoint.Checkpoint, error)
|
|
FetchCheckpointCount(ctx context.Context) (int64, error)
|
|
FetchMilestone(ctx context.Context) (*milestone.Milestone, error)
|
|
FetchMilestoneCount(ctx context.Context) (int64, error)
|
|
FetchNoAckMilestone(ctx context.Context, milestoneID string) error
|
|
FetchLastNoAckMilestone(ctx context.Context) (string, error)
|
|
FetchMilestoneID(ctx context.Context, milestoneID string) error
|
|
Close()
|
|
}
|