mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-22 19:50:36 +00:00
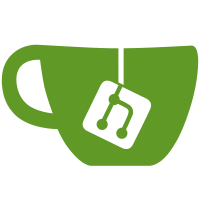
Starting from [Shanghai](https://github.com/ethereum/execution-specs/blob/master/network-upgrades/mainnet-upgrades/shanghai.md), forks are based on timestamps rather than block heights (see PR #6238). This PR extends [EIP-2124](https://eips.ethereum.org/EIPS/eip-2124) Fork ID to include timestamp-based blocks. See also https://github.com/ethereum/go-ethereum/pull/25878. Co-authored-by: Marius van der Wijden <m.vanderwijden@live.de>
28 lines
475 B
Go
28 lines
475 B
Go
package common
|
|
|
|
import (
|
|
"golang.org/x/exp/constraints"
|
|
"golang.org/x/exp/slices"
|
|
)
|
|
|
|
func SortedKeys[K constraints.Ordered, V any](m map[K]V) []K {
|
|
keys := make([]K, len(m))
|
|
i := 0
|
|
for k := range m {
|
|
keys[i] = k
|
|
i++
|
|
}
|
|
slices.Sort(keys)
|
|
return keys
|
|
}
|
|
|
|
func RemoveDuplicatesFromSorted[T constraints.Ordered](slice []T) []T {
|
|
for i := 1; i < len(slice); i++ {
|
|
if slice[i] == slice[i-1] {
|
|
slice = append(slice[:i], slice[i+1:]...)
|
|
i--
|
|
}
|
|
}
|
|
return slice
|
|
}
|