mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-25 21:17:16 +00:00
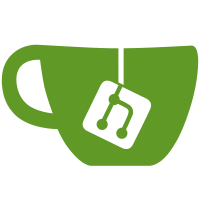
* Initial commit for CallTraces index * Fix compilation * fix lint, add comment * Fix integration * Add Close function to ethdb.Cursor, fix some compile errors * Try to stop cursor leak in Get * Fix compile errors in RPC daemon * Fix compile errors * fixing another way * Some fixes * More fixes * More fixes * More fixes * Fixes to core/state * Fix lint * Fix lint * Fixes * Stage caching for call trace stage * Add mem stats * Try to stop the leak * Turn off debug * Chunks for 10k blocks * Print * Revert "Print" This reverts commit 5ffada4828d61e00e5dad1ca12c98258dfbbad00. * Revert "Chunks for 10k blocks" This reverts commit cfb9d498e782e5583d41c30abf0e2137da27383e. * Trying to fix the leak * Don't compute receipts in re-tracing * Not compose block * Print speed, fix receipts, bigger caches * Fix lint * Utilise changeset info * Counters * Use NoReceipts and ReadOnly * ReadOnly is incompatible with caching * Skip test leaking transactions * Fix block test * Change disable message for call-traces stage * Use block option for call traces integration * Fix retracing due to incarnation
111 lines
2.8 KiB
Go
111 lines
2.8 KiB
Go
package state
|
|
|
|
import (
|
|
"context"
|
|
"encoding/binary"
|
|
|
|
"github.com/VictoriaMetrics/fastcache"
|
|
"github.com/holiman/uint256"
|
|
|
|
"github.com/ledgerwatch/turbo-geth/common"
|
|
"github.com/ledgerwatch/turbo-geth/common/dbutils"
|
|
"github.com/ledgerwatch/turbo-geth/core/types/accounts"
|
|
)
|
|
|
|
var _ WriterWithChangeSets = (*CacheStateWriter)(nil)
|
|
|
|
type CacheStateWriter struct {
|
|
accountCache *fastcache.Cache
|
|
storageCache *fastcache.Cache
|
|
codeCache *fastcache.Cache
|
|
codeSizeCache *fastcache.Cache
|
|
}
|
|
|
|
func NewCacheStateWriter() *CacheStateWriter {
|
|
return &CacheStateWriter{}
|
|
}
|
|
|
|
func (w *CacheStateWriter) SetAccountCache(accountCache *fastcache.Cache) {
|
|
w.accountCache = accountCache
|
|
}
|
|
|
|
func (w *CacheStateWriter) SetStorageCache(storageCache *fastcache.Cache) {
|
|
w.storageCache = storageCache
|
|
}
|
|
|
|
func (w *CacheStateWriter) SetCodeCache(codeCache *fastcache.Cache) {
|
|
w.codeCache = codeCache
|
|
}
|
|
|
|
func (w *CacheStateWriter) SetCodeSizeCache(codeSizeCache *fastcache.Cache) {
|
|
w.codeSizeCache = codeSizeCache
|
|
}
|
|
|
|
func (w *CacheStateWriter) UpdateAccountData(ctx context.Context, address common.Address, original, account *accounts.Account) error {
|
|
value := make([]byte, account.EncodingLengthForStorage())
|
|
account.EncodeForStorage(value)
|
|
if w.accountCache != nil {
|
|
w.accountCache.Set(address[:], value)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (w *CacheStateWriter) UpdateAccountCode(address common.Address, incarnation uint64, codeHash common.Hash, code []byte) error {
|
|
if w.codeCache != nil {
|
|
if len(code) <= 1024 {
|
|
w.codeCache.Set(address[:], code)
|
|
} else {
|
|
w.codeCache.Del(address[:])
|
|
}
|
|
}
|
|
if w.codeSizeCache != nil {
|
|
var b [4]byte
|
|
binary.BigEndian.PutUint32(b[:], uint32(len(code)))
|
|
w.codeSizeCache.Set(address[:], b[:])
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (w *CacheStateWriter) DeleteAccount(ctx context.Context, address common.Address, original *accounts.Account) error {
|
|
if w.accountCache != nil {
|
|
w.accountCache.Set(address[:], nil)
|
|
}
|
|
if w.codeCache != nil {
|
|
w.codeCache.Set(address[:], nil)
|
|
}
|
|
if w.codeSizeCache != nil {
|
|
var b [4]byte
|
|
binary.BigEndian.PutUint32(b[:], 0)
|
|
w.codeSizeCache.Set(address[:], b[:])
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (w *CacheStateWriter) WriteAccountStorage(ctx context.Context, address common.Address, incarnation uint64, key *common.Hash, original, value *uint256.Int) error {
|
|
if *original == *value {
|
|
return nil
|
|
}
|
|
|
|
if w.storageCache != nil {
|
|
compositeKey := dbutils.PlainGenerateCompositeStorageKey(address, incarnation, *key)
|
|
w.storageCache.Set(compositeKey, value.Bytes())
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (w *CacheStateWriter) CreateContract(address common.Address) error {
|
|
return nil
|
|
}
|
|
|
|
func (w *CacheStateWriter) WriteChangeSets() error {
|
|
return nil
|
|
}
|
|
|
|
func (w *CacheStateWriter) WriteHistory() error {
|
|
return nil
|
|
}
|
|
|
|
func (w *CacheStateWriter) ChangeSetWriter() *ChangeSetWriter {
|
|
return nil
|
|
}
|