mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-25 04:57:17 +00:00
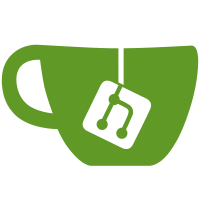
* make plain state + staged sync the default * remove sync mode flag completely * one thing * fix the console * simplify code * fix and skip tests * fixup for console tests
436 lines
14 KiB
Go
436 lines
14 KiB
Go
package commands
|
|
|
|
import (
|
|
"context"
|
|
"errors"
|
|
"fmt"
|
|
"math/big"
|
|
"strings"
|
|
|
|
"github.com/ledgerwatch/turbo-geth/common"
|
|
"github.com/ledgerwatch/turbo-geth/common/hexutil"
|
|
"github.com/ledgerwatch/turbo-geth/consensus"
|
|
"github.com/ledgerwatch/turbo-geth/core"
|
|
"github.com/ledgerwatch/turbo-geth/core/rawdb"
|
|
"github.com/ledgerwatch/turbo-geth/core/state"
|
|
"github.com/ledgerwatch/turbo-geth/core/types"
|
|
"github.com/ledgerwatch/turbo-geth/core/vm"
|
|
"github.com/ledgerwatch/turbo-geth/eth"
|
|
"github.com/ledgerwatch/turbo-geth/eth/stagedsync/stages"
|
|
"github.com/ledgerwatch/turbo-geth/ethdb"
|
|
"github.com/ledgerwatch/turbo-geth/internal/ethapi"
|
|
"github.com/ledgerwatch/turbo-geth/log"
|
|
"github.com/ledgerwatch/turbo-geth/node"
|
|
"github.com/ledgerwatch/turbo-geth/params"
|
|
"github.com/ledgerwatch/turbo-geth/rpc"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
// splitAndTrim splits input separated by a comma
|
|
// and trims excessive white space from the substrings.
|
|
func splitAndTrim(input string) []string {
|
|
result := strings.Split(input, ",")
|
|
for i, r := range result {
|
|
result[i] = strings.TrimSpace(r)
|
|
}
|
|
return result
|
|
}
|
|
|
|
// EthAPI is a collection of functions that are exposed in the
|
|
type EthAPI interface {
|
|
Coinbase(context.Context) (common.Address, error)
|
|
BlockNumber(ctx context.Context) (hexutil.Uint64, error)
|
|
GetBlockByNumber(ctx context.Context, number rpc.BlockNumber, fullTx bool) (map[string]interface{}, error)
|
|
GetBalance(_ context.Context, address common.Address, blockNrOrHash rpc.BlockNumberOrHash) (*hexutil.Big, error)
|
|
GetTransactionReceipt(ctx context.Context, hash common.Hash) (map[string]interface{}, error)
|
|
GetLogs(ctx context.Context, hash common.Hash) ([][]*types.Log, error)
|
|
}
|
|
|
|
// APIImpl is implementation of the EthAPI interface based on remote Db access
|
|
type APIImpl struct {
|
|
db ethdb.KV
|
|
dbReader ethdb.Getter
|
|
chainContext core.ChainContext
|
|
}
|
|
|
|
// PrivateDebugAPI
|
|
type PrivateDebugAPI interface {
|
|
StorageRangeAt(ctx context.Context, blockHash common.Hash, txIndex uint64, contractAddress common.Address, keyStart hexutil.Bytes, maxResult int) (StorageRangeResult, error)
|
|
TraceTransaction(ctx context.Context, hash common.Hash, config *eth.TraceConfig) (interface{}, error)
|
|
GetModifiedAccountsByNumber(ctx context.Context, startNum rpc.BlockNumber, endNum *rpc.BlockNumber) ([]common.Address, error)
|
|
GetModifiedAccountsByHash(_ context.Context, startHash common.Hash, endHash *common.Hash) ([]common.Address, error)
|
|
}
|
|
|
|
// APIImpl is implementation of the EthAPI interface based on remote Db access
|
|
type PrivateDebugAPIImpl struct {
|
|
db ethdb.KV
|
|
dbReader ethdb.Getter
|
|
chainContext core.ChainContext
|
|
}
|
|
|
|
// NewAPI returns APIImpl instance
|
|
func NewAPI(db ethdb.KV, dbReader ethdb.Getter, chainContext core.ChainContext) *APIImpl {
|
|
return &APIImpl{
|
|
db: db,
|
|
dbReader: dbReader,
|
|
chainContext: chainContext,
|
|
}
|
|
}
|
|
|
|
// NewPrivateDebugAPI returns PrivateDebugAPIImpl instance
|
|
func NewPrivateDebugAPI(db ethdb.KV, dbReader ethdb.Getter, chainContext core.ChainContext) *PrivateDebugAPIImpl {
|
|
return &PrivateDebugAPIImpl{
|
|
db: db,
|
|
dbReader: dbReader,
|
|
chainContext: chainContext,
|
|
}
|
|
}
|
|
|
|
func (api *APIImpl) BlockNumber(ctx context.Context) (hexutil.Uint64, error) {
|
|
execution, _, err := stages.GetStageProgress(api.dbReader, stages.Execution)
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
return hexutil.Uint64(execution), nil
|
|
}
|
|
|
|
// Etherbase is the address that mining rewards will be send to
|
|
func (api *APIImpl) Coinbase(_ context.Context) (common.Address, error) {
|
|
return common.Address{}, errors.New("not implemented")
|
|
}
|
|
|
|
func GetReceipts(ctx context.Context, db rawdb.DatabaseReader, cfg *params.ChainConfig, hash common.Hash) (types.Receipts, error) {
|
|
number := rawdb.ReadHeaderNumber(db, hash)
|
|
if number == nil {
|
|
return nil, fmt.Errorf("block not found: %x", hash)
|
|
}
|
|
|
|
block := rawdb.ReadBlock(db, hash, *number)
|
|
if cached := rawdb.ReadReceipts(db, block.Hash(), block.NumberU64(), cfg); cached != nil {
|
|
return cached, nil
|
|
}
|
|
|
|
bc := &blockGetter{db}
|
|
_, _, ibs, dbstate, err := ComputeTxEnv(ctx, bc, params.MainnetChainConfig, &chainContext{db: db}, db.(ethdb.HasKV).KV(), hash, 0, nil)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
var receipts types.Receipts
|
|
cc := &chainContext{db}
|
|
gp := new(core.GasPool).AddGas(block.GasLimit())
|
|
var usedGas = new(uint64)
|
|
for i, tx := range block.Transactions() {
|
|
ibs.Prepare(tx.Hash(), block.Hash(), i)
|
|
|
|
header := rawdb.ReadHeader(db, hash, *number)
|
|
receipt, err := core.ApplyTransaction(params.MainnetChainConfig, cc, nil, gp, ibs, dbstate, header, tx, usedGas, vm.Config{}, nil)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
receipts = append(receipts, receipt)
|
|
}
|
|
|
|
return receipts, nil
|
|
}
|
|
|
|
func (api *APIImpl) GetLogs(ctx context.Context, hash common.Hash) ([][]*types.Log, error) {
|
|
number := rawdb.ReadHeaderNumber(api.dbReader, hash)
|
|
if number == nil {
|
|
return nil, fmt.Errorf("block not found: %x", hash)
|
|
}
|
|
|
|
receipts, err := GetReceipts(ctx, api.dbReader, params.MainnetChainConfig, hash)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("getReceipt error: %v", err)
|
|
}
|
|
logs := make([][]*types.Log, len(receipts))
|
|
for i, receipt := range receipts {
|
|
logs[i] = receipt.Logs
|
|
}
|
|
return logs, nil
|
|
}
|
|
|
|
func (api *APIImpl) GetTransactionReceipt(ctx context.Context, hash common.Hash) (map[string]interface{}, error) {
|
|
// Retrieve the transaction and assemble its EVM context
|
|
tx, blockHash, blockNumber, txIndex := rawdb.ReadTransaction(api.dbReader, hash)
|
|
if tx == nil {
|
|
return nil, fmt.Errorf("transaction %#x not found", hash)
|
|
}
|
|
|
|
receipts, err := GetReceipts(ctx, api.dbReader, params.MainnetChainConfig, blockHash)
|
|
if err != nil {
|
|
return nil, fmt.Errorf("getReceipt error: %v", err)
|
|
}
|
|
if len(receipts) <= int(txIndex) {
|
|
return nil, fmt.Errorf("block has less receipts than expected: %d <= %d, block: %d", len(receipts), int(txIndex), blockNumber)
|
|
}
|
|
receipt := receipts[txIndex]
|
|
|
|
var signer types.Signer = types.FrontierSigner{}
|
|
if tx.Protected() {
|
|
signer = types.NewEIP155Signer(tx.ChainID().ToBig())
|
|
}
|
|
from, _ := types.Sender(signer, tx)
|
|
|
|
// Fill in the derived information in the logs
|
|
if receipt.Logs != nil {
|
|
for i, log := range receipt.Logs {
|
|
log.BlockNumber = blockNumber
|
|
log.TxHash = hash
|
|
log.TxIndex = uint(txIndex)
|
|
log.BlockHash = blockHash
|
|
log.Index = uint(i)
|
|
}
|
|
}
|
|
|
|
// Now reconstruct the bloom filter
|
|
fields := map[string]interface{}{
|
|
"blockHash": blockHash,
|
|
"blockNumber": hexutil.Uint64(blockNumber),
|
|
"transactionHash": hash,
|
|
"transactionIndex": hexutil.Uint64(txIndex),
|
|
"from": from,
|
|
"to": tx.To(),
|
|
"gasUsed": hexutil.Uint64(receipt.GasUsed),
|
|
"cumulativeGasUsed": hexutil.Uint64(receipt.CumulativeGasUsed),
|
|
"contractAddress": nil,
|
|
"logs": receipt.Logs,
|
|
"logsBloom": receipt.Bloom,
|
|
}
|
|
|
|
// Assign receipt status or post state.
|
|
if len(receipt.PostState) > 0 {
|
|
fields["root"] = hexutil.Bytes(receipt.PostState)
|
|
} else {
|
|
fields["status"] = hexutil.Uint(receipt.Status)
|
|
}
|
|
if receipt.Logs == nil {
|
|
fields["logs"] = [][]*types.Log{}
|
|
}
|
|
// If the ContractAddress is 20 0x0 bytes, assume it is not a contract creation
|
|
if receipt.ContractAddress != (common.Address{}) {
|
|
fields["contractAddress"] = receipt.ContractAddress
|
|
}
|
|
return fields, nil
|
|
}
|
|
|
|
type blockGetter struct {
|
|
dbReader rawdb.DatabaseReader
|
|
}
|
|
|
|
func (g *blockGetter) GetBlockByHash(hash common.Hash) *types.Block {
|
|
return rawdb.ReadBlockByHash(g.dbReader, hash)
|
|
|
|
}
|
|
|
|
func (g *blockGetter) GetBlock(hash common.Hash, number uint64) *types.Block {
|
|
return rawdb.ReadBlock(g.dbReader, hash, number)
|
|
}
|
|
|
|
type chainContext struct {
|
|
db rawdb.DatabaseReader
|
|
}
|
|
|
|
func NewChainContext(db rawdb.DatabaseReader) *chainContext {
|
|
return &chainContext{
|
|
db: db,
|
|
}
|
|
|
|
}
|
|
|
|
type powEngine struct {
|
|
}
|
|
|
|
func (c *powEngine) VerifyHeader(chain consensus.ChainReader, header *types.Header, seal bool) error {
|
|
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) VerifyHeaders(chain consensus.ChainReader, headers []*types.Header, seals []bool) (chan<- struct{}, <-chan error) {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) VerifyUncles(chain consensus.ChainReader, block *types.Block) error {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) VerifySeal(chain consensus.ChainReader, header *types.Header) error {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) Prepare(chain consensus.ChainReader, header *types.Header) error {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) Finalize(chainConfig *params.ChainConfig, header *types.Header, state *state.IntraBlockState, txs []*types.Transaction, uncles []*types.Header) {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) FinalizeAndAssemble(chainConfig *params.ChainConfig, header *types.Header, state *state.IntraBlockState, txs []*types.Transaction,
|
|
uncles []*types.Header, receipts []*types.Receipt) (*types.Block, error) {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) Seal(_ consensus.Cancel, chain consensus.ChainReader, block *types.Block, results chan<- consensus.ResultWithContext, stop <-chan struct{}) error {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) SealHash(header *types.Header) common.Hash {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) CalcDifficulty(chain consensus.ChainReader, time uint64, parent *types.Header) *big.Int {
|
|
panic("must not be called")
|
|
}
|
|
func (c *powEngine) APIs(chain consensus.ChainReader) []rpc.API {
|
|
panic("must not be called")
|
|
}
|
|
|
|
func (c *powEngine) Close() error {
|
|
panic("must not be called")
|
|
}
|
|
|
|
func (c *powEngine) Author(header *types.Header) (common.Address, error) {
|
|
return header.Coinbase, nil
|
|
}
|
|
|
|
func (c *chainContext) GetHeader(hash common.Hash, number uint64) *types.Header {
|
|
return rawdb.ReadHeader(c.db, hash, number)
|
|
}
|
|
|
|
func (c *chainContext) Engine() consensus.Engine {
|
|
return &powEngine{}
|
|
}
|
|
|
|
// GetBlockByNumber see https://github.com/ethereum/wiki/wiki/JSON-RPC#eth_getblockbynumber
|
|
// see internal/ethapi.PublicBlockChainAPI.GetBlockByNumber
|
|
func (api *APIImpl) GetBlockByNumber(ctx context.Context, number rpc.BlockNumber, fullTx bool) (map[string]interface{}, error) {
|
|
additionalFields := make(map[string]interface{})
|
|
|
|
block := rawdb.ReadBlockByNumber(api.dbReader, uint64(number.Int64()))
|
|
if block == nil {
|
|
return nil, fmt.Errorf("block not found: %d", number.Int64())
|
|
}
|
|
|
|
additionalFields["totalDifficulty"] = rawdb.ReadTd(api.dbReader, block.Hash(), uint64(number.Int64()))
|
|
response, err := api.rpcMarshalBlock(block, true, fullTx, additionalFields)
|
|
|
|
if err == nil && number == rpc.PendingBlockNumber {
|
|
// Pending blocks need to nil out a few fields
|
|
for _, field := range []string{"hash", "nonce", "miner"} {
|
|
response[field] = nil
|
|
}
|
|
}
|
|
return response, err
|
|
}
|
|
|
|
// StorageRangeAt re-implementation of eth/api.go:StorageRangeAt
|
|
func (api *PrivateDebugAPIImpl) StorageRangeAt(ctx context.Context, blockHash common.Hash, txIndex uint64, contractAddress common.Address, keyStart hexutil.Bytes, maxResult int) (StorageRangeResult, error) {
|
|
_, _, _, stateReader, err := ComputeTxEnv(ctx, &blockGetter{api.dbReader}, params.MainnetChainConfig, &chainContext{db: api.dbReader}, api.db, blockHash, txIndex, nil)
|
|
if err != nil {
|
|
return StorageRangeResult{}, err
|
|
}
|
|
return StorageRangeAt(stateReader, contractAddress, keyStart, maxResult)
|
|
}
|
|
|
|
// computeIntraBlockState retrieves the state database associated with a certain block.
|
|
// If no state is locally available for the given block, a number of blocks are
|
|
// attempted to be reexecuted to generate the desired state.
|
|
func (api *PrivateDebugAPIImpl) computeIntraBlockState(block *types.Block) (*state.IntraBlockState, *state.DbState) {
|
|
// If we have the state fully available, use that
|
|
dbstate := state.NewDbState(api.db, block.NumberU64())
|
|
statedb := state.New(dbstate)
|
|
return statedb, dbstate
|
|
}
|
|
|
|
// rpcMarshalBlock reimplementation of ethapi.rpcMarshalBlock
|
|
func (api *APIImpl) rpcMarshalBlock(b *types.Block, inclTx bool, fullTx bool, additional map[string]interface{}) (map[string]interface{}, error) {
|
|
fields, err := ethapi.RPCMarshalBlock(b, inclTx, fullTx)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
for k, v := range additional {
|
|
fields[k] = v
|
|
}
|
|
|
|
return fields, err
|
|
}
|
|
|
|
func GetAPI(db ethdb.KV, enabledApis []string) []rpc.API {
|
|
var rpcAPI = []rpc.API{}
|
|
|
|
dbReader := ethdb.NewObjectDatabase(db)
|
|
chainContext := NewChainContext(dbReader)
|
|
apiImpl := NewAPI(db, dbReader, chainContext)
|
|
dbgAPIImpl := NewPrivateDebugAPI(db, dbReader, chainContext)
|
|
|
|
for _, enabledAPI := range enabledApis {
|
|
switch enabledAPI {
|
|
case "eth":
|
|
rpcAPI = append(rpcAPI, rpc.API{
|
|
Namespace: "eth",
|
|
Public: true,
|
|
Service: EthAPI(apiImpl),
|
|
Version: "1.0",
|
|
})
|
|
case "debug":
|
|
rpcAPI = append(rpcAPI, rpc.API{
|
|
Namespace: "debug",
|
|
Public: true,
|
|
Service: PrivateDebugAPI(dbgAPIImpl),
|
|
Version: "1.0",
|
|
})
|
|
|
|
default:
|
|
log.Error("Unrecognised", "api", enabledAPI)
|
|
}
|
|
}
|
|
return rpcAPI
|
|
}
|
|
|
|
func daemon(cmd *cobra.Command, cfg Config) {
|
|
vhosts := splitAndTrim(cfg.rpcVirtualHost)
|
|
cors := splitAndTrim(cfg.rpcCORSDomain)
|
|
enabledApis := splitAndTrim(cfg.rpcAPI)
|
|
|
|
var db ethdb.KV
|
|
var err error
|
|
if cfg.remoteDbAddress != "" {
|
|
db, err = ethdb.NewRemote().Path(cfg.remoteDbAddress).Open()
|
|
} else if cfg.chaindata != "" {
|
|
if database, errOpen := ethdb.Open(cfg.chaindata); errOpen == nil {
|
|
db = database.KV()
|
|
} else {
|
|
err = errOpen
|
|
}
|
|
} else {
|
|
err = fmt.Errorf("either remote db or bolt db must be specified")
|
|
}
|
|
if err != nil {
|
|
log.Error("Could not connect to remoteDb", "error", err)
|
|
return
|
|
}
|
|
|
|
var rpcAPI = GetAPI(db, enabledApis)
|
|
|
|
httpEndpoint := fmt.Sprintf("%s:%d", cfg.rpcListenAddress, cfg.rpcPort)
|
|
|
|
// register apis and create handler stack
|
|
srv := rpc.NewServer()
|
|
err = node.RegisterApisFromWhitelist(rpcAPI, enabledApis, srv, false)
|
|
if err != nil {
|
|
log.Error("Could not start register RPC apis", "error", err)
|
|
return
|
|
}
|
|
handler := node.NewHTTPHandlerStack(srv, cors, vhosts)
|
|
|
|
listener, _, err := node.StartHTTPEndpoint(httpEndpoint, rpc.DefaultHTTPTimeouts, handler)
|
|
if err != nil {
|
|
log.Error("Could not start RPC api", "error", err)
|
|
return
|
|
}
|
|
extapiURL := fmt.Sprintf("http://%s", httpEndpoint)
|
|
log.Info("HTTP endpoint opened", "url", extapiURL)
|
|
|
|
defer func() {
|
|
listener.Close()
|
|
log.Info("HTTP endpoint closed", "url", httpEndpoint)
|
|
}()
|
|
|
|
sig := <-cmd.Context().Done()
|
|
log.Info("Exiting...", "signal", sig)
|
|
}
|