mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-25 21:17:16 +00:00
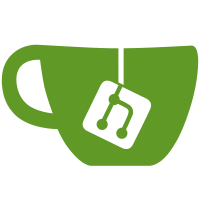
* #remove debug prints * remove storage-mode="i" * minnet re-execute hack with checkpoints * minnet re-execute hack with checkpoints * rollback to master setup * mainnet re-exec hack * rollback some changes * v0 of "push down" functionality * move all logic to own functions * handle case when re-created account already has some storage * clear path for storage * try to rely on tree structure (but maybe need to rely on DB because can be intra-block re-creations of account) * fix some bugs with indexes, moving to tests * tests added * make linter happy * make linter happy * simplify logic * adjust comparison of keys with and without incarnation * test for keyIsBefore * test for keyIsBefore * better nibbles alignment * better nibbles alignment * cleanup * continue work on tests * simplify test * check tombstone existence before pushing it down. * put tombstone only when account deleted, not created * put tombstone only when account has storage * make linter happy * test for storage resolver * make fixedbytes work without incarnation * fix panic on short keys * use special comparison only when working with keys from cache * add blockNr for better tracing * fix: incorrect tombstone check * fix: incorrect tombstone check * trigger ci * hack for problem block * more test-cases * add test case for too long keys * speedup cached resolver by removing bucket creation transaction * remove parent type check in pruning, remove unused copy from mutation.put * dump resolving info on fail * dump resolving info on fail * set tombstone everytime for now to check if it will help * on unload: check parent type, not type of node * fix wrong order of checking node type * fix wrong order of checking node type * rebase to new master * make linter happy * rebase to new master * place tombstone only if acc has storage * rebase master * rebase master * rebase master * rebase master Co-authored-by: alex.sharov <alex.sharov@lazada.com>
27 lines
655 B
Go
27 lines
655 B
Go
package trie
|
|
|
|
// CompressNibbles - supports only even number of nibbles
|
|
// This method supports only arrays of even nibbles
|
|
//
|
|
// HI_NIBBLE(b) = (b >> 4) & 0x0F
|
|
// LO_NIBBLE(b) = b & 0x0F
|
|
func CompressNibbles(nibbles []byte, out *[]byte) {
|
|
tmp := (*out)[:0]
|
|
for i := 0; i < len(nibbles); i += 2 {
|
|
tmp = append(tmp, nibbles[i]<<4|nibbles[i+1])
|
|
}
|
|
*out = tmp
|
|
}
|
|
|
|
// DecompressNibbles - supports only even number of nibbles
|
|
//
|
|
// HI_NIBBLE(b) = (b >> 4) & 0x0F
|
|
// LO_NIBBLE(b) = b & 0x0F
|
|
func DecompressNibbles(in []byte, out *[]byte) {
|
|
tmp := (*out)[:0]
|
|
for i := 0; i < len(in); i++ {
|
|
tmp = append(tmp, (in[i]>>4)&0x0F, in[i]&0x0F)
|
|
}
|
|
*out = tmp
|
|
}
|