mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2025-01-01 00:31:21 +00:00
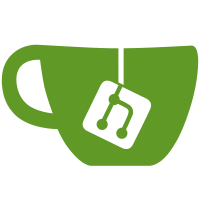
* Domain * First functions * change year * More on domain * More to test * More on test * More on domains * buildFiles * More on domains * Collation test * Fix collate * Add test for decompressors * Restructure history tables * Split history into 2 tables * Fix lint * Check index files in the test * Close files * Add file scanning * Fix lint * Fix lint * Add readFromFiles * Add ef history idx file * Start cleanup * More to cleanup, test for ef history * More test * Add prune to test * Test for prune and fix * Start history access * History test * Test for LastDup * Fix one lint * Workaround * History tests * Debug * Fix * Fix in history * Fix lint Co-authored-by: Alexey Sharp <alexeysharp@Alexeys-iMac.local> Co-authored-by: Alex Sharp <alexsharp@alexs-macbook-pro.home> Co-authored-by: Alex Sharp <alexsharp@Alexs-MacBook-Pro.local> Co-authored-by: Alex Sharp <alexsharp@alexs-mbp.lan>
110 lines
3.1 KiB
Go
110 lines
3.1 KiB
Go
/*
|
|
Copyright 2022 Erigon contributors
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
*/
|
|
|
|
package mdbx
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
|
|
"github.com/ledgerwatch/erigon-lib/kv"
|
|
"github.com/ledgerwatch/log/v3"
|
|
"github.com/stretchr/testify/require"
|
|
)
|
|
|
|
func TestSeekBothRange(t *testing.T) {
|
|
path := t.TempDir()
|
|
logger := log.New()
|
|
table := "Table"
|
|
db := NewMDBX(logger).Path(path).WithTablessCfg(func(defaultBuckets kv.TableCfg) kv.TableCfg {
|
|
return kv.TableCfg{
|
|
table: kv.TableCfgItem{Flags: kv.DupSort},
|
|
}
|
|
}).MustOpen()
|
|
defer db.Close()
|
|
|
|
tx, err := db.BeginRw(context.Background())
|
|
require.NoError(t, err)
|
|
defer tx.Rollback()
|
|
|
|
c, err := tx.RwCursorDupSort(table)
|
|
require.NoError(t, err)
|
|
defer c.Close()
|
|
|
|
// Insert some dupsorted records
|
|
require.NoError(t, c.Put([]byte("key1"), []byte("value1.1")))
|
|
require.NoError(t, c.Put([]byte("key3"), []byte("value3.1")))
|
|
require.NoError(t, c.Put([]byte("key1"), []byte("value1.3")))
|
|
require.NoError(t, c.Put([]byte("key3"), []byte("value3.3")))
|
|
|
|
v, err := c.SeekBothRange([]byte("key2"), []byte("value1.2"))
|
|
require.NoError(t, err)
|
|
// SeekBothRange does extact match of the key, but range match of the value, so we get nil here
|
|
require.Nil(t, v)
|
|
|
|
v, err = c.SeekBothRange([]byte("key3"), []byte("value3.2"))
|
|
require.NoError(t, err)
|
|
require.Equal(t, "value3.3", string(v))
|
|
}
|
|
|
|
func TestLastDup(t *testing.T) {
|
|
path := t.TempDir()
|
|
logger := log.New()
|
|
table := "Table"
|
|
db := NewMDBX(logger).Path(path).WithTablessCfg(func(defaultBuckets kv.TableCfg) kv.TableCfg {
|
|
return kv.TableCfg{
|
|
table: kv.TableCfgItem{Flags: kv.DupSort},
|
|
}
|
|
}).MustOpen()
|
|
defer db.Close()
|
|
|
|
tx, err := db.BeginRw(context.Background())
|
|
require.NoError(t, err)
|
|
defer tx.Rollback()
|
|
|
|
c, err := tx.RwCursorDupSort(table)
|
|
require.NoError(t, err)
|
|
defer c.Close()
|
|
|
|
// Insert some dupsorted records
|
|
require.NoError(t, c.Put([]byte("key1"), []byte("value1.1")))
|
|
require.NoError(t, c.Put([]byte("key3"), []byte("value3.1")))
|
|
require.NoError(t, c.Put([]byte("key1"), []byte("value1.3")))
|
|
require.NoError(t, c.Put([]byte("key3"), []byte("value3.3")))
|
|
|
|
err = tx.Commit()
|
|
require.NoError(t, err)
|
|
roTx, err := db.BeginRo(context.Background())
|
|
require.NoError(t, err)
|
|
defer roTx.Rollback()
|
|
|
|
roC, err := roTx.CursorDupSort(table)
|
|
require.NoError(t, err)
|
|
defer roC.Close()
|
|
|
|
var keys, vals []string
|
|
var k, v []byte
|
|
for k, _, err = roC.First(); err == nil && k != nil; k, _, err = roC.NextNoDup() {
|
|
v, err = roC.LastDup()
|
|
require.NoError(t, err)
|
|
keys = append(keys, string(k))
|
|
vals = append(vals, string(v))
|
|
}
|
|
require.NoError(t, err)
|
|
require.Equal(t, []string{"key1", "key3"}, keys)
|
|
require.Equal(t, []string{"value1.3", "value3.3"}, vals)
|
|
}
|