mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-28 14:47:16 +00:00
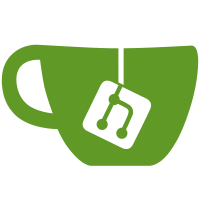
* Remove interfaces for replacement * Squashed 'interfaces/' content from commit a25a685 git-subtree-dir: interfaces git-subtree-split: a25a68528428cc6d792b5033dd97d094c14e1dd9 * Regenerate proto * eth/66 -> eth/67 * Auto-generated leftovers * Remove interfaces for replacement * Squashed 'interfaces/' content from commit 674172f git-subtree-dir: interfaces git-subtree-split: 674172f20ef9a77bcff1c97213f5f3865115f213 * Refresh generated proto * Remove version suffix from MessageId
2233 lines
77 KiB
Go
2233 lines
77 KiB
Go
// Code generated by protoc-gen-go. DO NOT EDIT.
|
|
// versions:
|
|
// protoc-gen-go v1.28.0
|
|
// protoc v3.20.1
|
|
// source: remote/ethbackend.proto
|
|
|
|
package remote
|
|
|
|
import (
|
|
types "github.com/ledgerwatch/erigon-lib/gointerfaces/types"
|
|
protoreflect "google.golang.org/protobuf/reflect/protoreflect"
|
|
protoimpl "google.golang.org/protobuf/runtime/protoimpl"
|
|
emptypb "google.golang.org/protobuf/types/known/emptypb"
|
|
reflect "reflect"
|
|
sync "sync"
|
|
)
|
|
|
|
const (
|
|
// Verify that this generated code is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(20 - protoimpl.MinVersion)
|
|
// Verify that runtime/protoimpl is sufficiently up-to-date.
|
|
_ = protoimpl.EnforceVersion(protoimpl.MaxVersion - 20)
|
|
)
|
|
|
|
type Event int32
|
|
|
|
const (
|
|
Event_HEADER Event = 0
|
|
Event_PENDING_LOGS Event = 1
|
|
Event_PENDING_BLOCK Event = 2
|
|
// NEW_SNAPSHOT - one or many new snapshots (of snapshot sync) were created,
|
|
// client need to close old file descriptors and open new (on new segments),
|
|
// then server can remove old files
|
|
Event_NEW_SNAPSHOT Event = 3
|
|
)
|
|
|
|
// Enum value maps for Event.
|
|
var (
|
|
Event_name = map[int32]string{
|
|
0: "HEADER",
|
|
1: "PENDING_LOGS",
|
|
2: "PENDING_BLOCK",
|
|
3: "NEW_SNAPSHOT",
|
|
}
|
|
Event_value = map[string]int32{
|
|
"HEADER": 0,
|
|
"PENDING_LOGS": 1,
|
|
"PENDING_BLOCK": 2,
|
|
"NEW_SNAPSHOT": 3,
|
|
}
|
|
)
|
|
|
|
func (x Event) Enum() *Event {
|
|
p := new(Event)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x Event) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (Event) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_remote_ethbackend_proto_enumTypes[0].Descriptor()
|
|
}
|
|
|
|
func (Event) Type() protoreflect.EnumType {
|
|
return &file_remote_ethbackend_proto_enumTypes[0]
|
|
}
|
|
|
|
func (x Event) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use Event.Descriptor instead.
|
|
func (Event) EnumDescriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
type EngineStatus int32
|
|
|
|
const (
|
|
EngineStatus_VALID EngineStatus = 0
|
|
EngineStatus_INVALID EngineStatus = 1
|
|
EngineStatus_SYNCING EngineStatus = 2
|
|
EngineStatus_ACCEPTED EngineStatus = 3
|
|
EngineStatus_INVALID_BLOCK_HASH EngineStatus = 4
|
|
)
|
|
|
|
// Enum value maps for EngineStatus.
|
|
var (
|
|
EngineStatus_name = map[int32]string{
|
|
0: "VALID",
|
|
1: "INVALID",
|
|
2: "SYNCING",
|
|
3: "ACCEPTED",
|
|
4: "INVALID_BLOCK_HASH",
|
|
}
|
|
EngineStatus_value = map[string]int32{
|
|
"VALID": 0,
|
|
"INVALID": 1,
|
|
"SYNCING": 2,
|
|
"ACCEPTED": 3,
|
|
"INVALID_BLOCK_HASH": 4,
|
|
}
|
|
)
|
|
|
|
func (x EngineStatus) Enum() *EngineStatus {
|
|
p := new(EngineStatus)
|
|
*p = x
|
|
return p
|
|
}
|
|
|
|
func (x EngineStatus) String() string {
|
|
return protoimpl.X.EnumStringOf(x.Descriptor(), protoreflect.EnumNumber(x))
|
|
}
|
|
|
|
func (EngineStatus) Descriptor() protoreflect.EnumDescriptor {
|
|
return file_remote_ethbackend_proto_enumTypes[1].Descriptor()
|
|
}
|
|
|
|
func (EngineStatus) Type() protoreflect.EnumType {
|
|
return &file_remote_ethbackend_proto_enumTypes[1]
|
|
}
|
|
|
|
func (x EngineStatus) Number() protoreflect.EnumNumber {
|
|
return protoreflect.EnumNumber(x)
|
|
}
|
|
|
|
// Deprecated: Use EngineStatus.Descriptor instead.
|
|
func (EngineStatus) EnumDescriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
type EtherbaseRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *EtherbaseRequest) Reset() {
|
|
*x = EtherbaseRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[0]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *EtherbaseRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*EtherbaseRequest) ProtoMessage() {}
|
|
|
|
func (x *EtherbaseRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[0]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use EtherbaseRequest.ProtoReflect.Descriptor instead.
|
|
func (*EtherbaseRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{0}
|
|
}
|
|
|
|
type EtherbaseReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Address *types.H160 `protobuf:"bytes,1,opt,name=address,proto3" json:"address,omitempty"`
|
|
}
|
|
|
|
func (x *EtherbaseReply) Reset() {
|
|
*x = EtherbaseReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[1]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *EtherbaseReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*EtherbaseReply) ProtoMessage() {}
|
|
|
|
func (x *EtherbaseReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[1]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use EtherbaseReply.ProtoReflect.Descriptor instead.
|
|
func (*EtherbaseReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{1}
|
|
}
|
|
|
|
func (x *EtherbaseReply) GetAddress() *types.H160 {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type NetVersionRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *NetVersionRequest) Reset() {
|
|
*x = NetVersionRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[2]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *NetVersionRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NetVersionRequest) ProtoMessage() {}
|
|
|
|
func (x *NetVersionRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[2]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NetVersionRequest.ProtoReflect.Descriptor instead.
|
|
func (*NetVersionRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{2}
|
|
}
|
|
|
|
type NetVersionReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id uint64 `protobuf:"varint,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
}
|
|
|
|
func (x *NetVersionReply) Reset() {
|
|
*x = NetVersionReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[3]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *NetVersionReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NetVersionReply) ProtoMessage() {}
|
|
|
|
func (x *NetVersionReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[3]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NetVersionReply.ProtoReflect.Descriptor instead.
|
|
func (*NetVersionReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{3}
|
|
}
|
|
|
|
func (x *NetVersionReply) GetId() uint64 {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type NetPeerCountRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *NetPeerCountRequest) Reset() {
|
|
*x = NetPeerCountRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[4]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *NetPeerCountRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NetPeerCountRequest) ProtoMessage() {}
|
|
|
|
func (x *NetPeerCountRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[4]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NetPeerCountRequest.ProtoReflect.Descriptor instead.
|
|
func (*NetPeerCountRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{4}
|
|
}
|
|
|
|
type NetPeerCountReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Count uint64 `protobuf:"varint,1,opt,name=count,proto3" json:"count,omitempty"`
|
|
}
|
|
|
|
func (x *NetPeerCountReply) Reset() {
|
|
*x = NetPeerCountReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[5]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *NetPeerCountReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NetPeerCountReply) ProtoMessage() {}
|
|
|
|
func (x *NetPeerCountReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[5]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NetPeerCountReply.ProtoReflect.Descriptor instead.
|
|
func (*NetPeerCountReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{5}
|
|
}
|
|
|
|
func (x *NetPeerCountReply) GetCount() uint64 {
|
|
if x != nil {
|
|
return x.Count
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type EngineGetPayloadRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
PayloadId uint64 `protobuf:"varint,1,opt,name=payloadId,proto3" json:"payloadId,omitempty"`
|
|
}
|
|
|
|
func (x *EngineGetPayloadRequest) Reset() {
|
|
*x = EngineGetPayloadRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[6]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *EngineGetPayloadRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*EngineGetPayloadRequest) ProtoMessage() {}
|
|
|
|
func (x *EngineGetPayloadRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[6]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use EngineGetPayloadRequest.ProtoReflect.Descriptor instead.
|
|
func (*EngineGetPayloadRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{6}
|
|
}
|
|
|
|
func (x *EngineGetPayloadRequest) GetPayloadId() uint64 {
|
|
if x != nil {
|
|
return x.PayloadId
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type EnginePayloadStatus struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Status EngineStatus `protobuf:"varint,1,opt,name=status,proto3,enum=remote.EngineStatus" json:"status,omitempty"`
|
|
LatestValidHash *types.H256 `protobuf:"bytes,2,opt,name=latestValidHash,proto3" json:"latestValidHash,omitempty"`
|
|
ValidationError string `protobuf:"bytes,3,opt,name=validationError,proto3" json:"validationError,omitempty"`
|
|
}
|
|
|
|
func (x *EnginePayloadStatus) Reset() {
|
|
*x = EnginePayloadStatus{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[7]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *EnginePayloadStatus) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*EnginePayloadStatus) ProtoMessage() {}
|
|
|
|
func (x *EnginePayloadStatus) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[7]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use EnginePayloadStatus.ProtoReflect.Descriptor instead.
|
|
func (*EnginePayloadStatus) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{7}
|
|
}
|
|
|
|
func (x *EnginePayloadStatus) GetStatus() EngineStatus {
|
|
if x != nil {
|
|
return x.Status
|
|
}
|
|
return EngineStatus_VALID
|
|
}
|
|
|
|
func (x *EnginePayloadStatus) GetLatestValidHash() *types.H256 {
|
|
if x != nil {
|
|
return x.LatestValidHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *EnginePayloadStatus) GetValidationError() string {
|
|
if x != nil {
|
|
return x.ValidationError
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type EnginePayloadAttributes struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Timestamp uint64 `protobuf:"varint,1,opt,name=timestamp,proto3" json:"timestamp,omitempty"`
|
|
PrevRandao *types.H256 `protobuf:"bytes,2,opt,name=prevRandao,proto3" json:"prevRandao,omitempty"`
|
|
SuggestedFeeRecipient *types.H160 `protobuf:"bytes,3,opt,name=suggestedFeeRecipient,proto3" json:"suggestedFeeRecipient,omitempty"`
|
|
}
|
|
|
|
func (x *EnginePayloadAttributes) Reset() {
|
|
*x = EnginePayloadAttributes{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[8]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *EnginePayloadAttributes) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*EnginePayloadAttributes) ProtoMessage() {}
|
|
|
|
func (x *EnginePayloadAttributes) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[8]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use EnginePayloadAttributes.ProtoReflect.Descriptor instead.
|
|
func (*EnginePayloadAttributes) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{8}
|
|
}
|
|
|
|
func (x *EnginePayloadAttributes) GetTimestamp() uint64 {
|
|
if x != nil {
|
|
return x.Timestamp
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *EnginePayloadAttributes) GetPrevRandao() *types.H256 {
|
|
if x != nil {
|
|
return x.PrevRandao
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *EnginePayloadAttributes) GetSuggestedFeeRecipient() *types.H160 {
|
|
if x != nil {
|
|
return x.SuggestedFeeRecipient
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type EngineForkChoiceState struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
HeadBlockHash *types.H256 `protobuf:"bytes,1,opt,name=headBlockHash,proto3" json:"headBlockHash,omitempty"`
|
|
SafeBlockHash *types.H256 `protobuf:"bytes,2,opt,name=safeBlockHash,proto3" json:"safeBlockHash,omitempty"`
|
|
FinalizedBlockHash *types.H256 `protobuf:"bytes,3,opt,name=finalizedBlockHash,proto3" json:"finalizedBlockHash,omitempty"`
|
|
}
|
|
|
|
func (x *EngineForkChoiceState) Reset() {
|
|
*x = EngineForkChoiceState{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[9]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *EngineForkChoiceState) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*EngineForkChoiceState) ProtoMessage() {}
|
|
|
|
func (x *EngineForkChoiceState) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[9]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use EngineForkChoiceState.ProtoReflect.Descriptor instead.
|
|
func (*EngineForkChoiceState) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{9}
|
|
}
|
|
|
|
func (x *EngineForkChoiceState) GetHeadBlockHash() *types.H256 {
|
|
if x != nil {
|
|
return x.HeadBlockHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *EngineForkChoiceState) GetSafeBlockHash() *types.H256 {
|
|
if x != nil {
|
|
return x.SafeBlockHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *EngineForkChoiceState) GetFinalizedBlockHash() *types.H256 {
|
|
if x != nil {
|
|
return x.FinalizedBlockHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type EngineForkChoiceUpdatedRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
ForkchoiceState *EngineForkChoiceState `protobuf:"bytes,1,opt,name=forkchoiceState,proto3" json:"forkchoiceState,omitempty"`
|
|
PayloadAttributes *EnginePayloadAttributes `protobuf:"bytes,2,opt,name=payloadAttributes,proto3" json:"payloadAttributes,omitempty"`
|
|
}
|
|
|
|
func (x *EngineForkChoiceUpdatedRequest) Reset() {
|
|
*x = EngineForkChoiceUpdatedRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[10]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *EngineForkChoiceUpdatedRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*EngineForkChoiceUpdatedRequest) ProtoMessage() {}
|
|
|
|
func (x *EngineForkChoiceUpdatedRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[10]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use EngineForkChoiceUpdatedRequest.ProtoReflect.Descriptor instead.
|
|
func (*EngineForkChoiceUpdatedRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{10}
|
|
}
|
|
|
|
func (x *EngineForkChoiceUpdatedRequest) GetForkchoiceState() *EngineForkChoiceState {
|
|
if x != nil {
|
|
return x.ForkchoiceState
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *EngineForkChoiceUpdatedRequest) GetPayloadAttributes() *EnginePayloadAttributes {
|
|
if x != nil {
|
|
return x.PayloadAttributes
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type EngineForkChoiceUpdatedReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
PayloadStatus *EnginePayloadStatus `protobuf:"bytes,1,opt,name=payloadStatus,proto3" json:"payloadStatus,omitempty"`
|
|
PayloadId uint64 `protobuf:"varint,2,opt,name=payloadId,proto3" json:"payloadId,omitempty"`
|
|
}
|
|
|
|
func (x *EngineForkChoiceUpdatedReply) Reset() {
|
|
*x = EngineForkChoiceUpdatedReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[11]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *EngineForkChoiceUpdatedReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*EngineForkChoiceUpdatedReply) ProtoMessage() {}
|
|
|
|
func (x *EngineForkChoiceUpdatedReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[11]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use EngineForkChoiceUpdatedReply.ProtoReflect.Descriptor instead.
|
|
func (*EngineForkChoiceUpdatedReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{11}
|
|
}
|
|
|
|
func (x *EngineForkChoiceUpdatedReply) GetPayloadStatus() *EnginePayloadStatus {
|
|
if x != nil {
|
|
return x.PayloadStatus
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *EngineForkChoiceUpdatedReply) GetPayloadId() uint64 {
|
|
if x != nil {
|
|
return x.PayloadId
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type ProtocolVersionRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *ProtocolVersionRequest) Reset() {
|
|
*x = ProtocolVersionRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[12]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ProtocolVersionRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ProtocolVersionRequest) ProtoMessage() {}
|
|
|
|
func (x *ProtocolVersionRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[12]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ProtocolVersionRequest.ProtoReflect.Descriptor instead.
|
|
func (*ProtocolVersionRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{12}
|
|
}
|
|
|
|
type ProtocolVersionReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Id uint64 `protobuf:"varint,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
}
|
|
|
|
func (x *ProtocolVersionReply) Reset() {
|
|
*x = ProtocolVersionReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[13]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ProtocolVersionReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ProtocolVersionReply) ProtoMessage() {}
|
|
|
|
func (x *ProtocolVersionReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[13]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ProtocolVersionReply.ProtoReflect.Descriptor instead.
|
|
func (*ProtocolVersionReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{13}
|
|
}
|
|
|
|
func (x *ProtocolVersionReply) GetId() uint64 {
|
|
if x != nil {
|
|
return x.Id
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type ClientVersionRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
}
|
|
|
|
func (x *ClientVersionRequest) Reset() {
|
|
*x = ClientVersionRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[14]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ClientVersionRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ClientVersionRequest) ProtoMessage() {}
|
|
|
|
func (x *ClientVersionRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[14]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ClientVersionRequest.ProtoReflect.Descriptor instead.
|
|
func (*ClientVersionRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{14}
|
|
}
|
|
|
|
type ClientVersionReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
NodeName string `protobuf:"bytes,1,opt,name=nodeName,proto3" json:"nodeName,omitempty"`
|
|
}
|
|
|
|
func (x *ClientVersionReply) Reset() {
|
|
*x = ClientVersionReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[15]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *ClientVersionReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*ClientVersionReply) ProtoMessage() {}
|
|
|
|
func (x *ClientVersionReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[15]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use ClientVersionReply.ProtoReflect.Descriptor instead.
|
|
func (*ClientVersionReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{15}
|
|
}
|
|
|
|
func (x *ClientVersionReply) GetNodeName() string {
|
|
if x != nil {
|
|
return x.NodeName
|
|
}
|
|
return ""
|
|
}
|
|
|
|
type SubscribeRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Type Event `protobuf:"varint,1,opt,name=type,proto3,enum=remote.Event" json:"type,omitempty"`
|
|
}
|
|
|
|
func (x *SubscribeRequest) Reset() {
|
|
*x = SubscribeRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[16]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *SubscribeRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SubscribeRequest) ProtoMessage() {}
|
|
|
|
func (x *SubscribeRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[16]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SubscribeRequest.ProtoReflect.Descriptor instead.
|
|
func (*SubscribeRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{16}
|
|
}
|
|
|
|
func (x *SubscribeRequest) GetType() Event {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return Event_HEADER
|
|
}
|
|
|
|
type SubscribeReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Type Event `protobuf:"varint,1,opt,name=type,proto3,enum=remote.Event" json:"type,omitempty"`
|
|
Data []byte `protobuf:"bytes,2,opt,name=data,proto3" json:"data,omitempty"` // serialized data
|
|
}
|
|
|
|
func (x *SubscribeReply) Reset() {
|
|
*x = SubscribeReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[17]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *SubscribeReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SubscribeReply) ProtoMessage() {}
|
|
|
|
func (x *SubscribeReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[17]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SubscribeReply.ProtoReflect.Descriptor instead.
|
|
func (*SubscribeReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{17}
|
|
}
|
|
|
|
func (x *SubscribeReply) GetType() Event {
|
|
if x != nil {
|
|
return x.Type
|
|
}
|
|
return Event_HEADER
|
|
}
|
|
|
|
func (x *SubscribeReply) GetData() []byte {
|
|
if x != nil {
|
|
return x.Data
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type LogsFilterRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
AllAddresses bool `protobuf:"varint,1,opt,name=allAddresses,proto3" json:"allAddresses,omitempty"`
|
|
Addresses []*types.H160 `protobuf:"bytes,2,rep,name=addresses,proto3" json:"addresses,omitempty"`
|
|
AllTopics bool `protobuf:"varint,3,opt,name=allTopics,proto3" json:"allTopics,omitempty"`
|
|
Topics []*types.H256 `protobuf:"bytes,4,rep,name=topics,proto3" json:"topics,omitempty"`
|
|
}
|
|
|
|
func (x *LogsFilterRequest) Reset() {
|
|
*x = LogsFilterRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[18]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *LogsFilterRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*LogsFilterRequest) ProtoMessage() {}
|
|
|
|
func (x *LogsFilterRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[18]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use LogsFilterRequest.ProtoReflect.Descriptor instead.
|
|
func (*LogsFilterRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{18}
|
|
}
|
|
|
|
func (x *LogsFilterRequest) GetAllAddresses() bool {
|
|
if x != nil {
|
|
return x.AllAddresses
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *LogsFilterRequest) GetAddresses() []*types.H160 {
|
|
if x != nil {
|
|
return x.Addresses
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *LogsFilterRequest) GetAllTopics() bool {
|
|
if x != nil {
|
|
return x.AllTopics
|
|
}
|
|
return false
|
|
}
|
|
|
|
func (x *LogsFilterRequest) GetTopics() []*types.H256 {
|
|
if x != nil {
|
|
return x.Topics
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type SubscribeLogsReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Address *types.H160 `protobuf:"bytes,1,opt,name=address,proto3" json:"address,omitempty"`
|
|
BlockHash *types.H256 `protobuf:"bytes,2,opt,name=blockHash,proto3" json:"blockHash,omitempty"`
|
|
BlockNumber uint64 `protobuf:"varint,3,opt,name=blockNumber,proto3" json:"blockNumber,omitempty"`
|
|
Data []byte `protobuf:"bytes,4,opt,name=data,proto3" json:"data,omitempty"`
|
|
LogIndex uint64 `protobuf:"varint,5,opt,name=logIndex,proto3" json:"logIndex,omitempty"`
|
|
Topics []*types.H256 `protobuf:"bytes,6,rep,name=topics,proto3" json:"topics,omitempty"`
|
|
TransactionHash *types.H256 `protobuf:"bytes,7,opt,name=transactionHash,proto3" json:"transactionHash,omitempty"`
|
|
TransactionIndex uint64 `protobuf:"varint,8,opt,name=transactionIndex,proto3" json:"transactionIndex,omitempty"`
|
|
Removed bool `protobuf:"varint,9,opt,name=removed,proto3" json:"removed,omitempty"`
|
|
}
|
|
|
|
func (x *SubscribeLogsReply) Reset() {
|
|
*x = SubscribeLogsReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[19]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *SubscribeLogsReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*SubscribeLogsReply) ProtoMessage() {}
|
|
|
|
func (x *SubscribeLogsReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[19]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use SubscribeLogsReply.ProtoReflect.Descriptor instead.
|
|
func (*SubscribeLogsReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{19}
|
|
}
|
|
|
|
func (x *SubscribeLogsReply) GetAddress() *types.H160 {
|
|
if x != nil {
|
|
return x.Address
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SubscribeLogsReply) GetBlockHash() *types.H256 {
|
|
if x != nil {
|
|
return x.BlockHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SubscribeLogsReply) GetBlockNumber() uint64 {
|
|
if x != nil {
|
|
return x.BlockNumber
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *SubscribeLogsReply) GetData() []byte {
|
|
if x != nil {
|
|
return x.Data
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SubscribeLogsReply) GetLogIndex() uint64 {
|
|
if x != nil {
|
|
return x.LogIndex
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *SubscribeLogsReply) GetTopics() []*types.H256 {
|
|
if x != nil {
|
|
return x.Topics
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SubscribeLogsReply) GetTransactionHash() *types.H256 {
|
|
if x != nil {
|
|
return x.TransactionHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *SubscribeLogsReply) GetTransactionIndex() uint64 {
|
|
if x != nil {
|
|
return x.TransactionIndex
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *SubscribeLogsReply) GetRemoved() bool {
|
|
if x != nil {
|
|
return x.Removed
|
|
}
|
|
return false
|
|
}
|
|
|
|
type BlockRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
BlockHeight uint64 `protobuf:"varint,2,opt,name=blockHeight,proto3" json:"blockHeight,omitempty"`
|
|
BlockHash *types.H256 `protobuf:"bytes,3,opt,name=blockHash,proto3" json:"blockHash,omitempty"`
|
|
}
|
|
|
|
func (x *BlockRequest) Reset() {
|
|
*x = BlockRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[20]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BlockRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BlockRequest) ProtoMessage() {}
|
|
|
|
func (x *BlockRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[20]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BlockRequest.ProtoReflect.Descriptor instead.
|
|
func (*BlockRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{20}
|
|
}
|
|
|
|
func (x *BlockRequest) GetBlockHeight() uint64 {
|
|
if x != nil {
|
|
return x.BlockHeight
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (x *BlockRequest) GetBlockHash() *types.H256 {
|
|
if x != nil {
|
|
return x.BlockHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type BlockReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
BlockRlp []byte `protobuf:"bytes,1,opt,name=blockRlp,proto3" json:"blockRlp,omitempty"`
|
|
Senders []byte `protobuf:"bytes,2,opt,name=senders,proto3" json:"senders,omitempty"`
|
|
}
|
|
|
|
func (x *BlockReply) Reset() {
|
|
*x = BlockReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[21]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *BlockReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*BlockReply) ProtoMessage() {}
|
|
|
|
func (x *BlockReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[21]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use BlockReply.ProtoReflect.Descriptor instead.
|
|
func (*BlockReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{21}
|
|
}
|
|
|
|
func (x *BlockReply) GetBlockRlp() []byte {
|
|
if x != nil {
|
|
return x.BlockRlp
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func (x *BlockReply) GetSenders() []byte {
|
|
if x != nil {
|
|
return x.Senders
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type TxnLookupRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
TxnHash *types.H256 `protobuf:"bytes,1,opt,name=txnHash,proto3" json:"txnHash,omitempty"`
|
|
}
|
|
|
|
func (x *TxnLookupRequest) Reset() {
|
|
*x = TxnLookupRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[22]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *TxnLookupRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*TxnLookupRequest) ProtoMessage() {}
|
|
|
|
func (x *TxnLookupRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[22]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use TxnLookupRequest.ProtoReflect.Descriptor instead.
|
|
func (*TxnLookupRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{22}
|
|
}
|
|
|
|
func (x *TxnLookupRequest) GetTxnHash() *types.H256 {
|
|
if x != nil {
|
|
return x.TxnHash
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type TxnLookupReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
BlockNumber uint64 `protobuf:"varint,1,opt,name=blockNumber,proto3" json:"blockNumber,omitempty"`
|
|
}
|
|
|
|
func (x *TxnLookupReply) Reset() {
|
|
*x = TxnLookupReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[23]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *TxnLookupReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*TxnLookupReply) ProtoMessage() {}
|
|
|
|
func (x *TxnLookupReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[23]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use TxnLookupReply.ProtoReflect.Descriptor instead.
|
|
func (*TxnLookupReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{23}
|
|
}
|
|
|
|
func (x *TxnLookupReply) GetBlockNumber() uint64 {
|
|
if x != nil {
|
|
return x.BlockNumber
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type NodesInfoRequest struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Limit uint32 `protobuf:"varint,1,opt,name=limit,proto3" json:"limit,omitempty"`
|
|
}
|
|
|
|
func (x *NodesInfoRequest) Reset() {
|
|
*x = NodesInfoRequest{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[24]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *NodesInfoRequest) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NodesInfoRequest) ProtoMessage() {}
|
|
|
|
func (x *NodesInfoRequest) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[24]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NodesInfoRequest.ProtoReflect.Descriptor instead.
|
|
func (*NodesInfoRequest) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{24}
|
|
}
|
|
|
|
func (x *NodesInfoRequest) GetLimit() uint32 {
|
|
if x != nil {
|
|
return x.Limit
|
|
}
|
|
return 0
|
|
}
|
|
|
|
type NodesInfoReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
NodesInfo []*types.NodeInfoReply `protobuf:"bytes,1,rep,name=nodesInfo,proto3" json:"nodesInfo,omitempty"`
|
|
}
|
|
|
|
func (x *NodesInfoReply) Reset() {
|
|
*x = NodesInfoReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[25]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *NodesInfoReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*NodesInfoReply) ProtoMessage() {}
|
|
|
|
func (x *NodesInfoReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[25]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use NodesInfoReply.ProtoReflect.Descriptor instead.
|
|
func (*NodesInfoReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{25}
|
|
}
|
|
|
|
func (x *NodesInfoReply) GetNodesInfo() []*types.NodeInfoReply {
|
|
if x != nil {
|
|
return x.NodesInfo
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type PeersReply struct {
|
|
state protoimpl.MessageState
|
|
sizeCache protoimpl.SizeCache
|
|
unknownFields protoimpl.UnknownFields
|
|
|
|
Peers []*types.PeerInfo `protobuf:"bytes,1,rep,name=peers,proto3" json:"peers,omitempty"`
|
|
}
|
|
|
|
func (x *PeersReply) Reset() {
|
|
*x = PeersReply{}
|
|
if protoimpl.UnsafeEnabled {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[26]
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
}
|
|
|
|
func (x *PeersReply) String() string {
|
|
return protoimpl.X.MessageStringOf(x)
|
|
}
|
|
|
|
func (*PeersReply) ProtoMessage() {}
|
|
|
|
func (x *PeersReply) ProtoReflect() protoreflect.Message {
|
|
mi := &file_remote_ethbackend_proto_msgTypes[26]
|
|
if protoimpl.UnsafeEnabled && x != nil {
|
|
ms := protoimpl.X.MessageStateOf(protoimpl.Pointer(x))
|
|
if ms.LoadMessageInfo() == nil {
|
|
ms.StoreMessageInfo(mi)
|
|
}
|
|
return ms
|
|
}
|
|
return mi.MessageOf(x)
|
|
}
|
|
|
|
// Deprecated: Use PeersReply.ProtoReflect.Descriptor instead.
|
|
func (*PeersReply) Descriptor() ([]byte, []int) {
|
|
return file_remote_ethbackend_proto_rawDescGZIP(), []int{26}
|
|
}
|
|
|
|
func (x *PeersReply) GetPeers() []*types.PeerInfo {
|
|
if x != nil {
|
|
return x.Peers
|
|
}
|
|
return nil
|
|
}
|
|
|
|
var File_remote_ethbackend_proto protoreflect.FileDescriptor
|
|
|
|
var file_remote_ethbackend_proto_rawDesc = []byte{
|
|
0x0a, 0x17, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2f, 0x65, 0x74, 0x68, 0x62, 0x61, 0x63, 0x6b,
|
|
0x65, 0x6e, 0x64, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x12, 0x06, 0x72, 0x65, 0x6d, 0x6f, 0x74,
|
|
0x65, 0x1a, 0x1b, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2f, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x62,
|
|
0x75, 0x66, 0x2f, 0x65, 0x6d, 0x70, 0x74, 0x79, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x1a, 0x11,
|
|
0x74, 0x79, 0x70, 0x65, 0x73, 0x2f, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x22, 0x12, 0x0a, 0x10, 0x45, 0x74, 0x68, 0x65, 0x72, 0x62, 0x61, 0x73, 0x65, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0x37, 0x0a, 0x0e, 0x45, 0x74, 0x68, 0x65, 0x72, 0x62, 0x61,
|
|
0x73, 0x65, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x25, 0x0a, 0x07, 0x61, 0x64, 0x64, 0x72, 0x65,
|
|
0x73, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73,
|
|
0x2e, 0x48, 0x31, 0x36, 0x30, 0x52, 0x07, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x22, 0x13,
|
|
0x0a, 0x11, 0x4e, 0x65, 0x74, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x71, 0x75,
|
|
0x65, 0x73, 0x74, 0x22, 0x21, 0x0a, 0x0f, 0x4e, 0x65, 0x74, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f,
|
|
0x6e, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x0e, 0x0a, 0x02, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x04, 0x52, 0x02, 0x69, 0x64, 0x22, 0x15, 0x0a, 0x13, 0x4e, 0x65, 0x74, 0x50, 0x65, 0x65,
|
|
0x72, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0x29, 0x0a,
|
|
0x11, 0x4e, 0x65, 0x74, 0x50, 0x65, 0x65, 0x72, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x52, 0x65, 0x70,
|
|
0x6c, 0x79, 0x12, 0x14, 0x0a, 0x05, 0x63, 0x6f, 0x75, 0x6e, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x05, 0x63, 0x6f, 0x75, 0x6e, 0x74, 0x22, 0x37, 0x0a, 0x17, 0x45, 0x6e, 0x67, 0x69,
|
|
0x6e, 0x65, 0x47, 0x65, 0x74, 0x50, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x52, 0x65, 0x71, 0x75,
|
|
0x65, 0x73, 0x74, 0x12, 0x1c, 0x0a, 0x09, 0x70, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x49, 0x64,
|
|
0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x09, 0x70, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x49,
|
|
0x64, 0x22, 0xa4, 0x01, 0x0a, 0x13, 0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x50, 0x61, 0x79, 0x6c,
|
|
0x6f, 0x61, 0x64, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x12, 0x2c, 0x0a, 0x06, 0x73, 0x74, 0x61,
|
|
0x74, 0x75, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x14, 0x2e, 0x72, 0x65, 0x6d, 0x6f,
|
|
0x74, 0x65, 0x2e, 0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x52,
|
|
0x06, 0x73, 0x74, 0x61, 0x74, 0x75, 0x73, 0x12, 0x35, 0x0a, 0x0f, 0x6c, 0x61, 0x74, 0x65, 0x73,
|
|
0x74, 0x56, 0x61, 0x6c, 0x69, 0x64, 0x48, 0x61, 0x73, 0x68, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x48, 0x32, 0x35, 0x36, 0x52, 0x0f, 0x6c,
|
|
0x61, 0x74, 0x65, 0x73, 0x74, 0x56, 0x61, 0x6c, 0x69, 0x64, 0x48, 0x61, 0x73, 0x68, 0x12, 0x28,
|
|
0x0a, 0x0f, 0x76, 0x61, 0x6c, 0x69, 0x64, 0x61, 0x74, 0x69, 0x6f, 0x6e, 0x45, 0x72, 0x72, 0x6f,
|
|
0x72, 0x18, 0x03, 0x20, 0x01, 0x28, 0x09, 0x52, 0x0f, 0x76, 0x61, 0x6c, 0x69, 0x64, 0x61, 0x74,
|
|
0x69, 0x6f, 0x6e, 0x45, 0x72, 0x72, 0x6f, 0x72, 0x22, 0xa7, 0x01, 0x0a, 0x17, 0x45, 0x6e, 0x67,
|
|
0x69, 0x6e, 0x65, 0x50, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x41, 0x74, 0x74, 0x72, 0x69, 0x62,
|
|
0x75, 0x74, 0x65, 0x73, 0x12, 0x1c, 0x0a, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61, 0x6d,
|
|
0x70, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x09, 0x74, 0x69, 0x6d, 0x65, 0x73, 0x74, 0x61,
|
|
0x6d, 0x70, 0x12, 0x2b, 0x0a, 0x0a, 0x70, 0x72, 0x65, 0x76, 0x52, 0x61, 0x6e, 0x64, 0x61, 0x6f,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x48,
|
|
0x32, 0x35, 0x36, 0x52, 0x0a, 0x70, 0x72, 0x65, 0x76, 0x52, 0x61, 0x6e, 0x64, 0x61, 0x6f, 0x12,
|
|
0x41, 0x0a, 0x15, 0x73, 0x75, 0x67, 0x67, 0x65, 0x73, 0x74, 0x65, 0x64, 0x46, 0x65, 0x65, 0x52,
|
|
0x65, 0x63, 0x69, 0x70, 0x69, 0x65, 0x6e, 0x74, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0b,
|
|
0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x48, 0x31, 0x36, 0x30, 0x52, 0x15, 0x73, 0x75, 0x67,
|
|
0x67, 0x65, 0x73, 0x74, 0x65, 0x64, 0x46, 0x65, 0x65, 0x52, 0x65, 0x63, 0x69, 0x70, 0x69, 0x65,
|
|
0x6e, 0x74, 0x22, 0xba, 0x01, 0x0a, 0x15, 0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x46, 0x6f, 0x72,
|
|
0x6b, 0x43, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x53, 0x74, 0x61, 0x74, 0x65, 0x12, 0x31, 0x0a, 0x0d,
|
|
0x68, 0x65, 0x61, 0x64, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x0b, 0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x48, 0x32, 0x35, 0x36,
|
|
0x52, 0x0d, 0x68, 0x65, 0x61, 0x64, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x12,
|
|
0x31, 0x0a, 0x0d, 0x73, 0x61, 0x66, 0x65, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x48,
|
|
0x32, 0x35, 0x36, 0x52, 0x0d, 0x73, 0x61, 0x66, 0x65, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61,
|
|
0x73, 0x68, 0x12, 0x3b, 0x0a, 0x12, 0x66, 0x69, 0x6e, 0x61, 0x6c, 0x69, 0x7a, 0x65, 0x64, 0x42,
|
|
0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x18, 0x03, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0b,
|
|
0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x48, 0x32, 0x35, 0x36, 0x52, 0x12, 0x66, 0x69, 0x6e,
|
|
0x61, 0x6c, 0x69, 0x7a, 0x65, 0x64, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x22,
|
|
0xb8, 0x01, 0x0a, 0x1e, 0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x46, 0x6f, 0x72, 0x6b, 0x43, 0x68,
|
|
0x6f, 0x69, 0x63, 0x65, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x64, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x12, 0x47, 0x0a, 0x0f, 0x66, 0x6f, 0x72, 0x6b, 0x63, 0x68, 0x6f, 0x69, 0x63, 0x65,
|
|
0x53, 0x74, 0x61, 0x74, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1d, 0x2e, 0x72, 0x65,
|
|
0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x46, 0x6f, 0x72, 0x6b, 0x43,
|
|
0x68, 0x6f, 0x69, 0x63, 0x65, 0x53, 0x74, 0x61, 0x74, 0x65, 0x52, 0x0f, 0x66, 0x6f, 0x72, 0x6b,
|
|
0x63, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x53, 0x74, 0x61, 0x74, 0x65, 0x12, 0x4d, 0x0a, 0x11, 0x70,
|
|
0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x41, 0x74, 0x74, 0x72, 0x69, 0x62, 0x75, 0x74, 0x65, 0x73,
|
|
0x18, 0x02, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x1f, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e,
|
|
0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x50, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x41, 0x74, 0x74,
|
|
0x72, 0x69, 0x62, 0x75, 0x74, 0x65, 0x73, 0x52, 0x11, 0x70, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64,
|
|
0x41, 0x74, 0x74, 0x72, 0x69, 0x62, 0x75, 0x74, 0x65, 0x73, 0x22, 0x7f, 0x0a, 0x1c, 0x45, 0x6e,
|
|
0x67, 0x69, 0x6e, 0x65, 0x46, 0x6f, 0x72, 0x6b, 0x43, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x55, 0x70,
|
|
0x64, 0x61, 0x74, 0x65, 0x64, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x41, 0x0a, 0x0d, 0x70, 0x61,
|
|
0x79, 0x6c, 0x6f, 0x61, 0x64, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x18, 0x01, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x1b, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x45, 0x6e, 0x67, 0x69, 0x6e,
|
|
0x65, 0x50, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x52, 0x0d,
|
|
0x70, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x12, 0x1c, 0x0a,
|
|
0x09, 0x70, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x49, 0x64, 0x18, 0x02, 0x20, 0x01, 0x28, 0x04,
|
|
0x52, 0x09, 0x70, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x49, 0x64, 0x22, 0x18, 0x0a, 0x16, 0x50,
|
|
0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0x26, 0x0a, 0x14, 0x50, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f,
|
|
0x6c, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x0e, 0x0a,
|
|
0x02, 0x69, 0x64, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52, 0x02, 0x69, 0x64, 0x22, 0x16, 0x0a,
|
|
0x14, 0x43, 0x6c, 0x69, 0x65, 0x6e, 0x74, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x22, 0x30, 0x0a, 0x12, 0x43, 0x6c, 0x69, 0x65, 0x6e, 0x74, 0x56,
|
|
0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x1a, 0x0a, 0x08, 0x6e,
|
|
0x6f, 0x64, 0x65, 0x4e, 0x61, 0x6d, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x09, 0x52, 0x08, 0x6e,
|
|
0x6f, 0x64, 0x65, 0x4e, 0x61, 0x6d, 0x65, 0x22, 0x35, 0x0a, 0x10, 0x53, 0x75, 0x62, 0x73, 0x63,
|
|
0x72, 0x69, 0x62, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x21, 0x0a, 0x04, 0x74,
|
|
0x79, 0x70, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x0d, 0x2e, 0x72, 0x65, 0x6d, 0x6f,
|
|
0x74, 0x65, 0x2e, 0x45, 0x76, 0x65, 0x6e, 0x74, 0x52, 0x04, 0x74, 0x79, 0x70, 0x65, 0x22, 0x47,
|
|
0x0a, 0x0e, 0x53, 0x75, 0x62, 0x73, 0x63, 0x72, 0x69, 0x62, 0x65, 0x52, 0x65, 0x70, 0x6c, 0x79,
|
|
0x12, 0x21, 0x0a, 0x04, 0x74, 0x79, 0x70, 0x65, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0e, 0x32, 0x0d,
|
|
0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x45, 0x76, 0x65, 0x6e, 0x74, 0x52, 0x04, 0x74,
|
|
0x79, 0x70, 0x65, 0x12, 0x12, 0x0a, 0x04, 0x64, 0x61, 0x74, 0x61, 0x18, 0x02, 0x20, 0x01, 0x28,
|
|
0x0c, 0x52, 0x04, 0x64, 0x61, 0x74, 0x61, 0x22, 0xa5, 0x01, 0x0a, 0x11, 0x4c, 0x6f, 0x67, 0x73,
|
|
0x46, 0x69, 0x6c, 0x74, 0x65, 0x72, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x22, 0x0a,
|
|
0x0c, 0x61, 0x6c, 0x6c, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x18, 0x01, 0x20,
|
|
0x01, 0x28, 0x08, 0x52, 0x0c, 0x61, 0x6c, 0x6c, 0x41, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65,
|
|
0x73, 0x12, 0x29, 0x0a, 0x09, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x18, 0x02,
|
|
0x20, 0x03, 0x28, 0x0b, 0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x48, 0x31, 0x36,
|
|
0x30, 0x52, 0x09, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x65, 0x73, 0x12, 0x1c, 0x0a, 0x09,
|
|
0x61, 0x6c, 0x6c, 0x54, 0x6f, 0x70, 0x69, 0x63, 0x73, 0x18, 0x03, 0x20, 0x01, 0x28, 0x08, 0x52,
|
|
0x09, 0x61, 0x6c, 0x6c, 0x54, 0x6f, 0x70, 0x69, 0x63, 0x73, 0x12, 0x23, 0x0a, 0x06, 0x74, 0x6f,
|
|
0x70, 0x69, 0x63, 0x73, 0x18, 0x04, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70,
|
|
0x65, 0x73, 0x2e, 0x48, 0x32, 0x35, 0x36, 0x52, 0x06, 0x74, 0x6f, 0x70, 0x69, 0x63, 0x73, 0x22,
|
|
0xda, 0x02, 0x0a, 0x12, 0x53, 0x75, 0x62, 0x73, 0x63, 0x72, 0x69, 0x62, 0x65, 0x4c, 0x6f, 0x67,
|
|
0x73, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x25, 0x0a, 0x07, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73,
|
|
0x73, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e,
|
|
0x48, 0x31, 0x36, 0x30, 0x52, 0x07, 0x61, 0x64, 0x64, 0x72, 0x65, 0x73, 0x73, 0x12, 0x29, 0x0a,
|
|
0x09, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x18, 0x02, 0x20, 0x01, 0x28, 0x0b,
|
|
0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x48, 0x32, 0x35, 0x36, 0x52, 0x09, 0x62,
|
|
0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x12, 0x20, 0x0a, 0x0b, 0x62, 0x6c, 0x6f, 0x63,
|
|
0x6b, 0x4e, 0x75, 0x6d, 0x62, 0x65, 0x72, 0x18, 0x03, 0x20, 0x01, 0x28, 0x04, 0x52, 0x0b, 0x62,
|
|
0x6c, 0x6f, 0x63, 0x6b, 0x4e, 0x75, 0x6d, 0x62, 0x65, 0x72, 0x12, 0x12, 0x0a, 0x04, 0x64, 0x61,
|
|
0x74, 0x61, 0x18, 0x04, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x04, 0x64, 0x61, 0x74, 0x61, 0x12, 0x1a,
|
|
0x0a, 0x08, 0x6c, 0x6f, 0x67, 0x49, 0x6e, 0x64, 0x65, 0x78, 0x18, 0x05, 0x20, 0x01, 0x28, 0x04,
|
|
0x52, 0x08, 0x6c, 0x6f, 0x67, 0x49, 0x6e, 0x64, 0x65, 0x78, 0x12, 0x23, 0x0a, 0x06, 0x74, 0x6f,
|
|
0x70, 0x69, 0x63, 0x73, 0x18, 0x06, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70,
|
|
0x65, 0x73, 0x2e, 0x48, 0x32, 0x35, 0x36, 0x52, 0x06, 0x74, 0x6f, 0x70, 0x69, 0x63, 0x73, 0x12,
|
|
0x35, 0x0a, 0x0f, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x48, 0x61,
|
|
0x73, 0x68, 0x18, 0x07, 0x20, 0x01, 0x28, 0x0b, 0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73,
|
|
0x2e, 0x48, 0x32, 0x35, 0x36, 0x52, 0x0f, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69,
|
|
0x6f, 0x6e, 0x48, 0x61, 0x73, 0x68, 0x12, 0x2a, 0x0a, 0x10, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61,
|
|
0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x6e, 0x64, 0x65, 0x78, 0x18, 0x08, 0x20, 0x01, 0x28, 0x04,
|
|
0x52, 0x10, 0x74, 0x72, 0x61, 0x6e, 0x73, 0x61, 0x63, 0x74, 0x69, 0x6f, 0x6e, 0x49, 0x6e, 0x64,
|
|
0x65, 0x78, 0x12, 0x18, 0x0a, 0x07, 0x72, 0x65, 0x6d, 0x6f, 0x76, 0x65, 0x64, 0x18, 0x09, 0x20,
|
|
0x01, 0x28, 0x08, 0x52, 0x07, 0x72, 0x65, 0x6d, 0x6f, 0x76, 0x65, 0x64, 0x22, 0x5b, 0x0a, 0x0c,
|
|
0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x12, 0x20, 0x0a, 0x0b,
|
|
0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x65, 0x69, 0x67, 0x68, 0x74, 0x18, 0x02, 0x20, 0x01, 0x28,
|
|
0x04, 0x52, 0x0b, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x65, 0x69, 0x67, 0x68, 0x74, 0x12, 0x29,
|
|
0x0a, 0x09, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x18, 0x03, 0x20, 0x01, 0x28,
|
|
0x0b, 0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x48, 0x32, 0x35, 0x36, 0x52, 0x09,
|
|
0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x48, 0x61, 0x73, 0x68, 0x22, 0x42, 0x0a, 0x0a, 0x42, 0x6c, 0x6f,
|
|
0x63, 0x6b, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x1a, 0x0a, 0x08, 0x62, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x52, 0x6c, 0x70, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0c, 0x52, 0x08, 0x62, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x52, 0x6c, 0x70, 0x12, 0x18, 0x0a, 0x07, 0x73, 0x65, 0x6e, 0x64, 0x65, 0x72, 0x73, 0x18, 0x02,
|
|
0x20, 0x01, 0x28, 0x0c, 0x52, 0x07, 0x73, 0x65, 0x6e, 0x64, 0x65, 0x72, 0x73, 0x22, 0x39, 0x0a,
|
|
0x10, 0x54, 0x78, 0x6e, 0x4c, 0x6f, 0x6f, 0x6b, 0x75, 0x70, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73,
|
|
0x74, 0x12, 0x25, 0x0a, 0x07, 0x74, 0x78, 0x6e, 0x48, 0x61, 0x73, 0x68, 0x18, 0x01, 0x20, 0x01,
|
|
0x28, 0x0b, 0x32, 0x0b, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x48, 0x32, 0x35, 0x36, 0x52,
|
|
0x07, 0x74, 0x78, 0x6e, 0x48, 0x61, 0x73, 0x68, 0x22, 0x32, 0x0a, 0x0e, 0x54, 0x78, 0x6e, 0x4c,
|
|
0x6f, 0x6f, 0x6b, 0x75, 0x70, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x20, 0x0a, 0x0b, 0x62, 0x6c,
|
|
0x6f, 0x63, 0x6b, 0x4e, 0x75, 0x6d, 0x62, 0x65, 0x72, 0x18, 0x01, 0x20, 0x01, 0x28, 0x04, 0x52,
|
|
0x0b, 0x62, 0x6c, 0x6f, 0x63, 0x6b, 0x4e, 0x75, 0x6d, 0x62, 0x65, 0x72, 0x22, 0x28, 0x0a, 0x10,
|
|
0x4e, 0x6f, 0x64, 0x65, 0x73, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74,
|
|
0x12, 0x14, 0x0a, 0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x18, 0x01, 0x20, 0x01, 0x28, 0x0d, 0x52,
|
|
0x05, 0x6c, 0x69, 0x6d, 0x69, 0x74, 0x22, 0x44, 0x0a, 0x0e, 0x4e, 0x6f, 0x64, 0x65, 0x73, 0x49,
|
|
0x6e, 0x66, 0x6f, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x32, 0x0a, 0x09, 0x6e, 0x6f, 0x64, 0x65,
|
|
0x73, 0x49, 0x6e, 0x66, 0x6f, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x14, 0x2e, 0x74, 0x79,
|
|
0x70, 0x65, 0x73, 0x2e, 0x4e, 0x6f, 0x64, 0x65, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x70, 0x6c,
|
|
0x79, 0x52, 0x09, 0x6e, 0x6f, 0x64, 0x65, 0x73, 0x49, 0x6e, 0x66, 0x6f, 0x22, 0x33, 0x0a, 0x0a,
|
|
0x50, 0x65, 0x65, 0x72, 0x73, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x25, 0x0a, 0x05, 0x70, 0x65,
|
|
0x65, 0x72, 0x73, 0x18, 0x01, 0x20, 0x03, 0x28, 0x0b, 0x32, 0x0f, 0x2e, 0x74, 0x79, 0x70, 0x65,
|
|
0x73, 0x2e, 0x50, 0x65, 0x65, 0x72, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x05, 0x70, 0x65, 0x65, 0x72,
|
|
0x73, 0x2a, 0x4a, 0x0a, 0x05, 0x45, 0x76, 0x65, 0x6e, 0x74, 0x12, 0x0a, 0x0a, 0x06, 0x48, 0x45,
|
|
0x41, 0x44, 0x45, 0x52, 0x10, 0x00, 0x12, 0x10, 0x0a, 0x0c, 0x50, 0x45, 0x4e, 0x44, 0x49, 0x4e,
|
|
0x47, 0x5f, 0x4c, 0x4f, 0x47, 0x53, 0x10, 0x01, 0x12, 0x11, 0x0a, 0x0d, 0x50, 0x45, 0x4e, 0x44,
|
|
0x49, 0x4e, 0x47, 0x5f, 0x42, 0x4c, 0x4f, 0x43, 0x4b, 0x10, 0x02, 0x12, 0x10, 0x0a, 0x0c, 0x4e,
|
|
0x45, 0x57, 0x5f, 0x53, 0x4e, 0x41, 0x50, 0x53, 0x48, 0x4f, 0x54, 0x10, 0x03, 0x2a, 0x59, 0x0a,
|
|
0x0c, 0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x12, 0x09, 0x0a,
|
|
0x05, 0x56, 0x41, 0x4c, 0x49, 0x44, 0x10, 0x00, 0x12, 0x0b, 0x0a, 0x07, 0x49, 0x4e, 0x56, 0x41,
|
|
0x4c, 0x49, 0x44, 0x10, 0x01, 0x12, 0x0b, 0x0a, 0x07, 0x53, 0x59, 0x4e, 0x43, 0x49, 0x4e, 0x47,
|
|
0x10, 0x02, 0x12, 0x0c, 0x0a, 0x08, 0x41, 0x43, 0x43, 0x45, 0x50, 0x54, 0x45, 0x44, 0x10, 0x03,
|
|
0x12, 0x16, 0x0a, 0x12, 0x49, 0x4e, 0x56, 0x41, 0x4c, 0x49, 0x44, 0x5f, 0x42, 0x4c, 0x4f, 0x43,
|
|
0x4b, 0x5f, 0x48, 0x41, 0x53, 0x48, 0x10, 0x04, 0x32, 0xa2, 0x08, 0x0a, 0x0a, 0x45, 0x54, 0x48,
|
|
0x42, 0x41, 0x43, 0x4b, 0x45, 0x4e, 0x44, 0x12, 0x3d, 0x0a, 0x09, 0x45, 0x74, 0x68, 0x65, 0x72,
|
|
0x62, 0x61, 0x73, 0x65, 0x12, 0x18, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x45, 0x74,
|
|
0x68, 0x65, 0x72, 0x62, 0x61, 0x73, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x16,
|
|
0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x45, 0x74, 0x68, 0x65, 0x72, 0x62, 0x61, 0x73,
|
|
0x65, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x40, 0x0a, 0x0a, 0x4e, 0x65, 0x74, 0x56, 0x65, 0x72,
|
|
0x73, 0x69, 0x6f, 0x6e, 0x12, 0x19, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x4e, 0x65,
|
|
0x74, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a,
|
|
0x17, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x4e, 0x65, 0x74, 0x56, 0x65, 0x72, 0x73,
|
|
0x69, 0x6f, 0x6e, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x46, 0x0a, 0x0c, 0x4e, 0x65, 0x74, 0x50,
|
|
0x65, 0x65, 0x72, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x12, 0x1b, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74,
|
|
0x65, 0x2e, 0x4e, 0x65, 0x74, 0x50, 0x65, 0x65, 0x72, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x52, 0x65,
|
|
0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x19, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x4e,
|
|
0x65, 0x74, 0x50, 0x65, 0x65, 0x72, 0x43, 0x6f, 0x75, 0x6e, 0x74, 0x52, 0x65, 0x70, 0x6c, 0x79,
|
|
0x12, 0x4e, 0x0a, 0x12, 0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x47, 0x65, 0x74, 0x50, 0x61, 0x79,
|
|
0x6c, 0x6f, 0x61, 0x64, 0x56, 0x31, 0x12, 0x1f, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e,
|
|
0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x47, 0x65, 0x74, 0x50, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64,
|
|
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x17, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e,
|
|
0x45, 0x78, 0x65, 0x63, 0x75, 0x74, 0x69, 0x6f, 0x6e, 0x50, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64,
|
|
0x12, 0x4a, 0x0a, 0x12, 0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x4e, 0x65, 0x77, 0x50, 0x61, 0x79,
|
|
0x6c, 0x6f, 0x61, 0x64, 0x56, 0x31, 0x12, 0x17, 0x2e, 0x74, 0x79, 0x70, 0x65, 0x73, 0x2e, 0x45,
|
|
0x78, 0x65, 0x63, 0x75, 0x74, 0x69, 0x6f, 0x6e, 0x50, 0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x1a,
|
|
0x1b, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x50,
|
|
0x61, 0x79, 0x6c, 0x6f, 0x61, 0x64, 0x53, 0x74, 0x61, 0x74, 0x75, 0x73, 0x12, 0x69, 0x0a, 0x19,
|
|
0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x46, 0x6f, 0x72, 0x6b, 0x43, 0x68, 0x6f, 0x69, 0x63, 0x65,
|
|
0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x64, 0x56, 0x31, 0x12, 0x26, 0x2e, 0x72, 0x65, 0x6d, 0x6f,
|
|
0x74, 0x65, 0x2e, 0x45, 0x6e, 0x67, 0x69, 0x6e, 0x65, 0x46, 0x6f, 0x72, 0x6b, 0x43, 0x68, 0x6f,
|
|
0x69, 0x63, 0x65, 0x55, 0x70, 0x64, 0x61, 0x74, 0x65, 0x64, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73,
|
|
0x74, 0x1a, 0x24, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x45, 0x6e, 0x67, 0x69, 0x6e,
|
|
0x65, 0x46, 0x6f, 0x72, 0x6b, 0x43, 0x68, 0x6f, 0x69, 0x63, 0x65, 0x55, 0x70, 0x64, 0x61, 0x74,
|
|
0x65, 0x64, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x36, 0x0a, 0x07, 0x56, 0x65, 0x72, 0x73, 0x69,
|
|
0x6f, 0x6e, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x13, 0x2e, 0x74, 0x79, 0x70,
|
|
0x65, 0x73, 0x2e, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12,
|
|
0x4f, 0x0a, 0x0f, 0x50, 0x72, 0x6f, 0x74, 0x6f, 0x63, 0x6f, 0x6c, 0x56, 0x65, 0x72, 0x73, 0x69,
|
|
0x6f, 0x6e, 0x12, 0x1e, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x50, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x63, 0x6f, 0x6c, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x1a, 0x1c, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x50, 0x72, 0x6f, 0x74,
|
|
0x6f, 0x63, 0x6f, 0x6c, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x70, 0x6c, 0x79,
|
|
0x12, 0x49, 0x0a, 0x0d, 0x43, 0x6c, 0x69, 0x65, 0x6e, 0x74, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f,
|
|
0x6e, 0x12, 0x1c, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x43, 0x6c, 0x69, 0x65, 0x6e,
|
|
0x74, 0x56, 0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a,
|
|
0x1a, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x43, 0x6c, 0x69, 0x65, 0x6e, 0x74, 0x56,
|
|
0x65, 0x72, 0x73, 0x69, 0x6f, 0x6e, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x3f, 0x0a, 0x09, 0x53,
|
|
0x75, 0x62, 0x73, 0x63, 0x72, 0x69, 0x62, 0x65, 0x12, 0x18, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74,
|
|
0x65, 0x2e, 0x53, 0x75, 0x62, 0x73, 0x63, 0x72, 0x69, 0x62, 0x65, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x1a, 0x16, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x53, 0x75, 0x62, 0x73,
|
|
0x63, 0x72, 0x69, 0x62, 0x65, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x30, 0x01, 0x12, 0x4a, 0x0a, 0x0d,
|
|
0x53, 0x75, 0x62, 0x73, 0x63, 0x72, 0x69, 0x62, 0x65, 0x4c, 0x6f, 0x67, 0x73, 0x12, 0x19, 0x2e,
|
|
0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x4c, 0x6f, 0x67, 0x73, 0x46, 0x69, 0x6c, 0x74, 0x65,
|
|
0x72, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x1a, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74,
|
|
0x65, 0x2e, 0x53, 0x75, 0x62, 0x73, 0x63, 0x72, 0x69, 0x62, 0x65, 0x4c, 0x6f, 0x67, 0x73, 0x52,
|
|
0x65, 0x70, 0x6c, 0x79, 0x28, 0x01, 0x30, 0x01, 0x12, 0x31, 0x0a, 0x05, 0x42, 0x6c, 0x6f, 0x63,
|
|
0x6b, 0x12, 0x14, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x42, 0x6c, 0x6f, 0x63, 0x6b,
|
|
0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74, 0x1a, 0x12, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65,
|
|
0x2e, 0x42, 0x6c, 0x6f, 0x63, 0x6b, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x3d, 0x0a, 0x09, 0x54,
|
|
0x78, 0x6e, 0x4c, 0x6f, 0x6f, 0x6b, 0x75, 0x70, 0x12, 0x18, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74,
|
|
0x65, 0x2e, 0x54, 0x78, 0x6e, 0x4c, 0x6f, 0x6f, 0x6b, 0x75, 0x70, 0x52, 0x65, 0x71, 0x75, 0x65,
|
|
0x73, 0x74, 0x1a, 0x16, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x54, 0x78, 0x6e, 0x4c,
|
|
0x6f, 0x6f, 0x6b, 0x75, 0x70, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x3c, 0x0a, 0x08, 0x4e, 0x6f,
|
|
0x64, 0x65, 0x49, 0x6e, 0x66, 0x6f, 0x12, 0x18, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e,
|
|
0x4e, 0x6f, 0x64, 0x65, 0x73, 0x49, 0x6e, 0x66, 0x6f, 0x52, 0x65, 0x71, 0x75, 0x65, 0x73, 0x74,
|
|
0x1a, 0x16, 0x2e, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x2e, 0x4e, 0x6f, 0x64, 0x65, 0x73, 0x49,
|
|
0x6e, 0x66, 0x6f, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x12, 0x33, 0x0a, 0x05, 0x50, 0x65, 0x65, 0x72,
|
|
0x73, 0x12, 0x16, 0x2e, 0x67, 0x6f, 0x6f, 0x67, 0x6c, 0x65, 0x2e, 0x70, 0x72, 0x6f, 0x74, 0x6f,
|
|
0x62, 0x75, 0x66, 0x2e, 0x45, 0x6d, 0x70, 0x74, 0x79, 0x1a, 0x12, 0x2e, 0x72, 0x65, 0x6d, 0x6f,
|
|
0x74, 0x65, 0x2e, 0x50, 0x65, 0x65, 0x72, 0x73, 0x52, 0x65, 0x70, 0x6c, 0x79, 0x42, 0x11, 0x5a,
|
|
0x0f, 0x2e, 0x2f, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65, 0x3b, 0x72, 0x65, 0x6d, 0x6f, 0x74, 0x65,
|
|
0x62, 0x06, 0x70, 0x72, 0x6f, 0x74, 0x6f, 0x33,
|
|
}
|
|
|
|
var (
|
|
file_remote_ethbackend_proto_rawDescOnce sync.Once
|
|
file_remote_ethbackend_proto_rawDescData = file_remote_ethbackend_proto_rawDesc
|
|
)
|
|
|
|
func file_remote_ethbackend_proto_rawDescGZIP() []byte {
|
|
file_remote_ethbackend_proto_rawDescOnce.Do(func() {
|
|
file_remote_ethbackend_proto_rawDescData = protoimpl.X.CompressGZIP(file_remote_ethbackend_proto_rawDescData)
|
|
})
|
|
return file_remote_ethbackend_proto_rawDescData
|
|
}
|
|
|
|
var file_remote_ethbackend_proto_enumTypes = make([]protoimpl.EnumInfo, 2)
|
|
var file_remote_ethbackend_proto_msgTypes = make([]protoimpl.MessageInfo, 27)
|
|
var file_remote_ethbackend_proto_goTypes = []interface{}{
|
|
(Event)(0), // 0: remote.Event
|
|
(EngineStatus)(0), // 1: remote.EngineStatus
|
|
(*EtherbaseRequest)(nil), // 2: remote.EtherbaseRequest
|
|
(*EtherbaseReply)(nil), // 3: remote.EtherbaseReply
|
|
(*NetVersionRequest)(nil), // 4: remote.NetVersionRequest
|
|
(*NetVersionReply)(nil), // 5: remote.NetVersionReply
|
|
(*NetPeerCountRequest)(nil), // 6: remote.NetPeerCountRequest
|
|
(*NetPeerCountReply)(nil), // 7: remote.NetPeerCountReply
|
|
(*EngineGetPayloadRequest)(nil), // 8: remote.EngineGetPayloadRequest
|
|
(*EnginePayloadStatus)(nil), // 9: remote.EnginePayloadStatus
|
|
(*EnginePayloadAttributes)(nil), // 10: remote.EnginePayloadAttributes
|
|
(*EngineForkChoiceState)(nil), // 11: remote.EngineForkChoiceState
|
|
(*EngineForkChoiceUpdatedRequest)(nil), // 12: remote.EngineForkChoiceUpdatedRequest
|
|
(*EngineForkChoiceUpdatedReply)(nil), // 13: remote.EngineForkChoiceUpdatedReply
|
|
(*ProtocolVersionRequest)(nil), // 14: remote.ProtocolVersionRequest
|
|
(*ProtocolVersionReply)(nil), // 15: remote.ProtocolVersionReply
|
|
(*ClientVersionRequest)(nil), // 16: remote.ClientVersionRequest
|
|
(*ClientVersionReply)(nil), // 17: remote.ClientVersionReply
|
|
(*SubscribeRequest)(nil), // 18: remote.SubscribeRequest
|
|
(*SubscribeReply)(nil), // 19: remote.SubscribeReply
|
|
(*LogsFilterRequest)(nil), // 20: remote.LogsFilterRequest
|
|
(*SubscribeLogsReply)(nil), // 21: remote.SubscribeLogsReply
|
|
(*BlockRequest)(nil), // 22: remote.BlockRequest
|
|
(*BlockReply)(nil), // 23: remote.BlockReply
|
|
(*TxnLookupRequest)(nil), // 24: remote.TxnLookupRequest
|
|
(*TxnLookupReply)(nil), // 25: remote.TxnLookupReply
|
|
(*NodesInfoRequest)(nil), // 26: remote.NodesInfoRequest
|
|
(*NodesInfoReply)(nil), // 27: remote.NodesInfoReply
|
|
(*PeersReply)(nil), // 28: remote.PeersReply
|
|
(*types.H160)(nil), // 29: types.H160
|
|
(*types.H256)(nil), // 30: types.H256
|
|
(*types.NodeInfoReply)(nil), // 31: types.NodeInfoReply
|
|
(*types.PeerInfo)(nil), // 32: types.PeerInfo
|
|
(*types.ExecutionPayload)(nil), // 33: types.ExecutionPayload
|
|
(*emptypb.Empty)(nil), // 34: google.protobuf.Empty
|
|
(*types.VersionReply)(nil), // 35: types.VersionReply
|
|
}
|
|
var file_remote_ethbackend_proto_depIdxs = []int32{
|
|
29, // 0: remote.EtherbaseReply.address:type_name -> types.H160
|
|
1, // 1: remote.EnginePayloadStatus.status:type_name -> remote.EngineStatus
|
|
30, // 2: remote.EnginePayloadStatus.latestValidHash:type_name -> types.H256
|
|
30, // 3: remote.EnginePayloadAttributes.prevRandao:type_name -> types.H256
|
|
29, // 4: remote.EnginePayloadAttributes.suggestedFeeRecipient:type_name -> types.H160
|
|
30, // 5: remote.EngineForkChoiceState.headBlockHash:type_name -> types.H256
|
|
30, // 6: remote.EngineForkChoiceState.safeBlockHash:type_name -> types.H256
|
|
30, // 7: remote.EngineForkChoiceState.finalizedBlockHash:type_name -> types.H256
|
|
11, // 8: remote.EngineForkChoiceUpdatedRequest.forkchoiceState:type_name -> remote.EngineForkChoiceState
|
|
10, // 9: remote.EngineForkChoiceUpdatedRequest.payloadAttributes:type_name -> remote.EnginePayloadAttributes
|
|
9, // 10: remote.EngineForkChoiceUpdatedReply.payloadStatus:type_name -> remote.EnginePayloadStatus
|
|
0, // 11: remote.SubscribeRequest.type:type_name -> remote.Event
|
|
0, // 12: remote.SubscribeReply.type:type_name -> remote.Event
|
|
29, // 13: remote.LogsFilterRequest.addresses:type_name -> types.H160
|
|
30, // 14: remote.LogsFilterRequest.topics:type_name -> types.H256
|
|
29, // 15: remote.SubscribeLogsReply.address:type_name -> types.H160
|
|
30, // 16: remote.SubscribeLogsReply.blockHash:type_name -> types.H256
|
|
30, // 17: remote.SubscribeLogsReply.topics:type_name -> types.H256
|
|
30, // 18: remote.SubscribeLogsReply.transactionHash:type_name -> types.H256
|
|
30, // 19: remote.BlockRequest.blockHash:type_name -> types.H256
|
|
30, // 20: remote.TxnLookupRequest.txnHash:type_name -> types.H256
|
|
31, // 21: remote.NodesInfoReply.nodesInfo:type_name -> types.NodeInfoReply
|
|
32, // 22: remote.PeersReply.peers:type_name -> types.PeerInfo
|
|
2, // 23: remote.ETHBACKEND.Etherbase:input_type -> remote.EtherbaseRequest
|
|
4, // 24: remote.ETHBACKEND.NetVersion:input_type -> remote.NetVersionRequest
|
|
6, // 25: remote.ETHBACKEND.NetPeerCount:input_type -> remote.NetPeerCountRequest
|
|
8, // 26: remote.ETHBACKEND.EngineGetPayloadV1:input_type -> remote.EngineGetPayloadRequest
|
|
33, // 27: remote.ETHBACKEND.EngineNewPayloadV1:input_type -> types.ExecutionPayload
|
|
12, // 28: remote.ETHBACKEND.EngineForkChoiceUpdatedV1:input_type -> remote.EngineForkChoiceUpdatedRequest
|
|
34, // 29: remote.ETHBACKEND.Version:input_type -> google.protobuf.Empty
|
|
14, // 30: remote.ETHBACKEND.ProtocolVersion:input_type -> remote.ProtocolVersionRequest
|
|
16, // 31: remote.ETHBACKEND.ClientVersion:input_type -> remote.ClientVersionRequest
|
|
18, // 32: remote.ETHBACKEND.Subscribe:input_type -> remote.SubscribeRequest
|
|
20, // 33: remote.ETHBACKEND.SubscribeLogs:input_type -> remote.LogsFilterRequest
|
|
22, // 34: remote.ETHBACKEND.Block:input_type -> remote.BlockRequest
|
|
24, // 35: remote.ETHBACKEND.TxnLookup:input_type -> remote.TxnLookupRequest
|
|
26, // 36: remote.ETHBACKEND.NodeInfo:input_type -> remote.NodesInfoRequest
|
|
34, // 37: remote.ETHBACKEND.Peers:input_type -> google.protobuf.Empty
|
|
3, // 38: remote.ETHBACKEND.Etherbase:output_type -> remote.EtherbaseReply
|
|
5, // 39: remote.ETHBACKEND.NetVersion:output_type -> remote.NetVersionReply
|
|
7, // 40: remote.ETHBACKEND.NetPeerCount:output_type -> remote.NetPeerCountReply
|
|
33, // 41: remote.ETHBACKEND.EngineGetPayloadV1:output_type -> types.ExecutionPayload
|
|
9, // 42: remote.ETHBACKEND.EngineNewPayloadV1:output_type -> remote.EnginePayloadStatus
|
|
13, // 43: remote.ETHBACKEND.EngineForkChoiceUpdatedV1:output_type -> remote.EngineForkChoiceUpdatedReply
|
|
35, // 44: remote.ETHBACKEND.Version:output_type -> types.VersionReply
|
|
15, // 45: remote.ETHBACKEND.ProtocolVersion:output_type -> remote.ProtocolVersionReply
|
|
17, // 46: remote.ETHBACKEND.ClientVersion:output_type -> remote.ClientVersionReply
|
|
19, // 47: remote.ETHBACKEND.Subscribe:output_type -> remote.SubscribeReply
|
|
21, // 48: remote.ETHBACKEND.SubscribeLogs:output_type -> remote.SubscribeLogsReply
|
|
23, // 49: remote.ETHBACKEND.Block:output_type -> remote.BlockReply
|
|
25, // 50: remote.ETHBACKEND.TxnLookup:output_type -> remote.TxnLookupReply
|
|
27, // 51: remote.ETHBACKEND.NodeInfo:output_type -> remote.NodesInfoReply
|
|
28, // 52: remote.ETHBACKEND.Peers:output_type -> remote.PeersReply
|
|
38, // [38:53] is the sub-list for method output_type
|
|
23, // [23:38] is the sub-list for method input_type
|
|
23, // [23:23] is the sub-list for extension type_name
|
|
23, // [23:23] is the sub-list for extension extendee
|
|
0, // [0:23] is the sub-list for field type_name
|
|
}
|
|
|
|
func init() { file_remote_ethbackend_proto_init() }
|
|
func file_remote_ethbackend_proto_init() {
|
|
if File_remote_ethbackend_proto != nil {
|
|
return
|
|
}
|
|
if !protoimpl.UnsafeEnabled {
|
|
file_remote_ethbackend_proto_msgTypes[0].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*EtherbaseRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[1].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*EtherbaseReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[2].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*NetVersionRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[3].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*NetVersionReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[4].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*NetPeerCountRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[5].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*NetPeerCountReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[6].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*EngineGetPayloadRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[7].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*EnginePayloadStatus); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[8].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*EnginePayloadAttributes); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[9].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*EngineForkChoiceState); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[10].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*EngineForkChoiceUpdatedRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[11].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*EngineForkChoiceUpdatedReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[12].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ProtocolVersionRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[13].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ProtocolVersionReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[14].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ClientVersionRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[15].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*ClientVersionReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[16].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*SubscribeRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[17].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*SubscribeReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[18].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*LogsFilterRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[19].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*SubscribeLogsReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[20].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BlockRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[21].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*BlockReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[22].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*TxnLookupRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[23].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*TxnLookupReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[24].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*NodesInfoRequest); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[25].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*NodesInfoReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
file_remote_ethbackend_proto_msgTypes[26].Exporter = func(v interface{}, i int) interface{} {
|
|
switch v := v.(*PeersReply); i {
|
|
case 0:
|
|
return &v.state
|
|
case 1:
|
|
return &v.sizeCache
|
|
case 2:
|
|
return &v.unknownFields
|
|
default:
|
|
return nil
|
|
}
|
|
}
|
|
}
|
|
type x struct{}
|
|
out := protoimpl.TypeBuilder{
|
|
File: protoimpl.DescBuilder{
|
|
GoPackagePath: reflect.TypeOf(x{}).PkgPath(),
|
|
RawDescriptor: file_remote_ethbackend_proto_rawDesc,
|
|
NumEnums: 2,
|
|
NumMessages: 27,
|
|
NumExtensions: 0,
|
|
NumServices: 1,
|
|
},
|
|
GoTypes: file_remote_ethbackend_proto_goTypes,
|
|
DependencyIndexes: file_remote_ethbackend_proto_depIdxs,
|
|
EnumInfos: file_remote_ethbackend_proto_enumTypes,
|
|
MessageInfos: file_remote_ethbackend_proto_msgTypes,
|
|
}.Build()
|
|
File_remote_ethbackend_proto = out.File
|
|
file_remote_ethbackend_proto_rawDesc = nil
|
|
file_remote_ethbackend_proto_goTypes = nil
|
|
file_remote_ethbackend_proto_depIdxs = nil
|
|
}
|