mirror of
https://gitlab.com/pulsechaincom/erigon-pulse.git
synced 2024-12-25 21:17:16 +00:00
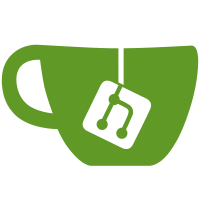
* plain state indexes * generalize index generation&tests * add plain state tests * fix regenerate index * сoncurrent changeset chunks processing * remove concurrency * fix lint * remove comments * add test to truncate * fix conflicts * fmt * remove shadowing
41 lines
655 B
Go
41 lines
655 B
Go
package etl
|
|
|
|
import "bytes"
|
|
|
|
type HeapElem struct {
|
|
Key []byte
|
|
TimeIdx int
|
|
Value []byte
|
|
}
|
|
|
|
type Heap []HeapElem
|
|
|
|
func (h Heap) Len() int {
|
|
return len(h)
|
|
}
|
|
|
|
func (h Heap) Less(i, j int) bool {
|
|
if c := bytes.Compare(h[i].Key, h[j].Key); c != 0 {
|
|
return c < 0
|
|
}
|
|
return h[i].TimeIdx < h[j].TimeIdx
|
|
}
|
|
|
|
func (h Heap) Swap(i, j int) {
|
|
h[i], h[j] = h[j], h[i]
|
|
}
|
|
|
|
func (h *Heap) Push(x interface{}) {
|
|
// Push and Pop use pointer receivers because they modify the slice's length,
|
|
// not just its contents.
|
|
*h = append(*h, x.(HeapElem))
|
|
}
|
|
|
|
func (h *Heap) Pop() interface{} {
|
|
old := *h
|
|
n := len(old)
|
|
x := old[n-1]
|
|
*h = old[0 : n-1]
|
|
return x
|
|
}
|