mirror of
https://gitlab.com/pulsechaincom/lighthouse-pulse.git
synced 2024-12-24 20:47:17 +00:00
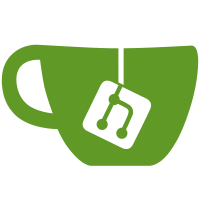
## Proposed Changes This is a refactor of the PeerDB and PeerManager. A number of bugs have been surfacing around the connection state of peers and their interaction with the score state. This refactor tightens the mutability properties of peers such that only specific modules are able to modify the state of peer information preventing inadvertant state changes that can lead to our local peer manager db being out of sync with libp2p. Further, the logic around connection and scoring was quite convoluted and the distinction between the PeerManager and Peerdb was not well defined. Although these issues are not fully resolved, this PR is step to cleaning up this logic. The peerdb solely manages most mutability operations of peers leaving high-order logic to the peer manager. A single `update_connection_state()` function has been added to the peer-db making it solely responsible for modifying the peer's connection state. The way the peer's scores can be modified have been reduced to three simple functions (`update_scores()`, `update_gossipsub_scores()` and `report_peer()`). This prevents any add-hoc modifications of scores and only natural processes of score modification is allowed which simplifies the reasoning of score and state changes.
158 lines
5.0 KiB
Rust
158 lines
5.0 KiB
Rust
use beacon_chain::{
|
|
test_utils::{BeaconChainHarness, EphemeralHarnessType},
|
|
BeaconChain, BeaconChainTypes,
|
|
};
|
|
use eth2::{BeaconNodeHttpClient, Timeouts};
|
|
use eth2_libp2p::{
|
|
discv5::enr::{CombinedKey, EnrBuilder},
|
|
rpc::methods::{MetaData, MetaDataV2},
|
|
types::{EnrAttestationBitfield, EnrSyncCommitteeBitfield, SyncState},
|
|
ConnectedPoint, Enr, NetworkConfig, NetworkGlobals, PeerId, PeerManager,
|
|
};
|
|
use http_api::{Config, Context};
|
|
use network::NetworkMessage;
|
|
use sensitive_url::SensitiveUrl;
|
|
use slog::Logger;
|
|
use std::future::Future;
|
|
use std::net::{Ipv4Addr, SocketAddr};
|
|
use std::sync::Arc;
|
|
use std::time::Duration;
|
|
use tokio::sync::{mpsc, oneshot};
|
|
use types::{test_utils::generate_deterministic_keypairs, ChainSpec, EthSpec};
|
|
|
|
pub const TCP_PORT: u16 = 42;
|
|
pub const UDP_PORT: u16 = 42;
|
|
pub const SEQ_NUMBER: u64 = 0;
|
|
pub const EXTERNAL_ADDR: &str = "/ip4/0.0.0.0/tcp/9000";
|
|
|
|
/// HTTP API tester that allows interaction with the underlying beacon chain harness.
|
|
pub struct InteractiveTester<E: EthSpec> {
|
|
pub harness: BeaconChainHarness<EphemeralHarnessType<E>>,
|
|
pub client: BeaconNodeHttpClient,
|
|
pub network_rx: mpsc::UnboundedReceiver<NetworkMessage<E>>,
|
|
_server_shutdown: oneshot::Sender<()>,
|
|
}
|
|
|
|
/// The result of calling `create_api_server`.
|
|
///
|
|
/// Glue-type between `tests::ApiTester` and `InteractiveTester`.
|
|
pub struct ApiServer<E: EthSpec, SFut: Future<Output = ()>> {
|
|
pub server: SFut,
|
|
pub listening_socket: SocketAddr,
|
|
pub shutdown_tx: oneshot::Sender<()>,
|
|
pub network_rx: tokio::sync::mpsc::UnboundedReceiver<NetworkMessage<E>>,
|
|
pub local_enr: Enr,
|
|
pub external_peer_id: PeerId,
|
|
}
|
|
|
|
impl<E: EthSpec> InteractiveTester<E> {
|
|
pub async fn new(spec: Option<ChainSpec>, validator_count: usize) -> Self {
|
|
let harness = BeaconChainHarness::new(
|
|
E::default(),
|
|
spec,
|
|
generate_deterministic_keypairs(validator_count),
|
|
);
|
|
|
|
let ApiServer {
|
|
server,
|
|
listening_socket,
|
|
shutdown_tx: _server_shutdown,
|
|
network_rx,
|
|
..
|
|
} = create_api_server(harness.chain.clone(), harness.logger().clone()).await;
|
|
|
|
tokio::spawn(server);
|
|
|
|
let client = BeaconNodeHttpClient::new(
|
|
SensitiveUrl::parse(&format!(
|
|
"http://{}:{}",
|
|
listening_socket.ip(),
|
|
listening_socket.port()
|
|
))
|
|
.unwrap(),
|
|
Timeouts::set_all(Duration::from_secs(1)),
|
|
);
|
|
|
|
Self {
|
|
harness,
|
|
client,
|
|
network_rx,
|
|
_server_shutdown,
|
|
}
|
|
}
|
|
}
|
|
|
|
pub async fn create_api_server<T: BeaconChainTypes>(
|
|
chain: Arc<BeaconChain<T>>,
|
|
log: Logger,
|
|
) -> ApiServer<T::EthSpec, impl Future<Output = ()>> {
|
|
let (network_tx, network_rx) = mpsc::unbounded_channel();
|
|
|
|
// Default metadata
|
|
let meta_data = MetaData::V2(MetaDataV2 {
|
|
seq_number: SEQ_NUMBER,
|
|
attnets: EnrAttestationBitfield::<T::EthSpec>::default(),
|
|
syncnets: EnrSyncCommitteeBitfield::<T::EthSpec>::default(),
|
|
});
|
|
let enr_key = CombinedKey::generate_secp256k1();
|
|
let enr = EnrBuilder::new("v4").build(&enr_key).unwrap();
|
|
let network_globals = Arc::new(NetworkGlobals::new(
|
|
enr.clone(),
|
|
TCP_PORT,
|
|
UDP_PORT,
|
|
meta_data,
|
|
vec![],
|
|
&log,
|
|
));
|
|
|
|
// Only a peer manager can add peers, so we create a dummy manager.
|
|
let network_config = NetworkConfig::default();
|
|
let mut pm = PeerManager::new(&network_config, network_globals.clone(), &log)
|
|
.await
|
|
.unwrap();
|
|
|
|
// add a peer
|
|
let peer_id = PeerId::random();
|
|
|
|
let connected_point = ConnectedPoint::Listener {
|
|
local_addr: EXTERNAL_ADDR.parse().unwrap(),
|
|
send_back_addr: EXTERNAL_ADDR.parse().unwrap(),
|
|
};
|
|
let num_established = std::num::NonZeroU32::new(1).unwrap();
|
|
pm.inject_connection_established(peer_id, connected_point, num_established, None);
|
|
*network_globals.sync_state.write() = SyncState::Synced;
|
|
|
|
let eth1_service = eth1::Service::new(eth1::Config::default(), log.clone(), chain.spec.clone());
|
|
|
|
let context = Arc::new(Context {
|
|
config: Config {
|
|
enabled: true,
|
|
listen_addr: Ipv4Addr::new(127, 0, 0, 1),
|
|
listen_port: 0,
|
|
allow_origin: None,
|
|
serve_legacy_spec: true,
|
|
},
|
|
chain: Some(chain.clone()),
|
|
network_tx: Some(network_tx),
|
|
network_globals: Some(network_globals),
|
|
eth1_service: Some(eth1_service),
|
|
log,
|
|
});
|
|
let ctx = context.clone();
|
|
let (shutdown_tx, shutdown_rx) = oneshot::channel();
|
|
let server_shutdown = async {
|
|
// It's not really interesting why this triggered, just that it happened.
|
|
let _ = shutdown_rx.await;
|
|
};
|
|
let (listening_socket, server) = http_api::serve(ctx, server_shutdown).unwrap();
|
|
|
|
ApiServer {
|
|
server,
|
|
listening_socket,
|
|
shutdown_tx,
|
|
network_rx,
|
|
local_enr: enr,
|
|
external_peer_id: peer_id,
|
|
}
|
|
}
|