mirror of
https://gitlab.com/pulsechaincom/lighthouse-pulse.git
synced 2025-01-09 20:41:22 +00:00
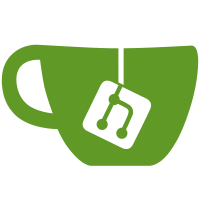
## Issue Addressed Running a beacon node I triggered a sync debug panic. And so finally the time to create tests for sync arrived. Fortunately, te bug was not in the sync algorithm itself but a wrong assertion ## Proposed Changes - Split Range's impl from the BeaconChain via a trait. This is needed for testing. The TestingRig/Harness is way bigger than needed and does not provide the modification functionalities that are needed to test sync. I find this simpler, tho some could disagree. - Add a regression test for sync that fails before the changes. - Fix the wrong assertion.
34 lines
1.2 KiB
Rust
34 lines
1.2 KiB
Rust
use std::sync::Arc;
|
|
|
|
use beacon_chain::{BeaconChain, BeaconChainError, BeaconChainTypes};
|
|
|
|
use lighthouse_network::rpc::StatusMessage;
|
|
/// Trait to produce a `StatusMessage` representing the state of the given `beacon_chain`.
|
|
///
|
|
/// NOTE: The purpose of this is simply to obtain a `StatusMessage` from the `BeaconChain` without
|
|
/// polluting/coupling the type with RPC concepts.
|
|
pub trait ToStatusMessage {
|
|
fn status_message(&self) -> Result<StatusMessage, BeaconChainError>;
|
|
}
|
|
|
|
impl<T: BeaconChainTypes> ToStatusMessage for BeaconChain<T> {
|
|
fn status_message(&self) -> Result<StatusMessage, BeaconChainError> {
|
|
let head_info = self.head_info()?;
|
|
let fork_digest = self.enr_fork_id().fork_digest;
|
|
|
|
Ok(StatusMessage {
|
|
fork_digest,
|
|
finalized_root: head_info.finalized_checkpoint.root,
|
|
finalized_epoch: head_info.finalized_checkpoint.epoch,
|
|
head_root: head_info.block_root,
|
|
head_slot: head_info.slot,
|
|
})
|
|
}
|
|
}
|
|
|
|
impl<T: BeaconChainTypes> ToStatusMessage for Arc<BeaconChain<T>> {
|
|
fn status_message(&self) -> Result<StatusMessage, BeaconChainError> {
|
|
<BeaconChain<T> as ToStatusMessage>::status_message(self)
|
|
}
|
|
}
|