mirror of
https://gitlab.com/pulsechaincom/lighthouse-pulse.git
synced 2025-01-18 17:54:14 +00:00
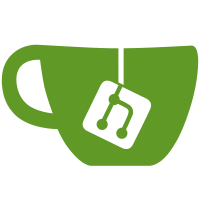
## Issue Addressed Fixes an issue identified by @remyroy whereby we were logging a recommendation to use `--eth1-endpoints` on merge-ready setups (when the execution layer was out of sync). ## Proposed Changes I took the opportunity to clean up the other eth1-related logs, replacing "eth1" by "deposit contract" or "execution" as appropriate. I've downgraded the severity of the `CRIT` log to `ERRO` and removed most of the recommendation text. The reason being that users lacking an execution endpoint will be informed by the new `WARN Not merge ready` log pre-Bellatrix, or the regular errors from block verification post-Bellatrix.
64 lines
1.7 KiB
Rust
64 lines
1.7 KiB
Rust
use itertools::{join, zip};
|
|
use std::fmt::{Debug, Display};
|
|
use std::future::Future;
|
|
|
|
#[derive(Clone)]
|
|
pub struct Fallback<T> {
|
|
pub servers: Vec<T>,
|
|
}
|
|
|
|
#[derive(Debug, PartialEq)]
|
|
pub enum FallbackError<E> {
|
|
AllErrored(Vec<E>),
|
|
}
|
|
|
|
impl<T> Fallback<T> {
|
|
pub fn new(servers: Vec<T>) -> Self {
|
|
Self { servers }
|
|
}
|
|
|
|
/// Return the first successful result along with number of previous errors encountered
|
|
/// or all the errors encountered if every server fails.
|
|
pub async fn first_success<'a, F, O, E, R>(
|
|
&'a self,
|
|
func: F,
|
|
) -> Result<(O, usize), FallbackError<E>>
|
|
where
|
|
F: Fn(&'a T) -> R,
|
|
R: Future<Output = Result<O, E>>,
|
|
{
|
|
let mut errors = vec![];
|
|
for server in &self.servers {
|
|
match func(server).await {
|
|
Ok(val) => return Ok((val, errors.len())),
|
|
Err(e) => errors.push(e),
|
|
}
|
|
}
|
|
Err(FallbackError::AllErrored(errors))
|
|
}
|
|
|
|
pub fn map_format_error<'a, E, F, S>(&'a self, f: F, error: &FallbackError<E>) -> String
|
|
where
|
|
F: FnMut(&'a T) -> &'a S,
|
|
S: Display + 'a,
|
|
E: Debug,
|
|
{
|
|
match error {
|
|
FallbackError::AllErrored(v) => format!(
|
|
"All fallbacks errored: {}",
|
|
join(
|
|
zip(self.servers.iter().map(f), v.iter())
|
|
.map(|(server, error)| format!("{} => {:?}", server, error)),
|
|
", "
|
|
)
|
|
),
|
|
}
|
|
}
|
|
}
|
|
|
|
impl<T: Display> Fallback<T> {
|
|
pub fn format_error<E: Debug>(&self, error: &FallbackError<E>) -> String {
|
|
self.map_format_error(|s| s, error)
|
|
}
|
|
}
|