mirror of
https://github.com/torvalds/linux.git
synced 2025-04-11 04:53:02 +00:00
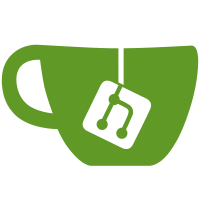
Add the initial nova-core driver stub. nova-core is intended to serve as a common base for nova-drm (the corresponding DRM driver) and the vGPU manager VFIO driver, serving as a hard- and firmware abstraction layer for GSP-based NVIDIA GPUs. The Nova project, including nova-core and nova-drm, in the long term, is intended to serve as the successor of Nouveau for all GSP-based GPUs. The motivation for both, starting a successor project for Nouveau and doing so using the Rust programming language, is documented in detail through a previous post on the mailing list [1], an LWN article [2] and a talk from LPC '24. In order to avoid the chicken and egg problem to require a user to upstream Rust abstractions, but at the same time require the Rust abstractions to implement the driver, nova-core kicks off as a driver stub and is subsequently developed upstream. Link: https://lore.kernel.org/dri-devel/Zfsj0_tb-0-tNrJy@cassiopeiae/T/#u [1] Link: https://lwn.net/Articles/990736/ [2] Link: https://youtu.be/3Igmx28B3BQ?si=sBdSEer4tAPKGpOs [3] Reviewed-by: Alexandre Courbot <acourbot@nvidia.com> Link: https://lore.kernel.org/r/20250306222336.23482-5-dakr@kernel.org Signed-off-by: Danilo Krummrich <dakr@kernel.org>
46 lines
1.3 KiB
Rust
46 lines
1.3 KiB
Rust
// SPDX-License-Identifier: GPL-2.0
|
|
|
|
use crate::gpu;
|
|
use kernel::firmware;
|
|
|
|
pub(crate) struct ModInfoBuilder<const N: usize>(firmware::ModInfoBuilder<N>);
|
|
|
|
impl<const N: usize> ModInfoBuilder<N> {
|
|
const VERSION: &'static str = "535.113.01";
|
|
|
|
const fn make_entry_file(self, chipset: &str, fw: &str) -> Self {
|
|
ModInfoBuilder(
|
|
self.0
|
|
.new_entry()
|
|
.push("nvidia/")
|
|
.push(chipset)
|
|
.push("/gsp/")
|
|
.push(fw)
|
|
.push("-")
|
|
.push(Self::VERSION)
|
|
.push(".bin"),
|
|
)
|
|
}
|
|
|
|
const fn make_entry_chipset(self, chipset: &str) -> Self {
|
|
self.make_entry_file(chipset, "booter_load")
|
|
.make_entry_file(chipset, "booter_unload")
|
|
.make_entry_file(chipset, "bootloader")
|
|
.make_entry_file(chipset, "gsp")
|
|
}
|
|
|
|
pub(crate) const fn create(
|
|
module_name: &'static kernel::str::CStr,
|
|
) -> firmware::ModInfoBuilder<N> {
|
|
let mut this = Self(firmware::ModInfoBuilder::new(module_name));
|
|
let mut i = 0;
|
|
|
|
while i < gpu::Chipset::NAMES.len() {
|
|
this = this.make_entry_chipset(gpu::Chipset::NAMES[i]);
|
|
i += 1;
|
|
}
|
|
|
|
this.0
|
|
}
|
|
}
|