mirror of
https://github.com/torvalds/linux.git
synced 2025-04-06 00:16:18 +00:00
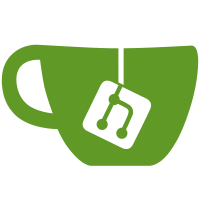
Flit mode introduced in PCIe r6.0 alters how the TLP Header Log is presented through AER and DPC Capability registers. The TLP Prefix Log Register is not present with Flit mode, and the register becomes an extension of the TLP Header Log (PCIe r6.1 secs 7.8.4.12 & 7.9.14.13). Adapt pcie_read_tlp_log() and struct pcie_tlp_log to read and store the extended TLP Header Log when the Link is in Flit mode. As the Prefix Log and Extended TLP Header are not present at the same time, a C union can be used. Determining whether the error occurred while the Link was in Flit mode is a bit complicated. In case of AER, the Advanced Error Capabilities and Control Register directly tells whether the error was logged in Flit mode or not (PCIe r6.1 sec 7.8.4.7). The DPC Capability (PCIe r6.1 sec 7.9.14), unfortunately, does not contain the same information. Unlike AER, the DPC Capability does not provide a way to discern whether the error was logged in Flit mode (this is confirmed by PCI WG to be an oversight in the spec). DPC will bring the Link down immediately following an error, which makes it impossible to acquire the Flit Mode Status directly from the Link Status 2 register because Flit Mode Status is only set in certain Link states (PCIe r6.1 sec 7.5.3.20). As a workaround, use the flit_mode value stored into the struct pci_bus. Link: https://lore.kernel.org/r/20250207161836.2755-3-ilpo.jarvinen@linux.intel.com Signed-off-by: Ilpo Järvinen <ilpo.jarvinen@linux.intel.com> Signed-off-by: Bjorn Helgaas <bhelgaas@google.com>
136 lines
3.4 KiB
C
136 lines
3.4 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* PCIe TLP Log handling
|
|
*
|
|
* Copyright (C) 2024 Intel Corporation
|
|
*/
|
|
|
|
#include <linux/aer.h>
|
|
#include <linux/array_size.h>
|
|
#include <linux/bitfield.h>
|
|
#include <linux/pci.h>
|
|
#include <linux/string.h>
|
|
|
|
#include "../pci.h"
|
|
|
|
/**
|
|
* aer_tlp_log_len - Calculate AER Capability TLP Header/Prefix Log length
|
|
* @dev: PCIe device
|
|
* @aercc: AER Capabilities and Control register value
|
|
*
|
|
* Return: TLP Header/Prefix Log length
|
|
*/
|
|
unsigned int aer_tlp_log_len(struct pci_dev *dev, u32 aercc)
|
|
{
|
|
if (aercc & PCI_ERR_CAP_TLP_LOG_FLIT)
|
|
return FIELD_GET(PCI_ERR_CAP_TLP_LOG_SIZE, aercc);
|
|
|
|
return PCIE_STD_NUM_TLP_HEADERLOG +
|
|
((aercc & PCI_ERR_CAP_PREFIX_LOG_PRESENT) ?
|
|
dev->eetlp_prefix_max : 0);
|
|
}
|
|
|
|
#ifdef CONFIG_PCIE_DPC
|
|
/**
|
|
* dpc_tlp_log_len - Calculate DPC RP PIO TLP Header/Prefix Log length
|
|
* @dev: PCIe device
|
|
*
|
|
* Return: TLP Header/Prefix Log length
|
|
*/
|
|
unsigned int dpc_tlp_log_len(struct pci_dev *dev)
|
|
{
|
|
/* Remove ImpSpec Log register from the count */
|
|
if (dev->dpc_rp_log_size >= PCIE_STD_NUM_TLP_HEADERLOG + 1)
|
|
return dev->dpc_rp_log_size - 1;
|
|
|
|
return dev->dpc_rp_log_size;
|
|
}
|
|
#endif
|
|
|
|
/**
|
|
* pcie_read_tlp_log - read TLP Header Log
|
|
* @dev: PCIe device
|
|
* @where: PCI Config offset of TLP Header Log
|
|
* @where2: PCI Config offset of TLP Prefix Log
|
|
* @tlp_len: TLP Log length (Header Log + TLP Prefix Log in DWORDs)
|
|
* @flit: TLP Logged in Flit mode
|
|
* @log: TLP Log structure to fill
|
|
*
|
|
* Fill @log from TLP Header Log registers, e.g., AER or DPC.
|
|
*
|
|
* Return: 0 on success and filled TLP Log structure, <0 on error.
|
|
*/
|
|
int pcie_read_tlp_log(struct pci_dev *dev, int where, int where2,
|
|
unsigned int tlp_len, bool flit, struct pcie_tlp_log *log)
|
|
{
|
|
unsigned int i;
|
|
int off, ret;
|
|
|
|
if (tlp_len > ARRAY_SIZE(log->dw))
|
|
tlp_len = ARRAY_SIZE(log->dw);
|
|
|
|
memset(log, 0, sizeof(*log));
|
|
|
|
for (i = 0; i < tlp_len; i++) {
|
|
if (i < PCIE_STD_NUM_TLP_HEADERLOG)
|
|
off = where + i * 4;
|
|
else
|
|
off = where2 + (i - PCIE_STD_NUM_TLP_HEADERLOG) * 4;
|
|
|
|
ret = pci_read_config_dword(dev, off, &log->dw[i]);
|
|
if (ret)
|
|
return pcibios_err_to_errno(ret);
|
|
}
|
|
|
|
/*
|
|
* Hard-code non-Flit mode to 4 DWORDs, for now. The exact length
|
|
* can only be known if the TLP is parsed.
|
|
*/
|
|
log->header_len = flit ? tlp_len : 4;
|
|
log->flit = flit;
|
|
|
|
return 0;
|
|
}
|
|
|
|
#define EE_PREFIX_STR " E-E Prefixes:"
|
|
|
|
/**
|
|
* pcie_print_tlp_log - Print TLP Header / Prefix Log contents
|
|
* @dev: PCIe device
|
|
* @log: TLP Log structure
|
|
* @pfx: String prefix
|
|
*
|
|
* Prints TLP Header and Prefix Log information held by @log.
|
|
*/
|
|
void pcie_print_tlp_log(const struct pci_dev *dev,
|
|
const struct pcie_tlp_log *log, const char *pfx)
|
|
{
|
|
/* EE_PREFIX_STR fits the extended DW space needed for the Flit mode */
|
|
char buf[11 * PCIE_STD_MAX_TLP_HEADERLOG + 1];
|
|
unsigned int i;
|
|
int len;
|
|
|
|
len = scnprintf(buf, sizeof(buf), "%#010x %#010x %#010x %#010x",
|
|
log->dw[0], log->dw[1], log->dw[2], log->dw[3]);
|
|
|
|
if (log->flit) {
|
|
for (i = PCIE_STD_NUM_TLP_HEADERLOG; i < log->header_len; i++) {
|
|
len += scnprintf(buf + len, sizeof(buf) - len,
|
|
" %#010x", log->dw[i]);
|
|
}
|
|
} else {
|
|
if (log->prefix[0])
|
|
len += scnprintf(buf + len, sizeof(buf) - len,
|
|
EE_PREFIX_STR);
|
|
for (i = 0; i < ARRAY_SIZE(log->prefix); i++) {
|
|
if (!log->prefix[i])
|
|
break;
|
|
len += scnprintf(buf + len, sizeof(buf) - len,
|
|
" %#010x", log->prefix[i]);
|
|
}
|
|
}
|
|
|
|
pci_err(dev, "%sTLP Header%s: %s\n", pfx,
|
|
log->flit ? " (Flit)" : "", buf);
|
|
}
|