mirror of
https://github.com/torvalds/linux.git
synced 2025-04-12 16:47:42 +00:00
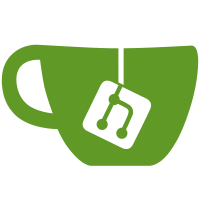
In commit ee2e3f50629f ("mount: fix mounting of detached mounts onto targets that reside on shared mounts") I fixed a bug where propagating the source mount tree of an anonymous mount namespace into a target mount tree of a non-anonymous mount namespace could be used to trigger an integer overflow in the non-anonymous mount namespace causing any new mounts to fail. The cause of this was that the propagation algorithm was unable to recognize mounts from the source mount tree that were already propagated into the target mount tree and then reappeared as propagation targets when walking the destination propagation mount tree. When fixing this I disabled mount propagation into anonymous mount namespaces. Make it possible for anonymous mount namespace to receive mount propagation events correctly. This is no also a correctness issue now that we allow mounting detached mount trees onto detached mount trees. Mark the source anonymous mount namespace with MNTNS_PROPAGATING indicating that all mounts belonging to this mount namespace are currently in the process of being propagated and make the propagation algorithm discard those if they appear as propagation targets. Link: https://lore.kernel.org/r/20250225-work-mount-propagation-v1-1-e6e3724500eb@kernel.org Signed-off-by: Christian Brauner <brauner@kernel.org>
60 lines
2.1 KiB
C
60 lines
2.1 KiB
C
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* linux/fs/pnode.h
|
|
*
|
|
* (C) Copyright IBM Corporation 2005.
|
|
*/
|
|
#ifndef _LINUX_PNODE_H
|
|
#define _LINUX_PNODE_H
|
|
|
|
#include <linux/list.h>
|
|
#include "mount.h"
|
|
|
|
#define IS_MNT_SHARED(m) ((m)->mnt.mnt_flags & MNT_SHARED)
|
|
#define IS_MNT_SLAVE(m) ((m)->mnt_master)
|
|
#define IS_MNT_PROPAGATED(m) (!(m)->mnt_ns || ((m)->mnt_ns->mntns_flags & MNTNS_PROPAGATING))
|
|
#define CLEAR_MNT_SHARED(m) ((m)->mnt.mnt_flags &= ~MNT_SHARED)
|
|
#define IS_MNT_UNBINDABLE(m) ((m)->mnt.mnt_flags & MNT_UNBINDABLE)
|
|
#define IS_MNT_MARKED(m) ((m)->mnt.mnt_flags & MNT_MARKED)
|
|
#define SET_MNT_MARK(m) ((m)->mnt.mnt_flags |= MNT_MARKED)
|
|
#define CLEAR_MNT_MARK(m) ((m)->mnt.mnt_flags &= ~MNT_MARKED)
|
|
#define IS_MNT_LOCKED(m) ((m)->mnt.mnt_flags & MNT_LOCKED)
|
|
|
|
#define CL_EXPIRE 0x01
|
|
#define CL_SLAVE 0x02
|
|
#define CL_COPY_UNBINDABLE 0x04
|
|
#define CL_MAKE_SHARED 0x08
|
|
#define CL_PRIVATE 0x10
|
|
#define CL_SHARED_TO_SLAVE 0x20
|
|
#define CL_COPY_MNT_NS_FILE 0x40
|
|
|
|
#define CL_COPY_ALL (CL_COPY_UNBINDABLE | CL_COPY_MNT_NS_FILE)
|
|
|
|
static inline void set_mnt_shared(struct mount *mnt)
|
|
{
|
|
mnt->mnt.mnt_flags &= ~MNT_SHARED_MASK;
|
|
mnt->mnt.mnt_flags |= MNT_SHARED;
|
|
}
|
|
|
|
void change_mnt_propagation(struct mount *, int);
|
|
int propagate_mnt(struct mount *, struct mountpoint *, struct mount *,
|
|
struct hlist_head *);
|
|
int propagate_umount(struct list_head *);
|
|
int propagate_mount_busy(struct mount *, int);
|
|
void propagate_mount_unlock(struct mount *);
|
|
void mnt_release_group_id(struct mount *);
|
|
int get_dominating_id(struct mount *mnt, const struct path *root);
|
|
int mnt_get_count(struct mount *mnt);
|
|
void mnt_set_mountpoint(struct mount *, struct mountpoint *,
|
|
struct mount *);
|
|
void mnt_change_mountpoint(struct mount *parent, struct mountpoint *mp,
|
|
struct mount *mnt);
|
|
struct mount *copy_tree(struct mount *, struct dentry *, int);
|
|
bool is_path_reachable(struct mount *, struct dentry *,
|
|
const struct path *root);
|
|
int count_mounts(struct mnt_namespace *ns, struct mount *mnt);
|
|
bool propagation_would_overmount(const struct mount *from,
|
|
const struct mount *to,
|
|
const struct mountpoint *mp);
|
|
#endif /* _LINUX_PNODE_H */
|