mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
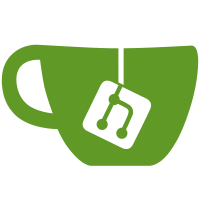
* remove api/gateway/apimiddleware * fix errors in api/gateway * remove beacon-chain/rpc/apimiddleware * fix errors in api/client/beacon * fix errors in validator/client/beacon-api * fix errors in beacon-chain/node * fix errors in validator/node * fix errors in cmd/prysmctl/validator * fix errors in testing/endtoend * fix all other code * remove comment * fix tests --------- Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
80 lines
1.7 KiB
Go
80 lines
1.7 KiB
Go
package gateway
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/gorilla/mux"
|
|
gwruntime "github.com/grpc-ecosystem/grpc-gateway/v2/runtime"
|
|
)
|
|
|
|
type Option func(g *Gateway) error
|
|
|
|
func WithPbHandlers(handlers []*PbMux) Option {
|
|
return func(g *Gateway) error {
|
|
g.cfg.pbHandlers = handlers
|
|
return nil
|
|
}
|
|
}
|
|
|
|
func WithMuxHandler(m MuxHandler) Option {
|
|
return func(g *Gateway) error {
|
|
g.cfg.muxHandler = m
|
|
return nil
|
|
}
|
|
}
|
|
|
|
func WithGatewayAddr(addr string) Option {
|
|
return func(g *Gateway) error {
|
|
g.cfg.gatewayAddr = addr
|
|
return nil
|
|
}
|
|
}
|
|
|
|
func WithRemoteAddr(addr string) Option {
|
|
return func(g *Gateway) error {
|
|
g.cfg.remoteAddr = addr
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithRouter allows adding a custom mux router to the gateway.
|
|
func WithRouter(r *mux.Router) Option {
|
|
return func(g *Gateway) error {
|
|
g.cfg.router = r
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithAllowedOrigins allows adding a set of allowed origins to the gateway.
|
|
func WithAllowedOrigins(origins []string) Option {
|
|
return func(g *Gateway) error {
|
|
g.cfg.allowedOrigins = origins
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithRemoteCert allows adding a custom certificate to the gateway,
|
|
func WithRemoteCert(cert string) Option {
|
|
return func(g *Gateway) error {
|
|
g.cfg.remoteCert = cert
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithMaxCallRecvMsgSize allows specifying the maximum allowed gRPC message size.
|
|
func WithMaxCallRecvMsgSize(size uint64) Option {
|
|
return func(g *Gateway) error {
|
|
g.cfg.maxCallRecvMsgSize = size
|
|
return nil
|
|
}
|
|
}
|
|
|
|
// WithTimeout allows changing the timeout value for API calls.
|
|
func WithTimeout(seconds uint64) Option {
|
|
return func(g *Gateway) error {
|
|
g.cfg.timeout = time.Second * time.Duration(seconds)
|
|
gwruntime.DefaultContextTimeout = time.Second * time.Duration(seconds)
|
|
return nil
|
|
}
|
|
}
|