mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
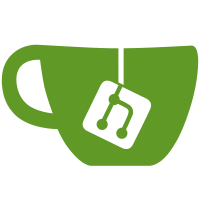
* First take at updating everything to v5 * Patch gRPC gateway to use prysm v5 Fix patch * Update go ssz --------- Co-authored-by: Preston Van Loon <pvanloon@offchainlabs.com>
71 lines
2.7 KiB
Go
71 lines
2.7 KiB
Go
package builder
|
|
|
|
import (
|
|
"context"
|
|
"testing"
|
|
"time"
|
|
|
|
buildertesting "github.com/prysmaticlabs/prysm/v5/api/client/builder/testing"
|
|
blockchainTesting "github.com/prysmaticlabs/prysm/v5/beacon-chain/blockchain/testing"
|
|
dbtesting "github.com/prysmaticlabs/prysm/v5/beacon-chain/db/testing"
|
|
"github.com/prysmaticlabs/prysm/v5/encoding/bytesutil"
|
|
eth "github.com/prysmaticlabs/prysm/v5/proto/prysm/v1alpha1"
|
|
"github.com/prysmaticlabs/prysm/v5/testing/assert"
|
|
"github.com/prysmaticlabs/prysm/v5/testing/require"
|
|
)
|
|
|
|
func Test_NewServiceWithBuilder(t *testing.T) {
|
|
s, err := NewService(context.Background(), WithBuilderClient(&buildertesting.MockClient{}))
|
|
require.NoError(t, err)
|
|
assert.Equal(t, true, s.Configured())
|
|
}
|
|
|
|
func Test_NewServiceWithoutBuilder(t *testing.T) {
|
|
s, err := NewService(context.Background())
|
|
require.NoError(t, err)
|
|
assert.Equal(t, false, s.Configured())
|
|
}
|
|
|
|
func Test_RegisterValidator(t *testing.T) {
|
|
ctx := context.Background()
|
|
db := dbtesting.SetupDB(t)
|
|
headFetcher := &blockchainTesting.ChainService{}
|
|
builder := buildertesting.NewClient()
|
|
s, err := NewService(ctx, WithDatabase(db), WithHeadFetcher(headFetcher), WithBuilderClient(&builder))
|
|
require.NoError(t, err)
|
|
pubkey := bytesutil.ToBytes48([]byte("pubkey"))
|
|
var feeRecipient [20]byte
|
|
require.NoError(t, s.RegisterValidator(ctx, []*eth.SignedValidatorRegistrationV1{{Message: ð.ValidatorRegistrationV1{Pubkey: pubkey[:], FeeRecipient: feeRecipient[:]}}}))
|
|
assert.Equal(t, true, builder.RegisteredVals[pubkey])
|
|
}
|
|
|
|
func Test_RegisterValidator_WithCache(t *testing.T) {
|
|
ctx := context.Background()
|
|
headFetcher := &blockchainTesting.ChainService{}
|
|
builder := buildertesting.NewClient()
|
|
s, err := NewService(ctx, WithRegistrationCache(), WithHeadFetcher(headFetcher), WithBuilderClient(&builder))
|
|
require.NoError(t, err)
|
|
pubkey := bytesutil.ToBytes48([]byte("pubkey"))
|
|
var feeRecipient [20]byte
|
|
reg := ð.ValidatorRegistrationV1{Pubkey: pubkey[:], Timestamp: uint64(time.Now().UTC().Unix()), FeeRecipient: feeRecipient[:]}
|
|
require.NoError(t, s.RegisterValidator(ctx, []*eth.SignedValidatorRegistrationV1{{Message: reg}}))
|
|
registration, err := s.registrationCache.RegistrationByIndex(0)
|
|
require.NoError(t, err)
|
|
require.DeepEqual(t, reg, registration)
|
|
}
|
|
|
|
func Test_BuilderMethodsWithouClient(t *testing.T) {
|
|
s, err := NewService(context.Background())
|
|
require.NoError(t, err)
|
|
assert.Equal(t, false, s.Configured())
|
|
|
|
_, err = s.GetHeader(context.Background(), 0, [32]byte{}, [48]byte{})
|
|
assert.ErrorContains(t, ErrNoBuilder.Error(), err)
|
|
|
|
_, _, err = s.SubmitBlindedBlock(context.Background(), nil)
|
|
assert.ErrorContains(t, ErrNoBuilder.Error(), err)
|
|
|
|
err = s.RegisterValidator(context.Background(), nil)
|
|
assert.ErrorContains(t, ErrNoBuilder.Error(), err)
|
|
}
|