mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
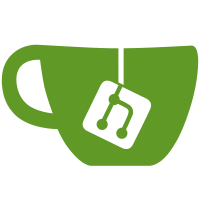
* First take at updating everything to v5 * Patch gRPC gateway to use prysm v5 Fix patch * Update go ssz --------- Co-authored-by: Preston Van Loon <pvanloon@offchainlabs.com>
74 lines
2.8 KiB
Go
74 lines
2.8 KiB
Go
package p2p
|
|
|
|
import (
|
|
"reflect"
|
|
|
|
"github.com/prysmaticlabs/prysm/v5/config/params"
|
|
"github.com/prysmaticlabs/prysm/v5/consensus-types/primitives"
|
|
ethpb "github.com/prysmaticlabs/prysm/v5/proto/prysm/v1alpha1"
|
|
"google.golang.org/protobuf/proto"
|
|
)
|
|
|
|
// gossipTopicMappings represent the protocol ID to protobuf message type map for easy
|
|
// lookup.
|
|
var gossipTopicMappings = map[string]proto.Message{
|
|
BlockSubnetTopicFormat: ðpb.SignedBeaconBlock{},
|
|
AttestationSubnetTopicFormat: ðpb.Attestation{},
|
|
ExitSubnetTopicFormat: ðpb.SignedVoluntaryExit{},
|
|
ProposerSlashingSubnetTopicFormat: ðpb.ProposerSlashing{},
|
|
AttesterSlashingSubnetTopicFormat: ðpb.AttesterSlashing{},
|
|
AggregateAndProofSubnetTopicFormat: ðpb.SignedAggregateAttestationAndProof{},
|
|
SyncContributionAndProofSubnetTopicFormat: ðpb.SignedContributionAndProof{},
|
|
SyncCommitteeSubnetTopicFormat: ðpb.SyncCommitteeMessage{},
|
|
BlsToExecutionChangeSubnetTopicFormat: ðpb.SignedBLSToExecutionChange{},
|
|
BlobSubnetTopicFormat: ðpb.BlobSidecar{},
|
|
}
|
|
|
|
// GossipTopicMappings is a function to return the assigned data type
|
|
// versioned by epoch.
|
|
func GossipTopicMappings(topic string, epoch primitives.Epoch) proto.Message {
|
|
if topic == BlockSubnetTopicFormat {
|
|
if epoch >= params.BeaconConfig().DenebForkEpoch {
|
|
return ðpb.SignedBeaconBlockDeneb{}
|
|
}
|
|
if epoch >= params.BeaconConfig().CapellaForkEpoch {
|
|
return ðpb.SignedBeaconBlockCapella{}
|
|
}
|
|
if epoch >= params.BeaconConfig().BellatrixForkEpoch {
|
|
return ðpb.SignedBeaconBlockBellatrix{}
|
|
}
|
|
if epoch >= params.BeaconConfig().AltairForkEpoch {
|
|
return ðpb.SignedBeaconBlockAltair{}
|
|
}
|
|
}
|
|
return gossipTopicMappings[topic]
|
|
}
|
|
|
|
// AllTopics returns all topics stored in our
|
|
// gossip mapping.
|
|
func AllTopics() []string {
|
|
var topics []string
|
|
for k := range gossipTopicMappings {
|
|
topics = append(topics, k)
|
|
}
|
|
return topics
|
|
}
|
|
|
|
// GossipTypeMapping is the inverse of GossipTopicMappings so that an arbitrary protobuf message
|
|
// can be mapped to a protocol ID string.
|
|
var GossipTypeMapping = make(map[reflect.Type]string, len(gossipTopicMappings))
|
|
|
|
func init() {
|
|
for k, v := range gossipTopicMappings {
|
|
GossipTypeMapping[reflect.TypeOf(v)] = k
|
|
}
|
|
// Specially handle Altair objects.
|
|
GossipTypeMapping[reflect.TypeOf(ðpb.SignedBeaconBlockAltair{})] = BlockSubnetTopicFormat
|
|
// Specially handle Bellatrix objects.
|
|
GossipTypeMapping[reflect.TypeOf(ðpb.SignedBeaconBlockBellatrix{})] = BlockSubnetTopicFormat
|
|
// Specially handle Capella objects.
|
|
GossipTypeMapping[reflect.TypeOf(ðpb.SignedBeaconBlockCapella{})] = BlockSubnetTopicFormat
|
|
// Specially handle Deneb objects.
|
|
GossipTypeMapping[reflect.TypeOf(ðpb.SignedBeaconBlockDeneb{})] = BlockSubnetTopicFormat
|
|
}
|