mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
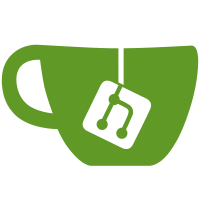
* First take at updating everything to v5 * Patch gRPC gateway to use prysm v5 Fix patch * Update go ssz --------- Co-authored-by: Preston Van Loon <pvanloon@offchainlabs.com>
67 lines
1.9 KiB
Go
67 lines
1.9 KiB
Go
package p2p
|
|
|
|
import (
|
|
"fmt"
|
|
"testing"
|
|
|
|
"github.com/ethereum/go-ethereum/crypto"
|
|
"github.com/ethereum/go-ethereum/p2p/enode"
|
|
"github.com/prysmaticlabs/prysm/v5/config/params"
|
|
"github.com/prysmaticlabs/prysm/v5/testing/assert"
|
|
"github.com/prysmaticlabs/prysm/v5/testing/require"
|
|
logTest "github.com/sirupsen/logrus/hooks/test"
|
|
)
|
|
|
|
// Test `verifyConnectivity` function by trying to connect to google.com (successfully)
|
|
// and then by connecting to an unreachable IP and ensuring that a log is emitted
|
|
func TestVerifyConnectivity(t *testing.T) {
|
|
params.SetupTestConfigCleanup(t)
|
|
hook := logTest.NewGlobal()
|
|
cases := []struct {
|
|
address string
|
|
port uint
|
|
expectedConnectivity bool
|
|
name string
|
|
}{
|
|
{"142.250.68.46", 80, true, "Dialing a reachable IP: 142.250.68.46:80"}, // google.com
|
|
{"123.123.123.123", 19000, false, "Dialing an unreachable IP: 123.123.123.123:19000"},
|
|
}
|
|
for _, tc := range cases {
|
|
t.Run(fmt.Sprintf(tc.name),
|
|
func(t *testing.T) {
|
|
verifyConnectivity(tc.address, tc.port, "tcp")
|
|
logMessage := "IP address is not accessible"
|
|
if tc.expectedConnectivity {
|
|
require.LogsDoNotContain(t, hook, logMessage)
|
|
} else {
|
|
require.LogsContain(t, hook, logMessage)
|
|
}
|
|
})
|
|
}
|
|
}
|
|
|
|
func TestSerializeENR(t *testing.T) {
|
|
params.SetupTestConfigCleanup(t)
|
|
t.Run("Ok", func(t *testing.T) {
|
|
key, err := crypto.GenerateKey()
|
|
require.NoError(t, err)
|
|
db, err := enode.OpenDB("")
|
|
require.NoError(t, err)
|
|
lNode := enode.NewLocalNode(db, key)
|
|
record := lNode.Node().Record()
|
|
s, err := SerializeENR(record)
|
|
require.NoError(t, err)
|
|
assert.NotEqual(t, "", s)
|
|
s = "enr:" + s
|
|
newRec, err := enode.Parse(enode.ValidSchemes, s)
|
|
require.NoError(t, err)
|
|
assert.Equal(t, s, newRec.String())
|
|
})
|
|
|
|
t.Run("Nil record", func(t *testing.T) {
|
|
_, err := SerializeENR(nil)
|
|
require.NotNil(t, err)
|
|
assert.ErrorContains(t, "could not serialize nil record", err)
|
|
})
|
|
}
|