mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
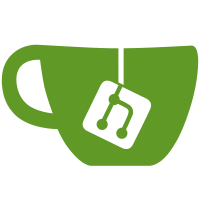
* First take at updating everything to v5 * Patch gRPC gateway to use prysm v5 Fix patch * Update go ssz --------- Co-authored-by: Preston Van Loon <pvanloon@offchainlabs.com>
50 lines
1.1 KiB
Go
50 lines
1.1 KiB
Go
package startup
|
|
|
|
import (
|
|
"testing"
|
|
"time"
|
|
|
|
"github.com/prysmaticlabs/prysm/v5/config/params"
|
|
"github.com/prysmaticlabs/prysm/v5/consensus-types/primitives"
|
|
"github.com/prysmaticlabs/prysm/v5/testing/require"
|
|
)
|
|
|
|
func TestClock(t *testing.T) {
|
|
vr := [32]byte{}
|
|
cases := []struct {
|
|
name string
|
|
nSlots primitives.Slot
|
|
}{
|
|
{
|
|
name: "3 slots",
|
|
nSlots: 3,
|
|
},
|
|
{
|
|
name: "0 slots",
|
|
nSlots: 0,
|
|
},
|
|
{
|
|
name: "1 epoch",
|
|
nSlots: params.BeaconConfig().SlotsPerEpoch,
|
|
},
|
|
}
|
|
for _, c := range cases {
|
|
t.Run(c.name, func(t *testing.T) {
|
|
genesis, now := testInterval(c.nSlots)
|
|
nower := func() time.Time { return now }
|
|
cl := NewClock(genesis, vr, WithNower(nower))
|
|
require.Equal(t, genesis, cl.GenesisTime())
|
|
require.Equal(t, now, cl.Now())
|
|
require.Equal(t, c.nSlots, cl.CurrentSlot())
|
|
})
|
|
}
|
|
}
|
|
|
|
func testInterval(nSlots primitives.Slot) (time.Time, time.Time) {
|
|
oneSlot := time.Second * time.Duration(params.BeaconConfig().SecondsPerSlot)
|
|
var start uint64 = 23
|
|
endOffset := oneSlot * time.Duration(nSlots)
|
|
startTime := time.Unix(int64(start), 0)
|
|
return startTime, startTime.Add(endOffset)
|
|
}
|