mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
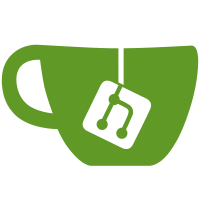
* First take at updating everything to v5 * Patch gRPC gateway to use prysm v5 Fix patch * Update go ssz --------- Co-authored-by: Preston Van Loon <pvanloon@offchainlabs.com>
33 lines
638 B
Go
33 lines
638 B
Go
package stategen
|
|
|
|
import (
|
|
"github.com/pkg/errors"
|
|
"github.com/prysmaticlabs/prysm/v5/beacon-chain/state"
|
|
)
|
|
|
|
var ErrNotInCache = errors.New("state not found in cache")
|
|
|
|
type CachedGetter interface {
|
|
ByBlockRoot([32]byte) (state.BeaconState, error)
|
|
}
|
|
|
|
type CombinedCache struct {
|
|
getters []CachedGetter
|
|
}
|
|
|
|
func (c CombinedCache) ByBlockRoot(r [32]byte) (state.BeaconState, error) {
|
|
for _, getter := range c.getters {
|
|
st, err := getter.ByBlockRoot(r)
|
|
if err == nil {
|
|
return st, nil
|
|
}
|
|
if errors.Is(err, ErrNotInCache) {
|
|
continue
|
|
}
|
|
return nil, err
|
|
}
|
|
return nil, ErrNotInCache
|
|
}
|
|
|
|
var _ CachedGetter = &CombinedCache{}
|