mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
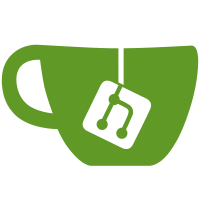
* Use go:build lines and remove obsolete +build lines * Run gazelle * Update crypto/bls/blst/stub.go * Update crypto/bls/blst/stub.go Co-authored-by: Raul Jordan <raul@prysmaticlabs.com> Co-authored-by: Preston Van Loon <preston@prysmaticlabs.com> Co-authored-by: Nishant Das <nishdas93@gmail.com>
40 lines
961 B
Go
40 lines
961 B
Go
//go:build develop
|
|
|
|
package params
|
|
|
|
import (
|
|
"sync"
|
|
|
|
"github.com/mohae/deepcopy"
|
|
)
|
|
|
|
var cfgrw sync.RWMutex
|
|
|
|
// BeaconConfig retrieves beacon chain config.
|
|
func BeaconConfig() *BeaconChainConfig {
|
|
cfgrw.RLock()
|
|
defer cfgrw.RUnlock()
|
|
return configs.getActive()
|
|
}
|
|
|
|
// OverrideBeaconConfig by replacing the config. The preferred pattern is to
|
|
// call BeaconConfig(), change the specific parameters, and then call
|
|
// OverrideBeaconConfig(c). Any subsequent calls to params.BeaconConfig() will
|
|
// return this new configuration.
|
|
func OverrideBeaconConfig(c *BeaconChainConfig) {
|
|
cfgrw.Lock()
|
|
defer cfgrw.Unlock()
|
|
configs.active = c
|
|
}
|
|
|
|
// Copy returns a copy of the config object.
|
|
func (b *BeaconChainConfig) Copy() *BeaconChainConfig {
|
|
cfgrw.RLock()
|
|
defer cfgrw.RUnlock()
|
|
config, ok := deepcopy.Copy(*b).(BeaconChainConfig)
|
|
if !ok {
|
|
panic("somehow deepcopy produced a BeaconChainConfig that is not of the same type as the original")
|
|
}
|
|
return &config
|
|
}
|