mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-21 19:20:38 +00:00
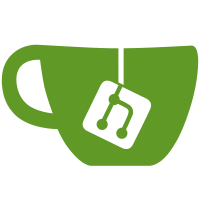
* First take at updating everything to v5 * Patch gRPC gateway to use prysm v5 Fix patch * Update go ssz --------- Co-authored-by: Preston Van Loon <pvanloon@offchainlabs.com>
35 lines
1.1 KiB
Go
35 lines
1.1 KiB
Go
package consensus_types
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"sync/atomic"
|
|
|
|
errors2 "github.com/pkg/errors"
|
|
"github.com/prysmaticlabs/prysm/v5/runtime/version"
|
|
)
|
|
|
|
var (
|
|
// ErrNilObjectWrapped is returned in a constructor when the underlying object is nil.
|
|
ErrNilObjectWrapped = errors.New("attempted to wrap nil object")
|
|
// ErrUnsupportedField is returned when a getter/setter access is not supported.
|
|
ErrUnsupportedField = errors.New("unsupported getter")
|
|
// ErrOutOfBounds is returned when a slice or array index does not exist.
|
|
ErrOutOfBounds = errors.New("index out of bounds")
|
|
)
|
|
|
|
// ErrNotSupported constructs a message informing about an unsupported field access.
|
|
func ErrNotSupported(funcName string, ver int) error {
|
|
return errors2.Wrap(ErrUnsupportedField, fmt.Sprintf("%s is not supported for %s", funcName, version.String(ver)))
|
|
}
|
|
|
|
// ThreadSafeEnumerator is a thread-safe counter of all objects created since the node's start.
|
|
type ThreadSafeEnumerator struct {
|
|
counter uint64
|
|
}
|
|
|
|
// Inc increments the enumerator and returns the new object count.
|
|
func (c *ThreadSafeEnumerator) Inc() uint64 {
|
|
return atomic.AddUint64(&c.counter, 1)
|
|
}
|