mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
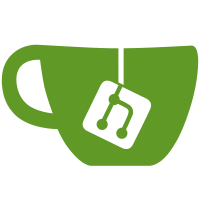
* Update to go1.20 * Update gohashtree * fix build tags for bytesutil and add nilness exclusion * more broad nilness exclusion * Reset nogo config * Update golang.org/x/tools * update rules_go to 0.39.0 --------- Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com>
62 lines
1.6 KiB
Go
62 lines
1.6 KiB
Go
//go:build !go1.20
|
|
// +build !go1.20
|
|
|
|
package bytesutil
|
|
|
|
// These methods use copy() to convert a byte slice to a fixed size array.
|
|
// This approach is used for go1.19 and below.
|
|
|
|
// ToBytes4 is a convenience method for converting a byte slice to a fix
|
|
// sized 4 byte array. This method will truncate the input if it is larger
|
|
// than 4 bytes.
|
|
func ToBytes4(x []byte) [4]byte {
|
|
var y [4]byte
|
|
copy(y[:], x)
|
|
return y
|
|
}
|
|
|
|
// ToBytes20 is a convenience method for converting a byte slice to a fix
|
|
// sized 20 byte array. This method will truncate the input if it is larger
|
|
// than 20 bytes.
|
|
func ToBytes20(x []byte) [20]byte {
|
|
var y [20]byte
|
|
copy(y[:], x)
|
|
return y
|
|
}
|
|
|
|
// ToBytes32 is a convenience method for converting a byte slice to a fix
|
|
// sized 32 byte array. This method will truncate the input if it is larger
|
|
// than 32 bytes.
|
|
func ToBytes32(x []byte) [32]byte {
|
|
var y [32]byte
|
|
copy(y[:], x)
|
|
return y
|
|
}
|
|
|
|
// ToBytes48 is a convenience method for converting a byte slice to a fix
|
|
// sized 48 byte array. This method will truncate the input if it is larger
|
|
// than 48 bytes.
|
|
func ToBytes48(x []byte) [48]byte {
|
|
var y [48]byte
|
|
copy(y[:], x)
|
|
return y
|
|
}
|
|
|
|
// ToBytes64 is a convenience method for converting a byte slice to a fix
|
|
// sized 64 byte array. This method will truncate the input if it is larger
|
|
// than 64 bytes.
|
|
func ToBytes64(x []byte) [64]byte {
|
|
var y [64]byte
|
|
copy(y[:], x)
|
|
return y
|
|
}
|
|
|
|
// ToBytes96 is a convenience method for converting a byte slice to a fix
|
|
// sized 96 byte array. This method will truncate the input if it is larger
|
|
// than 96 bytes.
|
|
func ToBytes96(x []byte) [96]byte {
|
|
var y [96]byte
|
|
copy(y[:], x)
|
|
return y
|
|
}
|