mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
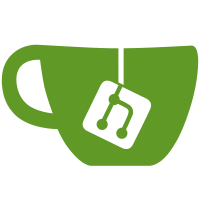
* Remove Feature Flag From Prater (#12082) * Use Epoch boundary cache to retrieve balances (#12083) * Use Epoch boundary cache to retrieve balances * save boundary states before inserting to forkchoice * move up last block save * remove boundary checks on balances * fix ordering --------- Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> * create the log file along with its parent directory if not present * only give ReadWritePermissions to the log file * use io/file package to create the parent directories * fix ci related issues * add regression tests * run gazelle * fix tests * remove print statements * gazelle * Remove failing test for MkdirAll, this failure is not expected --------- Co-authored-by: Nishant Das <nishdas93@gmail.com> Co-authored-by: Potuz <potuz@prysmaticlabs.com> Co-authored-by: prylabs-bulldozer[bot] <58059840+prylabs-bulldozer[bot]@users.noreply.github.com> Co-authored-by: Preston Van Loon <pvanloon@offchainlabs.com>
61 lines
1.8 KiB
Go
61 lines
1.8 KiB
Go
// Package logs creates a Multi writer instance that
|
|
// write all logs that are written to stdout.
|
|
package logs
|
|
|
|
import (
|
|
"io"
|
|
"net/url"
|
|
"os"
|
|
"path/filepath"
|
|
"strings"
|
|
|
|
"github.com/prysmaticlabs/prysm/v5/config/params"
|
|
"github.com/prysmaticlabs/prysm/v5/io/file"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
func addLogWriter(w io.Writer) {
|
|
mw := io.MultiWriter(logrus.StandardLogger().Out, w)
|
|
logrus.SetOutput(mw)
|
|
}
|
|
|
|
// ConfigurePersistentLogging adds a log-to-file writer. File content is identical to stdout.
|
|
func ConfigurePersistentLogging(logFileName string) error {
|
|
logrus.WithField("logFileName", logFileName).Info("Logs will be made persistent")
|
|
if err := file.MkdirAll(filepath.Dir(logFileName)); err != nil {
|
|
return err
|
|
}
|
|
f, err := os.OpenFile(logFileName, os.O_CREATE|os.O_WRONLY|os.O_APPEND, params.BeaconIoConfig().ReadWritePermissions) // #nosec G304
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
addLogWriter(f)
|
|
|
|
logrus.Info("File logging initialized")
|
|
return nil
|
|
}
|
|
|
|
// MaskCredentialsLogging masks the url credentials before logging for security purpose
|
|
// [scheme:][//[userinfo@]host][/]path[?query][#fragment] --> [scheme:][//[***]host][/***][#***]
|
|
// if the format is not matched nothing is done, string is returned as is.
|
|
func MaskCredentialsLogging(currUrl string) string {
|
|
// error if the input is not a URL
|
|
MaskedUrl := currUrl
|
|
u, err := url.Parse(currUrl)
|
|
if err != nil {
|
|
return currUrl // Not a URL, nothing to do
|
|
}
|
|
// Mask the userinfo and the URI (path?query or opaque?query ) and fragment, leave the scheme and host(host/port) untouched
|
|
if u.User != nil {
|
|
MaskedUrl = strings.Replace(MaskedUrl, u.User.String(), "***", 1)
|
|
}
|
|
if len(u.RequestURI()) > 1 { // Ignore the '/'
|
|
MaskedUrl = strings.Replace(MaskedUrl, u.RequestURI(), "/***", 1)
|
|
}
|
|
if len(u.Fragment) > 0 {
|
|
MaskedUrl = strings.Replace(MaskedUrl, u.RawFragment, "***", 1)
|
|
}
|
|
return MaskedUrl
|
|
}
|