mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
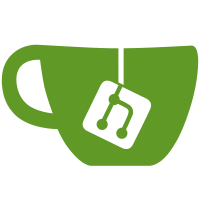
* Value assigned to a variable is never read before being overwritten * The result of append is not used anywhere * Suspicious assignment of range-loop vars detected * Unused method receiver detected * Revert "Auxiliary commit to revert individual files from 54edcb445484a2e5d79612e19af8e949b8861253" This reverts commit bbd1e1beabf7b0c5cfc4f514dcc820062ad6c063. * Method modifies receiver * Fix test * Duplicate imports detected * Incorrectly formatted error string * Types of function parameters can be combined * One more "Unused method receiver detected" * Unused parameter detected in function
52 lines
1.4 KiB
Go
52 lines
1.4 KiB
Go
package prometheus
|
|
|
|
import (
|
|
"errors"
|
|
|
|
"github.com/prometheus/client_golang/prometheus"
|
|
"github.com/prometheus/client_golang/prometheus/promauto"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
// LogrusCollector is a logrus hook to collect log counters.
|
|
type LogrusCollector struct {
|
|
counterVec *prometheus.CounterVec
|
|
}
|
|
|
|
var (
|
|
supportedLevels = []logrus.Level{logrus.InfoLevel, logrus.WarnLevel, logrus.ErrorLevel}
|
|
counterVec = promauto.NewCounterVec(prometheus.CounterOpts{
|
|
Name: "log_entries_total",
|
|
Help: "Total number of log messages.",
|
|
}, []string{"level", "prefix"})
|
|
)
|
|
|
|
const prefixKey = "prefix"
|
|
const defaultprefix = "global"
|
|
|
|
// NewLogrusCollector register internal metrics and return an logrus hook to collect log counters
|
|
// This function can be called only once, if more than one call is made an error will be returned.
|
|
func NewLogrusCollector() *LogrusCollector {
|
|
return &LogrusCollector{
|
|
counterVec: counterVec,
|
|
}
|
|
}
|
|
|
|
// Fire is called on every log call.
|
|
func (hook *LogrusCollector) Fire(entry *logrus.Entry) error {
|
|
prefix := defaultprefix
|
|
if prefixValue, ok := entry.Data[prefixKey]; ok {
|
|
prefix, ok = prefixValue.(string)
|
|
if !ok {
|
|
return errors.New("prefix is not a string")
|
|
}
|
|
}
|
|
hook.counterVec.WithLabelValues(entry.Level.String(), prefix).Inc()
|
|
return nil
|
|
}
|
|
|
|
// Levels return a slice of levels supported by this hook;
|
|
func (_ *LogrusCollector) Levels() []logrus.Level {
|
|
return supportedLevels
|
|
}
|