mirror of
https://gitlab.com/pulsechaincom/prysm-pulse.git
synced 2024-12-22 03:30:35 +00:00
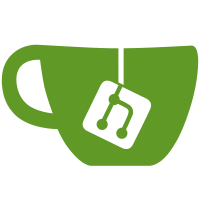
* First take at updating everything to v5 * Patch gRPC gateway to use prysm v5 Fix patch * Update go ssz --------- Co-authored-by: Preston Van Loon <pvanloon@offchainlabs.com>
56 lines
1.4 KiB
Go
56 lines
1.4 KiB
Go
package bls
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/hex"
|
|
"errors"
|
|
"testing"
|
|
|
|
"github.com/ghodss/yaml"
|
|
"github.com/prysmaticlabs/prysm/v5/crypto/bls"
|
|
"github.com/prysmaticlabs/prysm/v5/crypto/bls/common"
|
|
"github.com/prysmaticlabs/prysm/v5/testing/bls/utils"
|
|
"github.com/prysmaticlabs/prysm/v5/testing/require"
|
|
)
|
|
|
|
func TestSign(t *testing.T) {
|
|
t.Run("blst", testSign)
|
|
}
|
|
|
|
func testSign(t *testing.T) {
|
|
fNames, fContent := utils.RetrieveFiles("sign", t)
|
|
|
|
for i, file := range fNames {
|
|
t.Run(file, func(t *testing.T) {
|
|
test := &SignMsgTest{}
|
|
require.NoError(t, yaml.Unmarshal(fContent[i], test))
|
|
pkBytes, err := hex.DecodeString(test.Input.Privkey[2:])
|
|
require.NoError(t, err)
|
|
sk, err := bls.SecretKeyFromBytes(pkBytes)
|
|
if err != nil {
|
|
if test.Output == "" &&
|
|
(errors.Is(err, common.ErrZeroKey) || errors.Is(err, common.ErrSecretUnmarshal)) {
|
|
return
|
|
}
|
|
t.Fatalf("cannot unmarshal secret key: %v", err)
|
|
}
|
|
msgBytes, err := hex.DecodeString(test.Input.Message[2:])
|
|
require.NoError(t, err)
|
|
sig := sk.Sign(msgBytes)
|
|
|
|
if !sig.Verify(sk.PublicKey(), msgBytes) {
|
|
t.Fatal("could not verify signature")
|
|
}
|
|
|
|
outputBytes, err := hex.DecodeString(test.Output[2:])
|
|
require.NoError(t, err)
|
|
|
|
if !bytes.Equal(outputBytes, sig.Marshal()) {
|
|
t.Fatalf("Test Case %d: Signature does not match the expected output. "+
|
|
"Expected %#x but received %#x", i, outputBytes, sig.Marshal())
|
|
}
|
|
t.Log("Success")
|
|
})
|
|
}
|
|
}
|